循环队列找队头下标实例代码
时间: 2024-10-13 22:00:37 浏览: 23
循环队列是一种特殊的线性表,它利用数组的特性,在最后一个元素之后添加新元素,并将第一个元素视为队头。当队列满时,再添加的新元素会覆盖掉第一个元素的位置,形成循环。找到队头下标的基本思路是维护两个指针,front表示队头,rear表示队尾。如果队列为空,front等于rear;若非空,则front总是指向下一个待访问的元素。
下面是一个简单的Java循环队列求队头下标的示例:
```java
public class CircularQueue {
private int[] queue;
private int front; // 队头指针
private int rear; // 队尾指针
private final int capacity;
public CircularQueue(int size) {
this.capacity = size;
queue = new int[capacity];
front = -1; // 空队列前端标记
rear = -1;
}
// 入队操作
public boolean enqueue(int value) {
if ((rear + 1) % capacity == front) { // 判断是否已满
return false;
}
rear = (rear + 1) % capacity; // 后移并更新队尾
queue[rear] = value;
return true;
}
// 出队操作(假设存在队首)
public int dequeue() {
if (front == -1) { // 空队列异常
throw new IllegalStateException("Queue is empty");
}
int removedValue = queue[front]; // 获取队首值
front = (front + 1) % capacity; // 更新队头
if (front == rear) { // 队列变为空
rear = -1;
}
return removedValue;
}
// 查找队头元素的下标(非阻塞查找)
public int getFrontIndex() {
if (front == -1) {
return -1; // 如果队列为空
} else {
return front; // 返回队头下标
}
}
}
```
在这个例子中,`getFrontIndex`方法用于直接返回队头的索引,如果没有队列(front == -1),则返回-1表示队列为空。
阅读全文
相关推荐
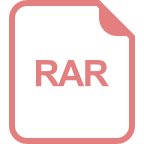
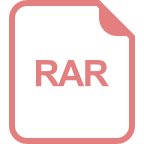
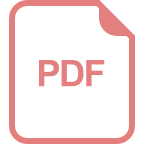















