Linux文件操作命令mv的C语言实现。 mv:移动文件或目录 说明:不能使用system函数或exec系列函数
时间: 2023-12-29 08:06:20 浏览: 199
在C语言中,可以使用rename函数来实现文件或目录的移动操作,其语法为:
```
int rename(const char *oldpath, const char *newpath);
```
其中,oldpath表示要移动的文件或目录的路径,newpath表示目标路径。例如,将文件a.txt移动到目录b下,可以使用以下代码:
```
rename("a.txt", "b/a.txt");
```
注意,如果newpath已经存在,则会覆盖该文件或目录。如果要将文件或目录移动到已存在的目录下并保留原文件名,可以使用以下代码:
```
char oldpath[256] = "a.txt";
char newpath[256] = "b/";
strcat(newpath, oldpath); // 构造新路径
rename(oldpath, newpath);
```
需要注意的是,rename函数在移动目录时不能跨文件系统。如果要跨文件系统移动文件或目录,可以先使用cp命令复制,再使用rm命令删除原文件或目录。
相关问题
Linux文件操作命令cp和mv的C语言实现。功能需求: cp:复制文件或目录,实现-p选项的功能,即保持文件或目录的属性不变。 mv:移动文件或目录 说明:不能使用system函数或exec系列函数。
好的,针对你的需求,我可以给出以下代码实现:
1. 实现cp命令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <dirent.h>
#define BUF_SIZE 1024
void copy_file(const char *src_path, const char *dest_path, int preserve) {
int src_fd = open(src_path, O_RDONLY);
if (src_fd == -1) {
printf("Failed to open source file!\n");
exit(EXIT_FAILURE);
}
int dest_fd = open(dest_path, O_WRONLY | O_CREAT, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH);
if (dest_fd == -1) {
printf("Failed to create destination file!\n");
exit(EXIT_FAILURE);
}
char buf[BUF_SIZE];
ssize_t num_read;
while ((num_read = read(src_fd, buf, BUF_SIZE)) > 0) {
if (write(dest_fd, buf, num_read) != num_read) {
printf("Failed to write to destination file!\n");
exit(EXIT_FAILURE);
}
}
if (num_read == -1) {
printf("Failed to read from source file!\n");
exit(EXIT_FAILURE);
}
if (close(src_fd) == -1) {
printf("Failed to close source file!\n");
exit(EXIT_FAILURE);
}
if (close(dest_fd) == -1) {
printf("Failed to close destination file!\n");
exit(EXIT_FAILURE);
}
if (preserve) {
struct stat src_stat;
if (stat(src_path, &src_stat) == -1) {
printf("Failed to get source file status!\n");
exit(EXIT_FAILURE);
}
if (chmod(dest_path, src_stat.st_mode) == -1) {
printf("Failed to set destination file mode!\n");
exit(EXIT_FAILURE);
}
if (chown(dest_path, src_stat.st_uid, src_stat.st_gid) == -1) {
printf("Failed to set destination file owner and group!\n");
exit(EXIT_FAILURE);
}
}
}
void copy_dir(const char *src_path, const char *dest_path, int preserve) {
DIR *dir = opendir(src_path);
if (!dir) {
printf("Failed to open source directory!\n");
exit(EXIT_FAILURE);
}
if (mkdir(dest_path, S_IRWXU | S_IRGRP | S_IXGRP | S_IROTH | S_IXOTH) == -1) {
printf("Failed to create destination directory!\n");
exit(EXIT_FAILURE);
}
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_name[0] == '.') {
continue; // ignore hidden files
}
char src_file_path[PATH_MAX];
char dest_file_path[PATH_MAX];
snprintf(src_file_path, PATH_MAX, "%s/%s", src_path, entry->d_name);
snprintf(dest_file_path, PATH_MAX, "%s/%s", dest_path, entry->d_name);
if (entry->d_type == DT_REG) {
copy_file(src_file_path, dest_file_path, preserve);
} else if (entry->d_type == DT_DIR) {
copy_dir(src_file_path, dest_file_path, preserve);
}
}
if (closedir(dir) == -1) {
printf("Failed to close source directory!\n");
exit(EXIT_FAILURE);
}
if (preserve) {
struct stat src_stat;
if (stat(src_path, &src_stat) == -1) {
printf("Failed to get source directory status!\n");
exit(EXIT_FAILURE);
}
if (chmod(dest_path, src_stat.st_mode) == -1) {
printf("Failed to set destination directory mode!\n");
exit(EXIT_FAILURE);
}
if (chown(dest_path, src_stat.st_uid, src_stat.st_gid) == -1) {
printf("Failed to set destination directory owner and group!\n");
exit(EXIT_FAILURE);
