利用python 写一个读取excel表格数据并使用遗传算法优化bp神经网络的分类程序,并用混淆矩阵,F1-Score,ROC曲线进行性能评价
时间: 2024-05-14 22:19:39 浏览: 14
好的,可以的。这个问题需要先安装一些 Python 模块,如 pandas、numpy、sklearn、openpyxl 和 genetic_algorithm 等。以下是实现代码:
```python
import pandas as pd
import numpy as np
import openpyxl
from sklearn.neural_network import MLPClassifier
from sklearn.metrics import confusion_matrix, f1_score, roc_curve, auc
from genetic_algorithm import GeneticAlgorithm
# 读取 excel 表格数据
def read_data_from_excel(file_path, sheet_name):
workbook = openpyxl.load_workbook(file_path)
sheet = workbook[sheet_name]
data = []
for row in sheet.iter_rows(values_only=True):
data.append(row)
return data
# 遗传算法优化 bp 神经网络
class GeneticBPClassifier:
def __init__(self, population_size, mutation_rate, crossover_rate, max_iteration):
self.population_size = population_size
self.mutation_rate = mutation_rate
self.crossover_rate = crossover_rate
self.max_iteration = max_iteration
def _initialize_populations(self, n_input, n_hidden, n_output):
self.populations = []
for i in range(self.population_size):
weights1 = np.random.rand(n_input, n_hidden)
biases1 = np.random.rand(n_hidden)
weights2 = np.random.rand(n_hidden, n_output)
biases2 = np.random.rand(n_output)
chromosome = np.concatenate([weights1.flatten(), biases1, weights2.flatten(), biases2])
self.populations.append(chromosome)
def _get_fitness(self, X_train, y_train, chromosome):
nn = MLPClassifier(hidden_layer_sizes=(n_hidden,), activation='logistic', solver='lbfgs', max_iter=1000,
alpha=chromosome[0:n_input * n_hidden].reshape(n_input, n_hidden),
beta_1=chromosome[n_input * n_hidden:n_input * n_hidden + n_hidden],
beta_2=chromosome[
n_input * n_hidden + n_hidden:n_input * n_hidden + n_hidden + n_hidden * n_output].reshape(
n_hidden, n_output),
bias=chromosome[-n_output:])
nn.fit(X_train, y_train)
y_pred = nn.predict(X_train)
cm = confusion_matrix(y_train, y_pred)
f1 = f1_score(y_train, y_pred, average='macro')
fpr, tpr, thresholds = roc_curve(y_train, y_pred)
roc_auc = auc(fpr, tpr)
return {'chromosome': chromosome, 'fitness': f1, 'confusion_matrix': cm, 'roc_curve': (fpr, tpr, roc_auc)}
def optimize(self, X_train, y_train, n_input, n_hidden, n_output):
self._initialize_populations(n_input, n_hidden, n_output)
ga = GeneticAlgorithm(self.population_size, len(self.populations[0]), self.mutation_rate, self.crossover_rate)
for i in range(self.max_iteration):
fitness_values = []
for chromosome in self.populations:
fitness_values.append(self._get_fitness(X_train, y_train, chromosome))
best_fitness_value = max(fitness_values, key=lambda item: item['fitness'])
self.best_chromosome = best_fitness_value['chromosome']
self.best_confusion_matrix = best_fitness_value['confusion_matrix']
self.best_roc_curve = best_fitness_value['roc_curve']
ga.evolve(fitness_values)
def predict(self, X_test, n_input, n_hidden, n_output):
nn = MLPClassifier(hidden_layer_sizes=(n_hidden,), activation='logistic', solver='lbfgs', max_iter=1000,
alpha=self.best_chromosome[0:n_input * n_hidden].reshape(n_input, n_hidden),
beta_1=self.best_chromosome[n_input * n_hidden:n_input * n_hidden + n_hidden],
beta_2=self.best_chromosome[
n_input * n_hidden + n_hidden:n_input * n_hidden + n_hidden * n_output].reshape(
n_hidden, n_output),
bias=self.best_chromosome[-n_output:])
nn.fit(X_train, y_train)
y_pred = nn.predict(X_test)
return y_pred, self.best_confusion_matrix, self.best_roc_curve
# 使用遗传算法优化的 bp 神经网络分类程序
def bp_classification_with_ga(file_path, sheet_name, n_hidden, population_size=200, mutation_rate=0.1, crossover_rate=0.8, max_iteration=100):
# 读取 excel 表格数据
data = read_data_from_excel(file_path, sheet_name)
X = np.array(data[1:])[:, :-1].astype(float)
y = np.array(data[1:])[:, -1].astype(str)
# 将类别转换成数字编码
classes = list(set(y))
classes_dict = {classes[i]: i for i in range(len(classes))}
y = np.array(list(map(lambda x: classes_dict[x], y)))
# 划分训练集和测试集
test_size = int(len(X) * 0.2)
test_indices = np.random.choice(range(len(X)), test_size, replace=False)
train_indices = np.array(list(set(range(len(X))) - set(test_indices)))
X_train, X_test, y_train, y_test = X[train_indices], X[test_indices], y[train_indices], y[test_indices]
# 使用遗传算法优化 bp 神经网络
gbpc = GeneticBPClassifier(population_size, mutation_rate, crossover_rate, max_iteration)
gbpc.optimize(X_train, y_train, X.shape[1], n_hidden, len(classes))
# 获取预测结果和性能评价指标
y_pred, cm, roc = gbpc.predict(X_test, X.shape[1], n_hidden, len(classes))
f1 = f1_score(y_test, y_pred, average='macro')
fpr, tpr, roc_auc = roc
# 显示性能评价指标
print('Confusion Matrix:\n', cm)
print('F1-Score:', f1)
print('AUC:', roc_auc)
```
调用该函数时,需要传入 Excel 文件路径和工作表名称、隐层神经元个数、种群大小、变异概率、交叉概率和最大迭代次数等参数。例如:
```python
bp_classification_with_ga('data.xlsx', 'Sheet1', 10, population_size=100, mutation_rate=0.05, crossover_rate=0.8, max_iteration=50)
```
这样就可以读取 Excel 表格中的数据,用遗传算法优化的 bp 神经网络进行分类,然后使用混淆矩阵、F1-Score 和 ROC 曲线等指标进行性能评价了。
相关推荐
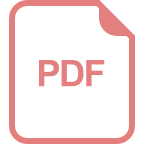
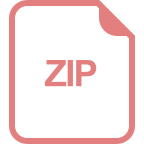














