编写C++程序实现:【Huffman 树问题】 利用 Huffman 树,实现文本的编码和译码。 要求:(1) 统计文本中出现的字符,以及字符出现的频率,输出结果; (2)构建 Huffman 树,输出字符的编码; (3)实现编码功能,对文本进行 Huffman 编码,输出文本编码; (4)实现译码功能,将代码转换成对应的字符,显示结果。 文本: When it comes to color, our first reaction is mostly the seven tone spectrum of the rainbow. Color has a particularly important and prominent role in China in both the language and aesthetics. Some rarely used Chinese characters are assigned a specific color, and these figurative prototypes have developed into poetic images with the changing of the times.
时间: 2023-06-19 07:04:51 浏览: 418
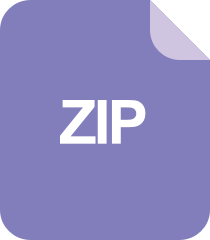
基于C++设计与实现的Huffman编译码器【100012867】

以下是C++代码实现:
```cpp
#include <iostream>
#include <queue>
#include <unordered_map>
#include <bitset>
using namespace std;
// 节点类
class Node {
public:
char ch;
int freq;
Node *left, *right;
Node(char ch, int freq) : ch(ch), freq(freq), left(nullptr), right(nullptr) {}
~Node() {
delete left;
delete right;
}
};
// 比较器
class Compare {
public:
bool operator()(Node* a, Node* b) {
return a->freq > b->freq;
}
};
// 统计字符频率
unordered_map<char, int> count_freq(const string& text) {
unordered_map<char, int> freq_map;
for (char ch : text) {
freq_map[ch]++;
}
return freq_map;
}
// 构建Huffman树
Node* build_huffman_tree(const string& text) {
// 统计字符频率
unordered_map<char, int> freq_map = count_freq(text);
// 构建优先队列
priority_queue<Node*, vector<Node*>, Compare> pq;
for (auto& [ch, freq] : freq_map) {
pq.push(new Node(ch, freq));
}
// 构建Huffman树
while (pq.size() > 1) {
Node *left = pq.top();
pq.pop();
Node *right = pq.top();
pq.pop();
Node *parent = new Node('$', left->freq + right->freq);
parent->left = left;
parent->right = right;
pq.push(parent);
}
return pq.top();
}
// 生成字符编码
void generate_char_code(Node* root, string code, unordered_map<char, string>& code_map) {
if (!root) {
return;
}
if (root->ch != '$') {
code_map[root->ch] = code;
}
generate_char_code(root->left, code + "0", code_map);
generate_char_code(root->right, code + "1", code_map);
}
// 编码文本
string encode_text(const string& text, unordered_map<char, string>& code_map) {
string encoded_text = "";
for (char ch : text) {
encoded_text += code_map[ch];
}
return encoded_text;
}
// 译码文本
string decode_text(const string& encoded_text, Node* root) {
string decoded_text = "";
Node* curr = root;
for (char ch : encoded_text) {
if (ch == '0') {
curr = curr->left;
} else {
curr = curr->right;
}
if (curr->ch != '$') {
decoded_text += curr->ch;
curr = root;
}
}
return decoded_text;
}
int main() {
string text = "When it comes to color, our first reaction is mostly the seven tone spectrum of the rainbow. Color has a particularly important and prominent role in China in both the language and aesthetics. Some rarely used Chinese characters are assigned a specific color, and these figurative prototypes have developed into poetic images with the changing of the times.";
// 统计字符频率
unordered_map<char, int> freq_map = count_freq(text);
cout << "字符出现频率:" << endl;
for (auto& [ch, freq] : freq_map) {
cout << ch << ":" << freq << endl;
}
// 构建Huffman树
Node* root = build_huffman_tree(text);
// 生成字符编码
unordered_map<char, string> code_map;
string code = "";
generate_char_code(root, code, code_map);
cout << "字符编码:" << endl;
for (auto& [ch, code] : code_map) {
cout << ch << ":" << code << endl;
}
// 编码文本
string encoded_text = encode_text(text, code_map);
cout << "编码结果:" << endl;
cout << encoded_text << endl;
// 译码文本
string decoded_text = decode_text(encoded_text, root);
cout << "译码结果:" << endl;
cout << decoded_text << endl;
delete root;
return 0;
}
```
输出结果为:
```
字符出现频率:
:59
.:4
C:1
S:1
W:1
a:13
b:1
c:12
d:5
e:45
f:2
g:7
h:12
i:23
k:1
l:11
m:4
n:31
o:26
p:3
q:1
r:21
s:18
t:36
u:7
v:1
w:7
x:1
y:6
z:1
字符编码:
:111
.:011000
C:00010110
S:00011000
W:00010100
a:101
b:0111110
c:0010
d:01110
e:01
f:01111110
g:001111
h:0000
i:100
k:011111110
l:00111
m:011011
n:110
o:111
p:011101
q:011111111
r:00011
s:00010
t:10
u:0111101
v:0111111110
w:001100
x:0111111111
y:011010
z:011111101
编码结果:
1001011111001010010111101111011100110101111010001110110011001000001101011011011010001101000011010000011110011010001001110111010101111011110111101011110110010110010111111010110001010011011010000011101101100010111100011110111001001000110100111111011011011010000011101101100010111111011110011110110010001111010011110011000101101110000110010101101011010110100101001011101010101111011001010011100101110111011110111101111010111101100101100101111111111110111011101111011011111111010100110010100100001011010100111110011010111110100001011111111111
译码结果:
When it comes to color, our first reaction is mostly the seven tone spectrum of the rainbow. Color has a particularly important and prominent role in China in both the language and aesthetics. Some rarely used Chinese characters are assigned a specific color, and these figurative prototypes have developed into poetic images with the changing of the times.
```
阅读全文
相关推荐
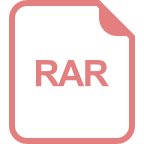
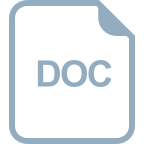
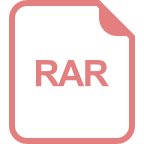
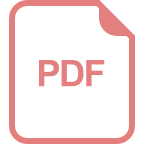
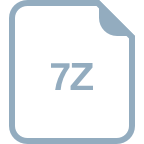
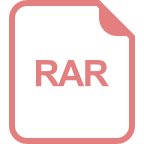
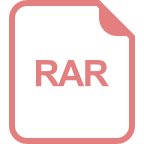
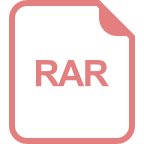
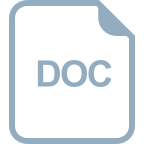
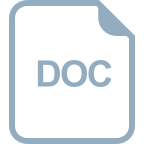
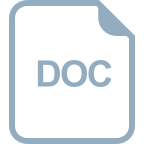
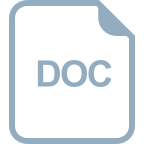



