图卷积网络进行骨骼识别代码_PyG训练图卷积网络GCN的代码参考
时间: 2024-06-08 12:06:26 浏览: 8
下面是使用PyG训练图卷积网络GCN进行骨骼识别的代码参考:
首先,需要安装PyG和其他必要的库:
```python
!pip install torch torchvision torchaudio
!pip install torch-scatter -f https://pytorch-geometric.com/whl/torch-1.7.0+${CUDA}.html
!pip install torch-sparse -f https://pytorch-geometric.com/whl/torch-1.7.0+${CUDA}.html
!pip install torch-cluster -f https://pytorch-geometric.com/whl/torch-1.7.0+${CUDA}.html
!pip install torch-spline-conv -f https://pytorch-geometric.com/whl/torch-1.7.0+${CUDA}.html
!pip install torch-geometric
```
接下来,加载数据集:
```python
import torch
from torch_geometric.datasets import HumanSkeletonDataset
train_dataset = HumanSkeletonDataset(root='./data', split='train')
test_dataset = HumanSkeletonDataset(root='./data', split='test')
```
然后,定义模型:
```python
from torch_geometric.nn import GCNConv
import torch.nn.functional as F
class GCN(torch.nn.Module):
def __init__(self, num_features, hidden_channels, num_classes):
super(GCN, self).__init__()
self.conv1 = GCNConv(num_features, hidden_channels)
self.conv2 = GCNConv(hidden_channels, num_classes)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return x
```
接下来,定义训练过程:
```python
import torch.optim as optim
from torch_geometric.data import DataLoader
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
def train(model, train_loader, optimizer):
model.train()
for data in train_loader:
data = data.to(device)
optimizer.zero_grad()
out = model(data.x, data.edge_index)
loss = F.cross_entropy(out, data.y)
loss.backward()
optimizer.step()
def test(model, loader):
model.eval()
correct = 0
for data in loader:
data = data.to(device)
with torch.no_grad():
pred = model(data.x, data.edge_index).argmax(dim=1)
correct += int((pred == data.y).sum())
return correct / len(loader.dataset)
```
最后,训练模型并测试:
```python
model = GCN(num_features=3, hidden_channels=16, num_classes=15).to(device)
optimizer = optim.Adam(model.parameters(), lr=0.01)
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=64, shuffle=False)
for epoch in range(1, 201):
train(model, train_loader, optimizer)
train_acc = test(model, train_loader)
test_acc = test(model, test_loader)
print(f'Epoch: {epoch:03d}, Train Acc: {train_acc:.4f}, Test Acc: {test_acc:.4f}')
```
这是一个简单的示例,你需要根据自己的数据集和需求进行修改和调整。
相关推荐
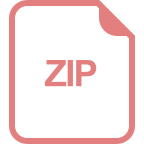
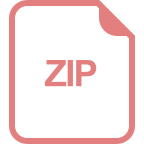












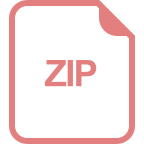
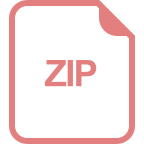