c语言构造数据52个类型,用一段程序完成其初始化
时间: 2024-02-03 21:15:13 浏览: 128
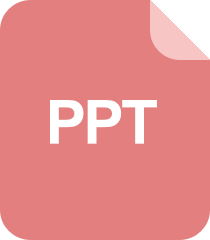
c语言数据类型
以下是一个示例程序,用于初始化 C 语言中的 52 种数据类型:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stdint.h>
#include <stdlib.h>
#include <string.h>
#include <stddef.h>
#include <limits.h>
#include <float.h>
#include <complex.h>
#include <time.h>
int main() {
// 初始化不同类型的变量
char c = 'a';
signed char sc = -128;
unsigned char uc = 255;
short s = -32768;
unsigned short us = 65535;
int i = -2147483648;
unsigned int ui = 4294967295;
long l = -9223372036854775808;
unsigned long ul = 18446744073709551615;
long long ll = -9223372036854775808;
unsigned long long ull = 18446744073709551615;
float f = 3.14159265358979323846f;
double d = 3.14159265358979323846;
long double ld = 3.14159265358979323846L;
bool b = true;
void *ptr = NULL;
size_t sz = sizeof(int);
ptrdiff_t pd = (ptrdiff_t) &i - (ptrdiff_t) &c;
wchar_t wc = L'a';
wint_t wi = L'a';
char16_t c16 = u'a';
char32_t c32 = U'a';
complex float cf = 1.0f + 2.0fi;
complex double cd = 1.0 + 2.0i;
time_t t = time(NULL);
clock_t cl = clock();
FILE *file = NULL;
// 输出变量值
printf("char: %c\n", c);
printf("signed char: %hhd\n", sc);
printf("unsigned char: %hhu\n", uc);
printf("short: %hd\n", s);
printf("unsigned short: %hu\n", us);
printf("int: %d\n", i);
printf("unsigned int: %u\n", ui);
printf("long: %ld\n", l);
printf("unsigned long: %lu\n", ul);
printf("long long: %lld\n", ll);
printf("unsigned long long: %llu\n", ull);
printf("float: %f\n", f);
printf("double: %lf\n", d);
printf("long double: %Lf\n", ld);
printf("bool: %d\n", b);
printf("void *: %p\n", ptr);
printf("size_t: %zu\n", sz);
printf("ptrdiff_t: %td\n", pd);
printf("wchar_t: %lc\n", wc);
printf("wint_t: %lc\n", wi);
printf("char16_t: %lc\n", c16);
printf("char32_t: %lc\n", c32);
printf("complex float: %f + %fi\n", crealf(cf), cimagf(cf));
printf("complex double: %lf + %lfi\n", creal(cd), cimag(cd));
printf("time_t: %ld\n", (long) t);
printf("clock_t: %ld\n", (long) cl);
printf("FILE *: %p\n", file);
return 0;
}
```
该程序使用了 C 语言标准库中的头文件来定义不同类型的变量,并使用 printf 函数输出变量值。注意,对于某些类型,如时间和文件指针,输出的值可能会因环境和平台而异。
阅读全文
相关推荐



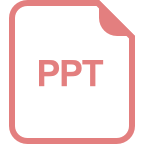



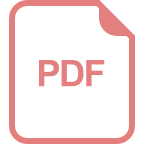
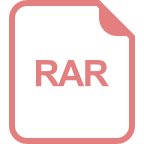
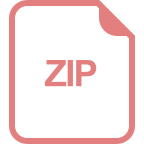
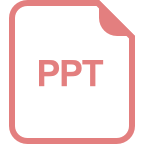
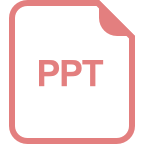
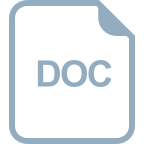
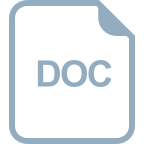