可以使用pyg(torch_geometric)搭建GCN网络
时间: 2024-09-30 17:02:25 浏览: 69
PyG(Torch Geometric)是一个用于图神经网络(Graph Neural Networks, GNNs)的Python库,它是基于PyTorch构建的。如果你想使用PyG搭建GCN(Graph Convolutional Network)网络,首先需要安装`torch_geometric`库。以下是一个简单的步骤概述:
1. **安装**:
```bash
pip install torch torchvision torchaudio -f https://download.pytorch.org/whl/torch_stable.html
pip install torch-scatter -f https://github.com/rusty1s/pytorch_scatter/blobs/main/dist/
pip install torch-sparse -f https://github.com/rusty1s/pytorch_sparse/blobs/main/dist/
pip install torch-cluster -f https://github.com/rusty1s/pytorch_cluster/blobs/main/dist/
pip install torch-geometric
```
2. **导入所需模块**:
```python
import torch
from torch_geometric.nn import GCNConv, global_mean_pool
```
3. **构建数据结构**:
使用`torch_geometric.data.Data`类来创建图的数据结构,包括节点特征和边的信息。
4. **定义模型**:
```python
class GCN(torch.nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GCN, self).__init__()
self.conv1 = GCNConv(in_channels, hidden_channels)
self.conv2 = GCNConv(hidden_channels, out_channels)
def forward(self, data):
x, edge_index = data.x, data.edge_index
x = F.relu(self.conv1(x, edge_index))
x = self.conv2(x, edge_index)
return global_mean_pool(x, data.batch)
```
5. **训练和预测**:
定义损失函数、优化器,并通过`DataLoader`加载数据进行训练。
```python
model = GCN(...).to(device)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
for epoch in range(100): # 假设这是训练循环
optimizer.zero_grad()
output = model(data)
loss = F.nll_loss(output[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
# 预测阶段
with torch.no_grad():
pred = model(data)
```
阅读全文
相关推荐
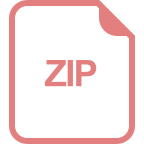
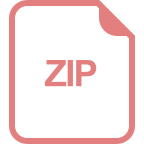
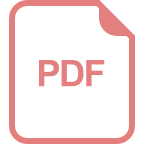













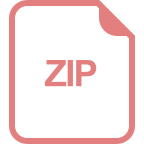
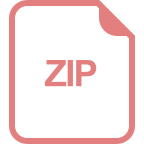
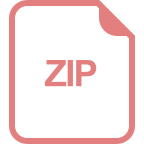