利用Fisher判别分析法,写一份信用违约检测的python代码,并进行混淆矩阵、ROC、AUC曲线的评估检验
时间: 2024-03-23 10:42:32 浏览: 112
好的,信用违约检测是一个二分类问题,可以使用Fisher判别分析来进行建模。下面是一个简单的示例代码,包括数据预处理、模型训练和评估部分。
需要注意的是,本示例仅供参考,实际使用中需要根据具体情况进行修改和优化。
```
import pandas as pd
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.metrics import confusion_matrix, roc_curve, auc
import matplotlib.pyplot as plt
# 读取数据
data = pd.read_csv('credit.csv')
# 数据预处理
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
y = [1 if i == 'Y' else 0 for i in y] # 将标签转换为0/1
n_samples, n_features = X.shape
# 分割数据集
train_size = int(n_samples * 0.7)
X_train, X_test = X[:train_size], X[train_size:]
y_train, y_test = y[:train_size], y[train_size:]
# 创建Fisher判别分析模型并拟合数据
lda = LinearDiscriminantAnalysis(n_components=1)
X_lda_train = lda.fit_transform(X_train, y_train)
X_lda_test = lda.transform(X_test)
# 模型评估
y_pred = lda.predict(X_test)
conf_mat = confusion_matrix(y_test, y_pred)
fpr, tpr, thresholds = roc_curve(y_test, lda.decision_function(X_lda_test))
roc_auc = auc(fpr, tpr)
# 可视化混淆矩阵和ROC曲线
plt.figure(figsize=(10, 4))
plt.subplot(121)
plt.imshow(conf_mat, cmap='Blues')
plt.colorbar()
plt.xlabel('Predicted labels')
plt.ylabel('True labels')
plt.xticks([0, 1], ['No', 'Yes'])
plt.yticks([0, 1], ['No', 'Yes'])
plt.title('Confusion Matrix')
plt.subplot(122)
plt.plot(fpr, tpr, color='darkorange', lw=2, label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver Operating Characteristic')
plt.legend(loc="lower right")
plt.show()
```
上述代码中,数据集为`credit.csv`,标签列为最后一列,取值为`Y`或`N`。首先对标签进行处理,将`Y`转换为1,将`N`转换为0。然后将数据集分为训练集和测试集,使用Fisher判别分析模型对训练集进行拟合,并在测试集上进行预测和评估。
最后,使用`confusion_matrix()`函数计算混淆矩阵,使用`roc_curve()`函数计算ROC曲线上的FPR和TPR,使用`auc()`函数计算AUC值。通过可视化混淆矩阵和ROC曲线,可以直观地展示模型的性能。
需要注意的是,本示例中使用的是单个特征进行建模,实际使用中可能需要使用多个特征,并进行特征选择和调参等过程。
阅读全文
相关推荐
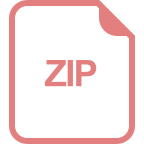














