gcn cluster
时间: 2023-08-16 11:09:30 浏览: 54
GCN(Graph Convolutional Networks)可以用于图聚类(Graph Clustering)任务,即将图中的节点分为多个簇或群组。在GCN中,每个节点都有一个向量表示,这个向量包含了节点的特征信息。通过GCN的卷积操作,可以将每个节点的特征进行聚合和更新,从而实现对整个图的特征提取和表示。这些特征可以用于图聚类任务,其中可以使用聚类算法(如k-means)将节点分为多个簇。这些簇可以代表不同的社区、子图或者模块,从而帮助我们理解和分析图的结构和特征。因此,GCN被广泛应用于社交网络分析、药物发现、推荐系统、图像分类等领域中的图聚类任务。
相关问题
gcn cluster code
以下是使用GCN实现图聚类的代码示例,代码使用Python和PyTorch实现:
```
import torch
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
# 定义GCN模型
class GCN(torch.nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(GCN, self).__init__()
self.conv1 = GCNConv(input_dim, hidden_dim)
self.conv2 = GCNConv(hidden_dim, output_dim)
def forward(self, x, edge_index):
x = F.relu(self.conv1(x, edge_index))
x = self.conv2(x, edge_index)
return x
# 定义聚类函数
def k_means(x, k):
centroids = x[:k, :].clone()
while True:
distances = torch.cdist(x, centroids)
_, cluster = distances.min(dim=1)
new_centroids = torch.stack([x[cluster == i, :].mean(dim=0) for i in range(k)])
if torch.equal(new_centroids, centroids):
break
centroids = new_centroids
return cluster
# 加载图数据
data = ... # 从文件或数据库中读取
# 初始化GCN模型
model = GCN(input_dim=data.num_features, hidden_dim=16, output_dim=8)
# 训练模型
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
for epoch in range(100):
model.train()
optimizer.zero_grad()
x = model(data.x, data.edge_index)
loss = F.mse_loss(x, data.y)
loss.backward()
optimizer.step()
# 聚类节点
with torch.no_grad():
model.eval()
x = model(data.x, data.edge_index)
clusters = k_means(x, k=4)
# 输出结果
print(clusters)
```
上述代码中,我们首先定义了一个GCN模型,并使用PyTorch中的GCNConv层实现了卷积操作。然后,我们定义了一个聚类函数k_means,该函数使用k-means算法对节点进行聚类。在模型训练完成后,我们使用训练好的GCN模型对节点进行特征提取,并使用k-means算法对节点进行聚类。最后,我们输出了聚类结果。
gcn pytorch
GCN(Graph Convolutional Network)是一种用于图数据的深度学习模型,它可以对节点和边进行特征学习和预测。GCN在图神经网络领域具有重要的应用价值。
GCN的PyTorch实现可以使用PyTorch Geometric库来实现。PyTorch Geometric是一个专门用于处理图数据的PyTorch扩展库,提供了一系列用于构建和训练图神经网络的工具和函数。
在PyTorch Geometric中,可以使用torch_geometric.nn模块中的GCNConv类来定义GCN层。GCNConv类实现了GCN的前向传播过程,可以根据输入的节点特征和图结构进行特征学习和传播。
以下是一个简单的GCN模型的示例代码:
```python
import torch
import torch.nn as nn
from torch_geometric.nn import GCNConv
class GCN(nn.Module):
def __init__(self, num_features, num_classes):
super(GCN, self).__init__()
self.conv1 = GCNConv(num_features, 16)
self.conv2 = GCNConv(16, num_classes)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = torch.relu(x)
x = self.conv2(x, edge_index)
return x
# 构建模型
model = GCN(num_features, num_classes)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
# 训练模型
for epoch in range(num_epochs):
# 前向传播
output = model(x, edge_index)
loss = criterion(output, y)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
在上述代码中,GCN类定义了一个简单的两层GCN模型,输入节点特征的维度为num_features,输出类别的数量为num_classes。模型的前向传播过程中使用了两个GCNConv层,并通过ReLU激活函数进行非线性变换。训练过程中使用交叉熵损失函数和Adam优化器进行模型的优化。
希望以上内容对你有所帮助!
相关推荐
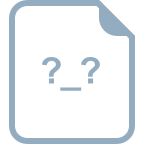
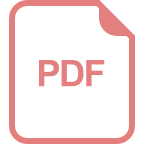












