r语言用sample.split划分数据集,如何做=写回归森林的代码
时间: 2023-05-08 13:58:05 浏览: 293
在使用R语言的sample.split函数划分数据集后,我们可以通过以下步骤来构建回归森林:
1. 导入所需库
首先,我们需要使用以下代码导入randomForest库:
library(randomForest)
2. 读取数据
接下来,我们需要使用以下代码读取我们划分好的训练集和测试集数据:
train_data <- read.csv("train_data.csv")
test_data <- read.csv("test_data.csv")
3. 训练模型
现在,我们可以使用以下代码来训练回归森林模型:
rf_model <- randomForest(y ~ ., data = train_data, ntree = 500)
在这里,y是我们要预测的变量,"."表示使用其余所有变量来预测y变量。
4. 预测结果
最后,我们可以使用以下代码,将我们的测试数据集用于我们刚刚训练好的模型进行预测:
predicted_vals <- predict(rf_model, test_data)
这个代码会将预测结果存在predicted_vals变量里,可以与测试数据集的结果进行对比,以评估模型的准确性。
总体而言,使用R语言的sample.split函数划分数据集,构建回归森林模型的流程主要包括导入所需库、读取数据、训练模型和预测结果这四个步骤。
相关问题
R语言中sample.split在哪个包
在R语言中,sample.split函数是在caTools包中。可以使用以下命令安装并加载该包:
```r
install.packages("caTools")
library(caTools)
```
然后就可以使用sample.split函数了。
sample.split
sample.split是一个在R语言中常用的函数,用于将数据集分为训练集和测试集。它可以根据指定的比例或样本数将数据集划分为两部分。
使用sample.split函数的基本语法如下:
```
sample.split(data, SplitRatio, Seed)
```
其中,data是要进行划分的数据集,SplitRatio是训练集所占的比例(0-1之间),Seed是随机数生成器的种子(可选参数)。
这个函数的返回值是一个逻辑向量,长度与输入数据集相等。它将根据SplitRatio的比例为每个样本生成TRUE或FALSE的值,表示该样本属于训练集(TRUE)或测试集(FALSE)。
以下是一个示例:
```R
# 加载需要划分的数据集
library(caTools)
data(iris)
# 将数据集划分为训练集和测试集,比例为0.7
split <- sample.split(iris$Species, SplitRatio = 0.7)
# 根据划分结果获取训练集和测试集
train <- subset(iris, split == TRUE)
test <- subset(iris, split == FALSE)
# 输出训练集和测试集的行数
nrow(train)
nrow(test)
```
这样就可以将数据集iris按照0.7的比例划分为训练集和测试集。
相关推荐
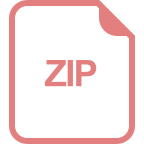
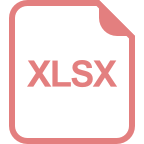













