用javafx实现猜数字游戏
时间: 2024-06-08 20:11:37 浏览: 13
好的,下面是一个简单的猜数字游戏的 JavaFX 实现示例:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.util.Random;
public class GuessNumberGame extends Application {
private int guessNumber;
private int guessCount;
private Label hintLabel;
private TextField guessTextField;
@Override
public void start(Stage primaryStage) throws Exception {
// 生成随机数
Random random = new Random();
guessNumber = random.nextInt(100) + 1;
// 创建 UI
Label titleLabel = new Label("猜数字游戏");
Label tipLabel = new Label("请输入 1~100 之间的整数:");
hintLabel = new Label("");
guessTextField = new TextField();
Button guessButton = new Button("猜");
guessButton.setOnAction(event -> guess());
HBox inputBox = new HBox(10, guessTextField, guessButton);
inputBox.setAlignment(Pos.CENTER);
VBox root = new VBox(20, titleLabel, tipLabel, inputBox, hintLabel);
root.setPadding(new Insets(20));
root.setAlignment(Pos.CENTER);
Scene scene = new Scene(root, 400, 300);
// 显示窗口
primaryStage.setScene(scene);
primaryStage.setTitle("猜数字游戏");
primaryStage.show();
}
private void guess() {
String guessText = guessTextField.getText();
if (guessText.isEmpty()) {
hintLabel.setText("请输入一个数!");
return;
}
int guess = 0;
try {
guess = Integer.parseInt(guessText);
} catch (NumberFormatException e) {
hintLabel.setText("请输入一个整数!");
return;
}
guessCount++;
if (guess == guessNumber) {
hintLabel.setText("恭喜你猜中了!共猜了 " + guessCount + " 次。");
guessTextField.setDisable(true);
} else if (guess < guessNumber) {
hintLabel.setText("猜小了,再试试!");
} else {
hintLabel.setText("猜大了,再试试!");
}
}
public static void main(String[] args) {
launch(args);
}
}
```
这个游戏会生成一个 1~100 之间的随机数,然后提示用户输入一个整数并猜测这个随机数是多少。如果猜中了,会显示恭喜信息并禁用输入框;如果猜错了,会提示用户再试一次并告诉用户猜的数字是偏大还是偏小。
相关推荐
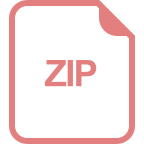
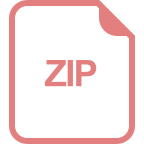
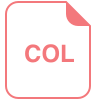
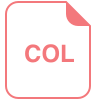
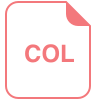
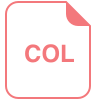
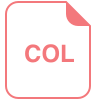









