做一个俄罗斯方块的Java,代码尽量简略
时间: 2024-02-09 21:09:44 浏览: 134
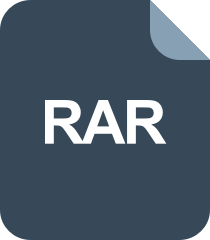
基于java的俄罗斯方块实现代码和作业报告
好的,以下是一个简略的俄罗斯方块Java代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Tetris extends JPanel {
private static final long serialVersionUID = 1L;
private final int WIDTH = 10;
private final int HEIGHT = 22;
private final int BLOCK_SIZE = 30;
private int[][] board = new int[WIDTH][HEIGHT];
private Shape[] shapes = new Shape[7];
private Shape currentShape;
private Random random = new Random();
private int score = 0;
public Tetris() {
shapes[0] = new Shape(new int[][] { { 1, 1, 1, 1 } }, this, Color.cyan);
shapes[1] = new Shape(new int[][] { { 1, 1, 0 }, { 0, 1, 1 } }, this, Color.orange);
shapes[2] = new Shape(new int[][] { { 0, 1, 1 }, { 1, 1, 0 } }, this, Color.blue);
shapes[3] = new Shape(new int[][] { { 1, 1, 0 }, { 1, 1, 0 } }, this, Color.yellow);
shapes[4] = new Shape(new int[][] { { 1, 0, 0 }, { 1, 1, 1 } }, this, Color.green);
shapes[5] = new Shape(new int[][] { { 0, 0, 1 }, { 1, 1, 1 } }, this, Color.pink);
shapes[6] = new Shape(new int[][] { { 0, 1, 0 }, { 1, 1, 1 } }, this, Color.red);
newPiece();
}
public void newPiece() {
currentShape = shapes[random.nextInt(7)];
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
currentShape.drawShape(g);
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
if (board[i][j] != 0) {
g.setColor(Color.gray);
g.fillRect(i * BLOCK_SIZE, j * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
}
public void removeRow(int row) {
for (int j = row; j > 0; j--) {
for (int i = 0; i < WIDTH; i++) {
board[i][j] = board[i][j - 1];
}
}
score += 100;
}
public boolean isValidMove(Shape shape, int x, int y) {
int[][] coords = shape.getCoords();
for (int i = 0; i < coords.length; i++) {
int newX = coords[i][0] + x;
int newY = coords[i][1] + y;
if (newX < 0 || newX >= WIDTH || newY < 0 || newY >= HEIGHT) {
return false;
}
if (board[newX][newY] != 0) {
return false;
}
}
return true;
}
public void placePiece(Shape shape) {
int[][] coords = shape.getCoords();
for (int i = 0; i < coords.length; i++) {
int x = coords[i][0];
int y = coords[i][1];
board[x][y] = shape.getColor();
}
}
public void clearBoard() {
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
board[i][j] = 0;
}
}
}
public void run() {
while (true) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
if (isValidMove(currentShape, currentShape.getX(), currentShape.getY() + 1)) {
currentShape.moveDown();
} else {
placePiece(currentShape);
int clearedRows = 0;
for (int j = 0; j < HEIGHT; j++) {
boolean rowIsFull = true;
for (int i = 0; i < WIDTH; i++) {
if (board[i][j] == 0) {
rowIsFull = false;
break;
}
}
if (rowIsFull) {
removeRow(j);
clearedRows++;
}
}
newPiece();
if (!isValidMove(currentShape, currentShape.getX(), currentShape.getY())) {
clearBoard();
score = 0;
}
}
repaint();
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(320, 640);
frame.getContentPane().add(new Tetris());
frame.setVisible(true);
}
}
class Shape {
private int[][] coords;
private Tetris tetris;
private Color color;
private int x, y;
public Shape(int[][] coords, Tetris tetris, Color color) {
this.coords = coords;
this.tetris = tetris;
this.color = color;
x = tetris.WIDTH / 2;
y = 0;
}
public int[][] getCoords() {
return coords;
}
public int getColor() {
return color.getRGB();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public void moveDown() {
y++;
}
public void moveLeft() {
if (tetris.isValidMove(this, x - 1, y)) {
x--;
}
}
public void moveRight() {
if (tetris.isValidMove(this, x + 1, y)) {
x++;
}
}
public void rotate() {
int[][] rotatedCoords = new int[coords[0].length][coords.length];
for (int i = 0; i < coords.length; i++) {
for (int j = 0; j < coords[0].length; j++) {
rotatedCoords[j][coords.length - 1 - i] = coords[i][j];
}
}
if (tetris.isValidMove(this, x, y, rotatedCoords)) {
coords = rotatedCoords;
}
}
public void drawShape(Graphics g) {
g.setColor(color);
for (int i = 0; i < coords.length; i++) {
int x = (coords[i][0] + this.x) * tetris.BLOCK_SIZE;
int y = (coords[i][1] + this.y) * tetris.BLOCK_SIZE;
g.fillRect(x, y, tetris.BLOCK_SIZE, tetris.BLOCK_SIZE);
}
}
}
```
这是一个比较基础的俄罗斯方块代码,实现了基本的方块下落、移动、旋转、消行等功能。如果想要更完整的代码实现,可以在此基础上进行修改和拓展。
阅读全文
相关推荐
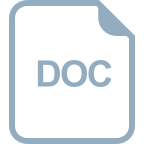
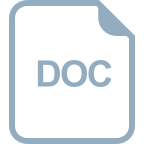
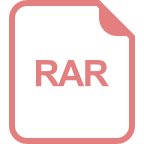
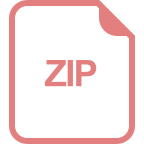
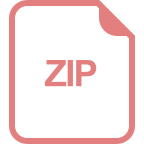
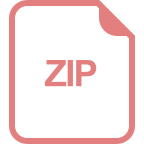
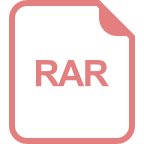
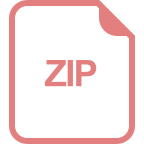
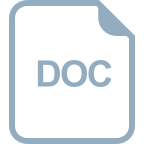
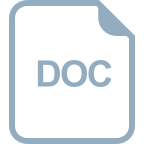
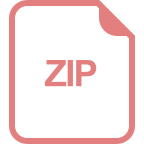
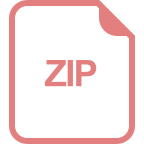
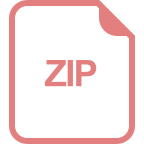
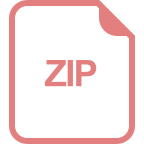