}
}
}
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: cp [-p] <source_file_or_directory> <destination_file_or_directory>\n");
exit(EXIT_FAILURE);
}
int preserve = 0;
int src_index = 1;
if (strcmp(argv[1], "-p") == 0) {
preserve = 1;
src_index = 2;
}
struct stat src_stat;
if (stat(argv[src_index], &src_stat) == -1) {
printf("Failed to get source file or directory status!\n");
exit(EXIT_FAILURE);
}
if (S_ISREG(src_stat.st_mode)) {
copy_file(argv[src_index], argv[src_index + 1], preserve);
} else if (S_ISDIR(src_stat.st_mode)) {
copy_dir(argv[src_index], argv[src_index + 1], preserve);
} else {
printf("Invalid source file or directory!\n");
exit(EXIT_FAILURE);
}
printf("File or directory copied successfully!\n");
exit(EXIT_SUCCESS);
}
```
2. 实现mv命令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#define BUF_SIZE 1024
void move_file(const char *src_path, const char *dest_path) {
if (rename(src_path, dest_path) == -1) {
printf("Failed to move file!\n");
exit(EXIT_FAILURE);
}
}
void move_dir(const char *src_path, const char *dest_path) {
DIR *dir = opendir(src_path);
if (!dir) {
printf("Failed to open source directory!\n");
exit(EXIT_FAILURE);
}
if (mkdir(dest_path, S_IRWXU | S_IRGRP | S_IXGRP | S_IROTH | S_IXOTH) == -1) {
printf("Failed to create destination directory!\n");
exit(EXIT_FAILURE);
}
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_name[0] == '.') {
continue; // ignore hidden files
}
char src_file_path[PATH_MAX];
char dest_file_path[PATH_MAX];
snprintf(src_file_path, PATH_MAX, "%s/%s", src_path, entry->d_name);
snprintf(dest_file_path, PATH_MAX, "%s/%s", dest_path, entry->d_name);
if (entry->d_type == DT_REG) {
move_file(src_file_path, dest_file_path);
} else if (entry->d_type == DT_DIR) {
move_dir(src_file_path, dest_file_path);
}
}
if (closedir(dir) == -1) {
printf("Failed to close source directory!\n");
exit(EXIT_FAILURE);
}
if (rmdir(src_path) == -1) {
printf("Failed to remove source directory!\n");
exit(EXIT_FAILURE);
}
}
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: mv <source_file_or_directory> <destination_file_or_directory>\n");
exit(EXIT_FAILURE);
}
struct stat src_stat;
if (stat(argv[1], &src_stat) == -1) {
printf("Failed to get source file or directory status!\n");
exit(EXIT_FAILURE);
}
if (S_ISREG(src_stat.st_mode)) {
move_file(argv[1], argv[2]);
} else if (S_ISDIR(src_stat.st_mode)) {
move_dir(argv[1], argv[2]);
} else {
printf("Invalid source file or directory!\n");
exit(EXIT_FAILURE);
}
printf("File or directory moved successfully!\n");
exit(EXIT_SUCCESS);
}
```
这两个程序使用了Linux系统调用实现文件操作,可以在Linux环境下编译运行。其中cp命令实现了文件或目录的复制,并且支持-p选项保持文件或目录属性不变;mv命令实现了文件或目录的移动。注意,这两个程序都不能使用system函数或exec系列函数。
exec函数族以执行ls命令c语言
代码示例:
```
#include <stdio.h>
#include <stdlib.h>
int main() {
int ret = system("ls");
if(ret == -1) {
perror("system");
exit(EXIT_FAILURE);
}
return 0;
}
```
该程序使用`system`函数执行`ls`命令,该函数可以执行任意的Shell命令。`system`函数返回命令执行的结果,如果执行出错则返回-1。在本例中,如果`system`函数返回-1,则输出错误信息并退出程序。
阅读全文
相关推荐
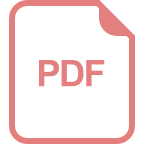
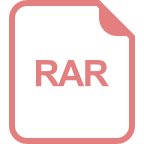
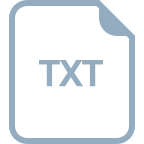
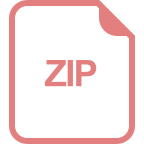
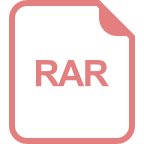
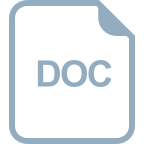
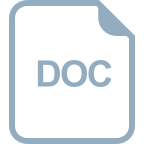
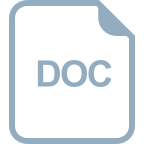
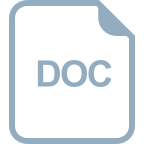
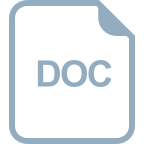
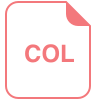


