qt中如何编写一个具备调节大小,平移,旋转的矩形类
时间: 2023-05-19 20:02:25 浏览: 209
在Qt中,可以使用QGraphicsItem类来实现具备调节大小、平移、旋转的矩形类。具体实现步骤如下:
1. 创建一个自定义的矩形类,继承自QGraphicsItem类。
2. 在该类中重写boundingRect()函数,返回矩形的边界矩形。
3. 在该类中重写paint()函数,实现矩形的绘制。
4. 在该类中重写mousePressEvent()、mouseMoveEvent()、mouseReleaseEvent()函数,实现矩形的平移、调节大小、旋转等功能。
5. 在主窗口中创建一个QGraphicsScene对象,并将自定义的矩形类添加到该场景中。
6. 在主窗口中创建一个QGraphicsView对象,并将场景设置为该视图的场景。
7. 运行程序,即可看到具备调节大小、平移、旋转功能的矩形类。
下面是一个简单的示例代码:
```
#include <QGraphicsItem>
#include <QPainter>
#include <QPointF>
#include <QRectF>
#include <QGraphicsSceneMouseEvent>
class MyRectItem : public QGraphicsItem
{
public:
MyRectItem(QGraphicsItem *parent = nullptr);
QRectF boundingRect() const override;
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override;
protected:
void mousePressEvent(QGraphicsSceneMouseEvent *event) override;
void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override;
void mouseReleaseEvent(QGraphicsSceneMouseEvent *event) override;
private:
QPointF m_lastPos;
QRectF m_rect;
};
MyRectItem::MyRectItem(QGraphicsItem *parent)
: QGraphicsItem(parent)
{
m_rect = QRectF(-50, -50, 100, 100);
}
QRectF MyRectItem::boundingRect() const
{
return m_rect;
}
void MyRectItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
painter->drawRect(m_rect);
}
void MyRectItem::mousePressEvent(QGraphicsSceneMouseEvent *event)
{
m_lastPos = event->pos();
}
void MyRectItem::mouseMoveEvent(QGraphicsSceneMouseEvent *event)
{
QPointF delta = event->pos() - m_lastPos;
m_rect.translate(delta);
m_lastPos = event->pos();
update();
}
void MyRectItem::mouseReleaseEvent(QGraphicsSceneMouseEvent *event)
{
Q_UNUSED(event);
}
// 在主窗口中添加如下代码:
QGraphicsScene *scene = new QGraphicsScene(this);
MyRectItem *rectItem = new MyRectItem();
scene->addItem(rectItem);
QGraphicsView *view = new QGraphicsView(this);
view->setScene(scene);
```
这样就可以在Qt中实现具备调节大小、平移、旋转功能的矩形类了。
阅读全文
相关推荐


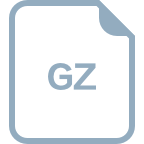









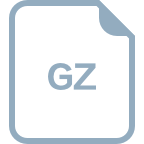



