输出所有三位数的水仙花数。 输入一个字符串,分别统计其中小写字母、大写字母和其他字符个数并输出。 找出1-50中是3的倍数、5的倍数、但不是3和5的共同倍数的数。 输入一个百分制成绩(整数成绩),输出对应五分制等级。
时间: 2024-10-14 16:02:35 浏览: 22
首先,关于三位数的水仙花数,水仙花数是指一个三位数,其各位数字立方之和等于该数本身。例如,153就是水仙花数,因为1^3 + 5^3 + 3^3 = 153。我们可以编写一个程序来寻找所有的三位水仙花数:
```python
def find_water_lily_numbers():
for num in range(100, 1000):
if str(num) == str(sum(int(digit)**3 for digit in str(num))):
print(num)
find_water_lily_numbers()
```
对于第二个任务,统计字符串中字母和其他字符的数量:
```python
def count_chars(s):
lower_count = sum(1 for c in s if c.islower())
upper_count = sum(1 for c in s if c.isupper())
other_count = len(s) - lower_count - upper_count
return lower_count, upper_count, other_count
input_string = "Hello World!"
count = count_chars(input_string)
print(f"小写字母: {count[0]}, 大写字母: {count[1]}, 其他字符: {count[2]}")
```
第三个任务,找出1-50之间符合条件的数:
```python
def find_numbers_divisible(n):
result = []
for i in range(1, 51):
if i % 3 == 0 and i % 5 == 0 and i % 15 != 0:
result.append(i)
return result
numbers = find_numbers_divisible(50)
print("符合条件的数:", numbers)
```
第四个任务,将百分制成绩转换为五分制:
```python
def convert_to_fives(score):
if score >= 90:
return 5
elif score >= 80:
return 4
# ... 分别处理其他范围的分数...
else:
return 1
score = 85
fives_grade = convert_to_fives(score)
print(f"{score}分对应五分制等级为:{fives_grade}")
```
阅读全文
相关推荐
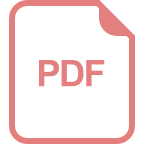
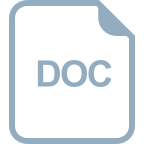
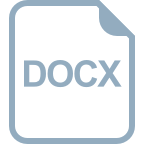

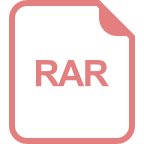
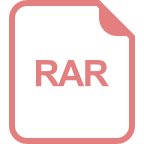
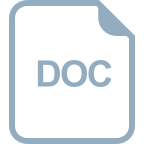
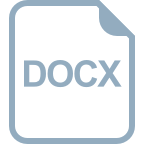
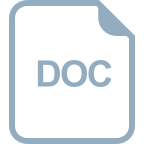
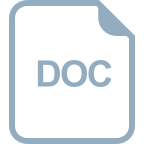
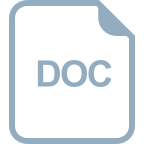
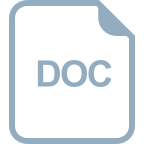
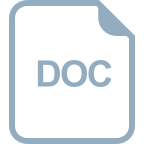
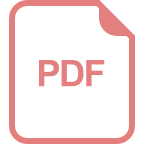
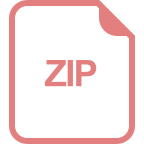
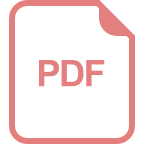
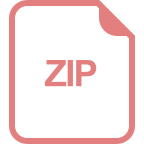