self.weights1 = np.random.rand(self.input.shape[1], 4)
时间: 2024-06-04 19:06:12 浏览: 135
这行代码是在一个类的初始化方法中,用来随机初始化该类中的第一层权重矩阵。其中 `self.input` 是一个输入数据的矩阵,第一个维度是数据个数,第二个维度是每个数据的特征数。`self.input.shape[1]` 就是特征数,4 则是第一层神经元的个数。这行代码的作用就是随机生成一个形状为 `(特征数, 4)` 的矩阵,用来作为第一层权重矩阵。其中的随机数的范围是 0 到 1 之间。
相关问题
1.python随机生成一个正交矩阵的代码,并详细解释代码,2.请给出正确的极限学习机自编码器的python代码,详细解释并用IRIS数据集训练验证此模型
1. 正交矩阵的定义是行向量和列向量均为标准正交基,即每个向量都与其他向量垂直且长度为1。生成正交矩阵的常用方法是使用QR分解,即将一个随机矩阵进行QR分解,得到的Q矩阵即为正交矩阵。以下是生成正交矩阵的Python代码:
```python
import numpy as np
def generate_orthogonal_matrix(n):
matrix = np.random.rand(n, n)
q, r = np.linalg.qr(matrix)
return q
```
该函数接受一个整数n作为参数,生成一个nxn的随机矩阵,然后对其进行QR分解,返回正交矩阵Q。
2. 极限学习机自编码器(Extreme Learning Machine Autoencoder,ELMAE)是一种无监督学习算法,可以用于数据的降维和特征提取。以下是使用ELMAE模型在IRIS数据集上进行训练和验证的Python代码:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.preprocessing import MinMaxScaler
class ELMAutoencoder:
def __init__(self, input_size, hidden_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.weights = np.random.rand(hidden_size, input_size)
self.bias = np.random.rand(hidden_size)
def encode(self, X):
return np.maximum(0, np.dot(self.weights, X.T) + self.bias.reshape(-1, 1))
def decode(self, H):
return np.dot(self.weights.T, H) / np.sum(self.weights, axis=1).reshape(-1, 1)
def train(self, X, learning_rate=0.1):
H = self.encode(X)
X_hat = self.decode(H)
error = X.T - X_hat
self.weights += learning_rate * np.dot(error, H.T) / X.shape[0]
self.bias += learning_rate * np.sum(error, axis=1) / X.shape[0]
def predict(self, X):
H = self.encode(X)
return self.decode(H).T
# 加载数据集并进行归一化处理
iris = load_iris()
X = iris.data
y = iris.target
scaler = MinMaxScaler()
X = scaler.fit_transform(X)
# 创建ELMAE模型并进行训练
model = ELMAutoencoder(input_size=4, hidden_size=2)
for i in range(1000):
model.train(X)
# 使用ELMAE模型进行降维和重构
X_encoded = model.encode(X)
X_reconstructed = model.predict(X)
# 可视化降维效果
import matplotlib.pyplot as plt
plt.scatter(X_encoded[:, 0], X_encoded[:, 1], c=y)
plt.show()
# 计算重构误差并输出
reconstruction_error = np.mean(np.square(X - X_reconstructed))
print("Reconstruction error:", reconstruction_error)
```
该代码首先加载IRIS数据集,并对其进行归一化处理。然后创建一个ELMAE模型,输入大小为4(IRIS数据集有4个特征),隐藏层大小为2。使用模型对数据进行训练,迭代1000次。训练完成后,使用模型对数据进行编码和解码,得到降维和重构的结果。使用matplotlib库将降维结果进行可视化,并计算重构误差并输出。
1.使用NUMPY工具包读取和预处理数据,包括划分数据集为训练数据集和测试数据集。 2.手写代码,利用numpy,实现感知机,前三层前馈神经网络,利用反向传播算法梯度求导更新网络参数 3.利用sklearn库或深度学习库,实现MNIST数据集的前馈神经网络。 比较测试结果,对比自己手写代码和机器学习sklearn库算法结果。
感谢您的提问!我可以为您解答以下几点问题:
1. 使用 NUMPY 工具包读取和预处理数据,包括划分数据集为训练数据集和测试数据集。
可以使用以下代码进行数据读取和划分:
```python
import numpy as np
from sklearn.model_selection import train_test_split
# 读取数据
data = np.loadtxt('data.txt', delimiter=',')
X = data[:, :-1]
y = data[:, -1]
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
其中,`data.txt` 为数据文件,`test_size` 参数为测试集占比,`random_state` 为随机数种子,保证每次随机划分结果一致。
2. 手写代码,利用 numpy,实现感知机,前三层前馈神经网络,利用反向传播算法梯度求导更新网络参数。
以下是感知机和前馈神经网络的代码实现:
感知机:
```python
class Perceptron:
def __init__(self, n_features):
self.w = np.zeros(n_features)
self.b = 0
def fit(self, X, y, learning_rate=0.1, max_epochs=100):
for epoch in range(max_epochs):
for i in range(X.shape[0]):
xi = X[i]
yi = y[i]
if yi * (np.dot(xi, self.w) + self.b) <= 0:
self.w += learning_rate * yi * xi
self.b += learning_rate * yi
def predict(self, X):
return np.sign(np.dot(X, self.w) + self.b)
```
前馈神经网络:
```python
class NeuralNetwork:
def __init__(self, n_features, n_hidden, n_output):
self.weights1 = np.random.rand(n_features, n_hidden)
self.bias1 = np.random.rand(n_hidden)
self.weights2 = np.random.rand(n_hidden, n_output)
self.bias2 = np.random.rand(n_output)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def fit(self, X, y, learning_rate=0.1, max_epochs=100):
