用c语言创建一个有6个结点的单向链表,实现增加、删除、查找、移动、显示结点的基本功能。
时间: 2024-05-30 22:12:39 浏览: 7
好的,可以帮您解决这个问题。以下是实现这个功能的C语言代码:
// 定义链表结点结构体
typedef struct node {
int data; // 结点数据
struct node *next; // 指向下一个结点的指针
} Node;
// 创建链表
Node *createList() {
Node *head = NULL;
Node *p = NULL;
int data;
for (int i = 0; i < 6; i++) {
scanf("%d", &data);
Node *node = (Node*)malloc(sizeof(Node));
node->data = data;
if (head == NULL) {
head = node;
p = head;
} else {
p->next = node;
p = p->next;
}
}
p->next = NULL; // 最后一个结点的指针置为NULL
return head;
}
// 插入结点
Node *insertNode(Node *head, int position, int data) {
Node *p = head;
Node *node = (Node*)malloc(sizeof(Node));
node->data = data;
for (int i = 1; i < position && p != NULL; i++) {
p = p->next;
}
if (p == NULL) {
printf("插入结点失败,位置大于链表长度\n");
free(node);
return head;
} else if (position == 1) {
node->next = head;
return node;
} else {
node->next = p->next;
p->next = node;
return head;
}
}
// 删除结点
Node *deleteNode(Node *head, int position) {
Node *p = head;
if (position == 1) {
head = head->next;
free(p);
return head;
} else {
for (int i = 1; i < position - 1 && p != NULL; i++) {
p = p->next;
}
if (p == NULL || p->next == NULL) {
printf("删除结点失败,位置大于链表长度\n");
return head;
} else {
Node *q = p->next;
p->next = q->next;
free(q);
return head;
}
}
}
// 查找结点
int searchNode(Node *head, int data) {
Node *p = head;
int position = 1;
while (p != NULL && p->data != data) {
p = p->next;
position++;
}
if (p == NULL) {
printf("未找到数据为%d的结点\n", data);
return -1;
} else {
printf("数据为%d的结点在第%d个位置\n", data, position);
return position;
}
}
// 移动结点
Node *moveNode(Node *head, int src, int dst) {
Node *p = head;
int temp;
for (int i = 1; i < src && p != NULL; i++) {
p = p->next;
}
if (p == NULL) {
printf("移动结点失败,源位置大于链表长度\n");
return head;
} else {
Node *q = p->next;
temp = q->data;
deleteNode(head, src);
head = insertNode(head, dst, temp);
return head;
}
}
// 显示链表
void displayList(Node *head) {
Node *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 测试函数
void test() {
Node *head = createList();
displayList(head);
head = insertNode(head, 1, 0);
displayList(head);
head = insertNode(head, 3, 2);
displayList(head);
head = deleteNode(head, 5);
displayList(head);
int position = searchNode(head, 3);
head = moveNode(head, position, 2);
displayList(head);
}
// 主函数
int main() {
test();
return 0;
}
相关推荐
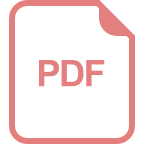
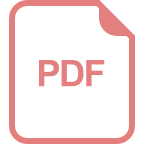
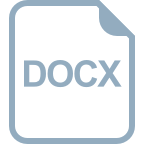
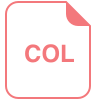
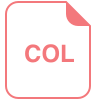
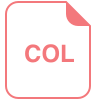
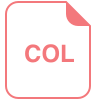
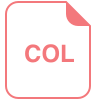









