用c语言写学生成绩管理系统,查询时可实现按姓名、按学号查询,输入任意的一个课程名(如数学)和一个分数段(如 60--70),统计出在此分数段的学生情况。C
时间: 2024-03-13 18:44:39 浏览: 115
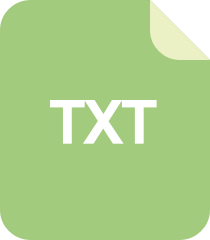
C语言课程设计—学生成绩管理系统
好的,这是一个比较典型的学生成绩管理系统,下面是一个简单的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT 100
typedef struct {
char name[20];
int id;
int math;
int english;
int physics;
} Student;
Student students[MAX_STUDENT];
int num_students = 0;
int search_by_name(char *name) {
int i;
for (i = 0; i < num_students; i++) {
if (strcmp(students[i].name, name) == 0) {
return i;
}
}
return -1;
}
int search_by_id(int id) {
int i;
for (i = 0; i < num_students; i++) {
if (students[i].id == id) {
return i;
}
}
return -1;
}
void add_student() {
if (num_students >= MAX_STUDENT) {
printf("Too many students, cannot add more.\n");
return;
}
Student *s = &students[num_students++];
printf("Please enter student information:\n");
printf("Name: ");
scanf("%s", s->name);
printf("ID: ");
scanf("%d", &s->id);
printf("Math score: ");
scanf("%d", &s->math);
printf("English score: ");
scanf("%d", &s->english);
printf("Physics score: ");
scanf("%d", &s->physics);
}
void print_student(Student *s) {
printf("Name: %s\n", s->name);
printf("ID: %d\n", s->id);
printf("Math score: %d\n", s->math);
printf("English score: %d\n", s->english);
printf("Physics score: %d\n", s->physics);
}
void list_students() {
int i;
for (i = 0; i < num_students; i++) {
printf("Student %d:\n", i+1);
print_student(&students[i]);
}
}
void search_student() {
int option, id, index;
char name[20];
printf("Please select search option:\n");
printf("1. Search by name\n");
printf("2. Search by ID\n");
scanf("%d", &option);
switch (option) {
case 1:
printf("Please enter student name:\n");
scanf("%s", name);
index = search_by_name(name);
break;
case 2:
printf("Please enter student ID:\n");
scanf("%d", &id);
index = search_by_id(id);
break;
default:
printf("Invalid option.\n");
return;
}
if (index == -1) {
printf("Student not found.\n");
} else {
printf("Student found:\n");
print_student(&students[index]);
}
}
void search_by_score() {
char subject[20];
int low, high, i, count = 0;
printf("Please enter subject name:\n");
scanf("%s", subject);
printf("Please enter low score:\n");
scanf("%d", &low);
printf("Please enter high score:\n");
scanf("%d", &high);
printf("Students with %s score between %d and %d:\n", subject, low, high);
for (i = 0; i < num_students; i++) {
int score = 0;
if (strcmp(subject, "math") == 0) {
score = students[i].math;
} else if (strcmp(subject, "english") == 0) {
score = students[i].english;
} else if (strcmp(subject, "physics") == 0) {
score = students[i].physics;
}
if (score >= low && score <= high) {
print_student(&students[i]);
count++;
}
}
if (count == 0) {
printf("No students found with %s score between %d and %d.\n", subject, low, high);
}
}
int main() {
int option;
while (1) {
printf("Please select an option:\n");
printf("1. Add student\n");
printf("2. List all students\n");
printf("3. Search student\n");
printf("4. Search by score\n");
printf("5. Quit\n");
scanf("%d", &option);
switch (option) {
case 1:
add_student();
break;
case 2:
list_students();
break;
case 3:
search_student();
break;
case 4:
search_by_score();
break;
case 5:
return 0;
default:
printf("Invalid option.\n");
break;
}
}
}
```
这个程序中,我们使用了一个 `Student` 结构体来表示学生,其中包含了姓名、学号、数学、英语、物理三门科目的成绩。我们使用一个数组 `students` 来存储所有学生的信息,还有一个整数变量 `num_students` 表示当前学生数量。
程序的主要功能包括:
- 添加学生信息,使用 `add_student` 函数实现;
- 列出所有学生信息,使用 `list_students` 函数实现;
- 按姓名或学号查询学生信息,使用 `search_student` 函数实现;
- 按科目和分数段查询学生信息,使用 `search_by_score` 函数实现。
在查询学生信息时,我们使用了两个简单的查找函数 `search_by_name` 和 `search_by_id`,它们分别按姓名和学号进行查找,并返回找到的学生在数组中的索引。在查询科目和分数段时,我们使用了一个循环遍历所有学生,对每个学生的指定科目成绩进行比较,符合条件的就输出。
注意到这个程序还有一些简单的输入输出和错误处理,这些内容可以根据实际需要进行修改和完善。
阅读全文
相关推荐
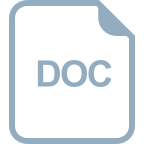
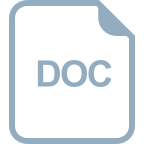
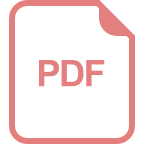
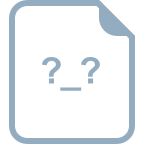
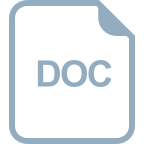
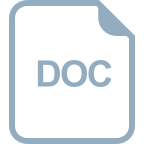
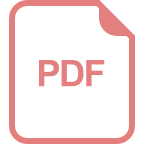
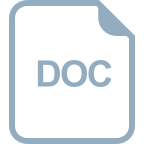
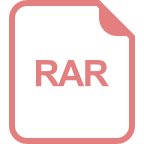
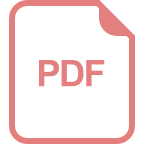
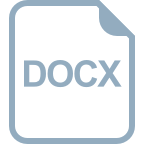
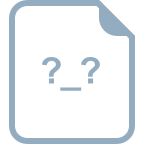
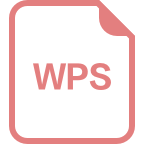
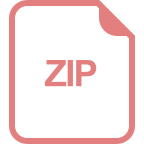
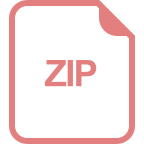

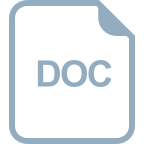
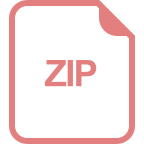