使用java写一个android项目,一个activity中包含一个PreferenceScreen,PreferenceScreen中有一个SwitchPreference,SwitchPreference使用自定义布局,要求switch在最左侧,title、icon
时间: 2024-01-24 19:16:54 浏览: 170
在XML布局文件中,可以使用PreferenceScreen标签来创建一个PreferenceScreen。然后在PreferenceScreen中添加SwitchPreference,使用android:widgetLayout属性来设置自定义布局。
下面是一个示例代码:
```xml
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android">
<SwitchPreference
android:key="example_switch"
android:title="Example Switch"
android:summary="This is an example switch preference"
android:widgetLayout="@layout/custom_switch_preference"
/>
</PreferenceScreen>
```
在这个示例中,我们创建了一个PreferenceScreen,其中包含一个SwitchPreference。我们使用了android:widgetLayout属性来设置SwitchPreference的自定义布局为@layout/custom_switch_preference。
接下来,在res/layout文件夹下创建一个名为custom_switch_preference.xml的布局文件,用于定义SwitchPreference的自定义布局:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@android:id/icon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_marginStart="16dp"
android:contentDescription="@null"
android:src="@drawable/ic_launcher"/>
<TextView
android:id="@android:id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="72dp"
android:layout_marginTop="16dp"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textColor="?android:attr/textColorPrimary"
android:text="Example Switch"/>
<Switch
android:id="@android:id/switch_widget"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:layout_marginEnd="16dp"/>
</RelativeLayout>
```
在这个布局文件中,我们使用了一个相对布局,其中包含了一个ImageView、一个TextView和一个Switch。我们使用android:id属性来设置它们的id,以便在Java代码中引用它们。
最后,在Java代码中,我们可以使用SharedPreferences类来读取和写入SwitchPreference的值。例如,以下代码演示了如何读取SwitchPreference的值:
```java
SharedPreferences sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this);
boolean isChecked = sharedPreferences.getBoolean("example_switch", false);
```
其中,我们使用getDefaultSharedPreferences()方法获取默认的SharedPreferences实例,并使用getBoolean()方法来获取SwitchPreference的值。第二个参数是默认值,如果SwitchPreference的值不存在,则使用该默认值。
如果要写入SwitchPreference的值,可以使用SharedPreferences.Editor类。例如,以下代码演示了如何将SwitchPreference的值写入SharedPreferences:
```java
SharedPreferences sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putBoolean("example_switch", true);
editor.apply();
```
其中,我们使用edit()方法获取SharedPreferences.Editor实例,并使用putBoolean()方法将SwitchPreference的值写入SharedPreferences。最后,我们使用apply()方法提交更改。
阅读全文
相关推荐
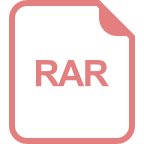
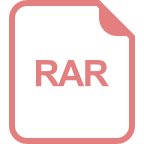
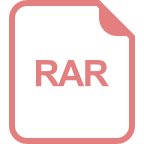














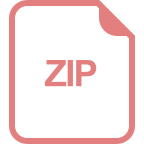
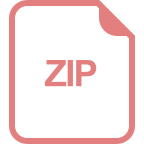