PSO优化算法优化LSTM神经网络参数:units、batch size、learning rate,其中LSTM的输出为2维的,请提供使用pyswarms库代码示例
时间: 2024-03-26 14:37:41 浏览: 85
好的,以下是使用pyswarms库进行PSO优化算法优化LSTM神经网络参数的示例代码:
``` python
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, LSTM
from keras.callbacks import EarlyStopping
from pyswarms.single.global_best import GlobalBestPSO
# Define the LSTM model
def create_lstm_model(units, batch_size, learning_rate):
model = Sequential()
model.add(LSTM(units, input_shape=(1, 1)))
model.add(Dense(2, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
return model
# Define the fitness function
def fitness_function(params):
units = params[0]
batch_size = params[1]
learning_rate = params[2]
# Create the LSTM model
lstm_model = create_lstm_model(units, batch_size, learning_rate)
# Fit the model on training data
lstm_model.fit(X_train, y_train, epochs=10, batch_size=batch_size, verbose=0, callbacks=[early_stopping])
# Evaluate the model on validation data
loss, accuracy = lstm_model.evaluate(X_val, y_val, verbose=0)
return -accuracy
# Load the data
X_train = np.random.rand(100, 1, 1)
y_train = np.random.randint(2, size=(100, 2))
X_val = np.random.rand(50, 1, 1)
y_val = np.random.randint(2, size=(50, 2))
# Define the hyperparameters bounds
bounds = [(16, 256), (16, 128), (0.001, 0.1)]
# Define the early stopping callback
early_stopping = EarlyStopping(monitor='val_loss', patience=3)
# Create the optimizer
optimizer = GlobalBestPSO(n_particles=10, dimensions=3, options={'c1': 0.5, 'c2': 0.3, 'w':0.9})
# Perform the optimization
best_params, best_fitness = optimizer.optimize(fitness_function, iters=100)
# Print the results
print('Best parameters:', best_params)
print('Best fitness:', -best_fitness)
```
在上面的代码中,我们首先定义了一个 `create_lstm_model()` 函数,用于创建具有给定参数的 LSTM 模型。然后定义了一个适应度函数 `fitness_function()`,用于评估给定参数的模型在验证数据上的性能。该函数将使用给定参数创建 LSTM 模型,然后在训练数据上拟合模型,并在验证数据上评估模型性能。最终将返回模型的验证精度的相反数(因为PSO算法是最小化问题)。
然后我们加载数据并定义超参数的范围。接着我们定义了一个 `GlobalBestPSO` 类型的优化器,并使用 `optimize()` 方法执行优化。在优化过程中,优化器将多次调用适应度函数,并寻找产生最小适应度值的参数组合。最终,我们输出找到的最佳参数和最佳适应度值。
注意:这里的数据是随机生成的,实际应用中需要使用真实数据进行优化。
阅读全文
相关推荐
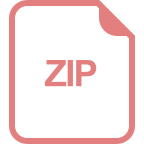
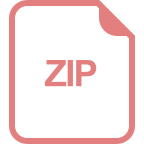




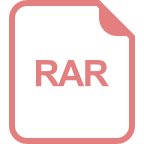
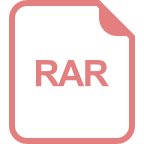
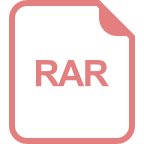
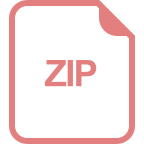
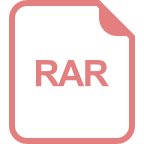
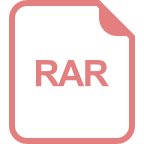
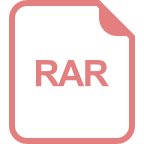
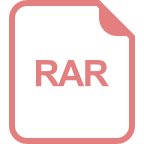
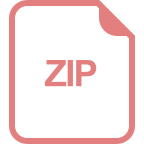
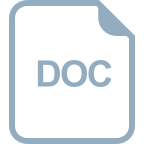