#include <stdio.h> #define year(n) (n%4==0)? y='L':y='N'; int main(void) { int n=0; char y='0'; year(n) printf("%c",y); return 0; }改正该代码并注释
时间: 2024-04-18 14:24:11 浏览: 71
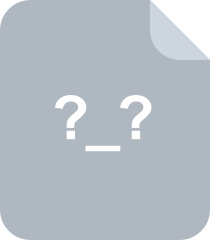
#include <stdio.h>
#include <stdio.h>
// 定义宏,判断年份是否为闰年
#define is_leap_year(n) ((n % 4 == 0 && n % 100 != 0) || n % 400 == 0)
int main(void) {
int year = 0;
char isLeapYear = 'N';
// 输入年份
printf("请输入一个年份:");
scanf("%d", &year);
// 判断是否为闰年
if (is_leap_year(year)) {
isLeapYear = 'L';
}
// 输出结果
printf("该年份是否为闰年:%c\n", isLeapYear);
return 0;
}
// 注释:
// 1. 引入<stdio.h>头文件,用于输入输出操作。
// 2. 定义宏is_leap_year(n)来判断年份n是否为闰年。
// 3. 主函数main中,声明一个整型变量year用于存储输入的年份,声明一个字符型变量isLeapYear用于表示该年份是否为闰年。
// 4. 提示用户输入一个年份并通过scanf函数将输入的值存储到year变量中。
// 5. 利用is_leap_year宏判断year是否为闰年,并将结果存储到isLeapYear变量中。
// 6. 使用printf函数输出结果,提示用户该年份是否为闰年。
// 7. 返回0,表示程序顺利执行结束。
阅读全文
相关推荐
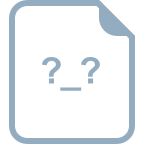















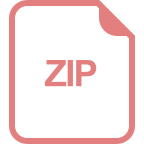
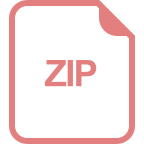
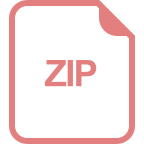