router.GET("/", func(c *gin.Context) { id := c.Query("id") ret := res(id) c.JSON(http.StatusOK, gin.H{ "msg": ret, }
时间: 2024-04-27 18:25:20 浏览: 117
这段代码是一个路由处理函数,当请求的URL路径为"/"时,会执行这个函数。函数中使用了c.Query("id")来获取URL参数中名为"id"的参数值,并将其赋值给变量id。接着,使用函数res(id)来处理这个参数,并将处理结果赋值给变量ret。最后,使用c.JSON()函数将处理结果以JSON格式返回给客户端。其中,使用http.StatusOK指定HTTP状态码为200,表示请求成功。
需要注意的是,这段代码中的变量和函数res()都是没有定义的,你需要根据具体的业务逻辑来定义和实现它们。另外,这个路由处理函数中只处理了GET请求,如果需要处理其他HTTP请求方法,可以使用类似的代码结构,并将router.GET()改为对应的方法,例如router.POST()、router.PUT()等。
相关问题
router.POST("/post", func(c *gin.Context) { id := c.Query("id") page := c.DefaultQuery("page", "0") name := c.PostForm("name") message := c.PostForm("message") }这是Gin框架中的一段代码,发送的JSON数据怎么写?使用的根据是APIFOX
要发送JSON数据,可以使用`c.JSON()`方法将JSON数据作为响应返回给客户端。以下是将JSON数据作为响应发送的示例代码:
```go
type Response struct {
ID string `json:"id"`
Message string `json:"message"`
}
func main() {
router := gin.Default()
router.POST("/post", func(c *gin.Context) {
// 解析请求JSON数据到结构体
var requestData struct {
ID string `json:"id"`
Name string `json:"name"`
Message string `json:"message"`
}
if err := c.ShouldBindJSON(&requestData); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
// 处理请求数据
response := Response{
ID: requestData.ID,
Message: requestData.Message,
}
// 返回JSON响应
c.JSON(http.StatusOK, response)
})
router.Run(":8080")
}
```
在上面的示例中,我们定义了一个结构体`Response`来表示响应数据的结构。在处理POST请求时,使用`c.ShouldBindJSON()`方法将请求的JSON数据绑定到`requestData`结构体上。然后,根据需要处理请求数据,并将响应数据填充到`response`结构体中。最后,使用`c.JSON()`方法将响应数据作为JSON格式返回给客户端。
对于发送JSON数据的请求,可以使用工具(如Postman、curl等)来发送HTTP请求,将请求体中的数据以JSON格式发送即可。例如,使用curl发送POST请求并发送JSON数据的命令如下:
```bash
curl -X POST -H "Content-Type: application/json" -d '{
"id": "123",
"name": "John",
"message": "Hello world"
}' http://localhost:8080/post
```
以上示例代码和命令仅供参考,具体根据实际情况进行调整。
func qidong() { gin.SetMode(gin.ReleaseMode) gin.DisableConsoleColor() router := gin.Default() //router.Use(log.Logger()) router.GET("/", SlowHandler) // 写一个服务监听 server := &http.Server{ Addr: ":8081", Handler: router, ReadTimeout: 10 * time.Second, WriteTimeout: 10 * time.Second, MaxHeaderBytes: 1 << 20, } // 启动服务 go server.ListenAndServe() // 优雅退出 gracefulExitServer(server) } 如何关闭go qidong协程
你可以使用 `context` 包来关闭 `go qidong()` 协程。以下是示例代码:
```go
package main
import (
"context"
"fmt"
"net/http"
"time"
"github.com/gin-gonic/gin"
)
func qidong(ctx context.Context) error {
gin.SetMode(gin.ReleaseMode)
gin.DisableConsoleColor()
router := gin.Default()
//router.Use(log.Logger())
router.GET("/", SlowHandler)
server := &http.Server{
Addr: ":8081",
Handler: router,
ReadTimeout: 10 * time.Second,
WriteTimeout: 10 * time.Second,
MaxHeaderBytes: 1 << 20,
}
go func() {
if err := server.ListenAndServe(); err != nil && err != http.ErrServerClosed {
fmt.Printf("listen: %s\n", err)
}
}()
<-ctx.Done() // 等待接收关闭信号
ctxShutDown, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer func() {
cancel()
}()
if err := server.Shutdown(ctxShutDown); err != nil {
fmt.Printf("Server Shutdown Failed:%s\n", err)
return err
}
fmt.Println("Server exiting")
return nil
}
func main() {
ctx, cancel := context.WithCancel(context.Background())
defer func() {
cancel()
}()
go qidong(ctx)
// 等待 10 秒钟,然后发送一个关闭信号给协程
time.Sleep(10 * time.Second)
fmt.Println("发送关闭信号")
cancel()
// 等待 1 秒钟,观察协程是否被正常关闭。
time.Sleep(1 * time.Second)
fmt.Println("main 函数退出")
}
func SlowHandler(c *gin.Context) {
time.Sleep(2 * time.Second)
c.JSON(http.StatusOK, gin.H{"message": "slow response"})
}
```
在上面的示例代码中,我们使用 `context` 包创建了一个 `ctx` 对象,并把它传递给 `qidong()` 协程。在协程函数中,我们使用 `select` 语句监听 `ctx.Done()` 通道,一旦收到关闭信号,就会退出协程。
在 `main()` 函数中,我们等待了 10 秒钟,然后发送一个关闭信号给协程。协程函数会在执行过程中不断地检查 `ctx.Done()` 通道,一旦收到关闭信号,就会退出协程。在 `main()` 函数中,我们等待了 1 秒钟,观察协程是否被正常关闭。在协程函数中,我们还使用了 `server.Shutdown()` 方法来关闭 HTTP 服务,以确保服务正常退出。
阅读全文
相关推荐
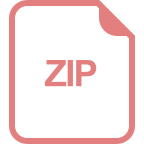
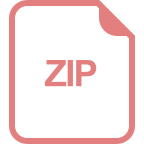
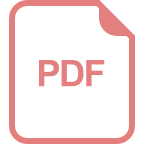