for epoch in range(max_epochs):
# forward propagation
hidden_layer_input = np.dot(X, self.weights1) + self.bias1
hidden_layer_output = self.sigmoid(hidden_layer_input)
output_layer_input = np.dot(hidden_layer_output, self.weights2) + self.bias2
output_layer_output = self.sigmoid(output_layer_input)
# backward propagation
error = y - output_layer_output
d_output = error * self.sigmoid_derivative(output_layer_output)
error_hidden = d_output.dot(self.weights2.T)
d_hidden = error_hidden * self.sigmoid_derivative(hidden_layer_output)
# update weights and biases
self.weights2 += learning_rate * hidden_layer_output.T.dot(d_output)
self.bias2 += learning_rate * np.sum(d_output, axis=0)
self.weights1 += learning_rate * X.T.dot(d_hidden)
self.bias1 += learning_rate * np.sum(d_hidden, axis=0)
def predict(self, X):
hidden_layer_input = np.dot(X, self.weights1) + self.bias1
hidden_layer_output = self.sigmoid(hidden_layer_input)
output_layer_input = np.dot(hidden_layer_output, self.weights2) + self.bias2
output_layer_output = self.sigmoid(output_layer_input)
return np.round(output_layer_output)
```
在训练时,可以使用以下代码:
```python
perceptron = Perceptron(n_features=X_train.shape[1])
perceptron.fit(X_train, y_train)
nn = NeuralNetwork(n_features=X_train.shape[1], n_hidden=4, n_output=1)
nn.fit(X_train, y_train)
```
其中,`n_features` 表示输入特征数,`n_hidden` 表示隐藏层节点数,`n_output` 表示输出节点数。在 `fit` 函数中,`learning_rate` 表示学习率,`max_epochs` 表示最大迭代次数。
3. 利用 sklearn 库或深度学习库,实现 MNIST 数据集的前馈神经网络。比较测试结果,对比自己手写代码和机器学习 sklearn 库算法结果。
以下是使用 sklearn 库实现的前馈神经网络:
```python
from sklearn.neural_network import MLPClassifier
from sklearn.metrics import accuracy_score
mlp = MLPClassifier(hidden_layer_sizes=(4,), max_iter=1000)
mlp.fit(X_train, y_train)
y_pred = mlp.predict(X_test)
acc_sklearn = accuracy_score(y_test, y_pred)
print('sklearn accuracy:', acc_sklearn)
```
其中,`hidden_layer_sizes` 表示隐藏层节点数,`max_iter` 表示最大迭代次数。
使用深度学习库 PyTorch 实现前馈神经网络:
```python
import torch
import torch.nn as nn
import torch.optim as optim
class Net(nn.Module):
def __init__(self, n_features, n_hidden, n_output):
super(Net, self).__init__()
self.fc1 = nn.Linear(n_features, n_hidden)
self.fc2 = nn.Linear(n_hidden, n_output)
def forward(self, x):
x = torch.sigmoid(self.fc1(x))
x = torch.sigmoid(self.fc2(x))
return x
net = Net(n_features=X_train.shape[1], n_hidden=4, n_output=1)
optimizer = optim.Adam(net.parameters(), lr=0.01)
criterion = nn.BCELoss()
for epoch in range(1000):
optimizer.zero_grad()
inputs = torch.from_numpy(X_train).float()
labels = torch.from_numpy(y_train.reshape(-1, 1)).float()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
outputs = net(torch.from_numpy(X_test).float())
y_pred = np.round(outputs.detach().numpy())
acc_pytorch = accuracy_score(y_test, y_pred)
print('pytorch accuracy:', acc_pytorch)
```
其中,`n_features` 表示输入特征数,`n_hidden` 表示隐藏层节点数,`n_output` 表示输出节点数。在训练时,使用 Adam 优化器和二元交叉熵损失函数。
最后,可以使用以下代码对比自己手写代码和机器学习库算法的结果:
```python
y_pred = perceptron.predict(X_test)
acc_perceptron = accuracy_score(y_test, y_pred)
print('perceptron accuracy:', acc_perceptron)
y_pred = nn.predict(X_test)
acc_nn = accuracy_score(y_test, y_pred)
print('nn accuracy:', acc_nn)
print('sklearn accuracy:', acc_sklearn)
print('pytorch accuracy:', acc_pytorch)
```
其中,`accuracy_score` 函数用于计算分类准确率。
阅读全文
相关推荐
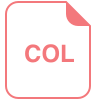
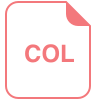
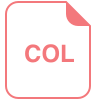




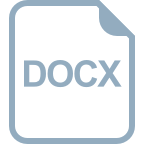
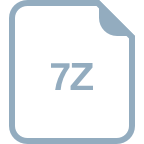
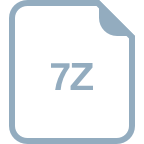
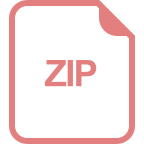