for num in range(100,1000): r=map(lambda x:int(x)**3,str(num))
时间: 2024-05-29 17:12:38 浏览: 137
if sum(r)==num: print(num)
This code finds all the Armstrong numbers between 100 and 999. An Armstrong number is a number that is equal to the sum of its own digits raised to the power of the number of digits. For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
The code uses a for loop to iterate through all the numbers between 100 and 999. It then converts each number to a string and applies a lambda function to each digit to raise it to the power of 3. The resulting list of cubed digits is then summed up and checked against the original number. If they are equal, the number is printed out as an Armstrong number.
相关问题
for num in range(100, 1000): if sum(map(lambda x:int(x)**3, str(num))) == num: print(num) 详细解释这段代码
这段代码是用来找出三位数中的所有阿姆斯特朗数的。阿姆斯特朗数(又称水仙花数)是指一个 n 位正整数(n≥3)的每个位上的数字的 n 次幂之和等于它本身。例如,153 是一个阿姆斯特朗数,因为 1^3 + 5^3 + 3^3 = 153。
现在来详细解释这段代码:
```python
for num in range(100, 1000): # 遍历所有三位数
if sum(map(lambda x:int(x)**3, str(num))) == num: # 判断是否为阿姆斯特朗数
print(num) # 输出阿姆斯特朗数
```
代码中的 `range(100, 1000)` 表示遍历所有从 100 到 999 之间的整数,即所有的三位数。遍历过程中,对于每一个数 `num`,都会执行 `if` 语句中的判断。
`map(lambda x:int(x)**3, str(num))` 这一部分是将 `num` 转换为字符串后,对每个字符执行 `int(x)**3` 操作,然后将结果组成一个列表。这样可以得到所有位数的立方和。例如,对于数字 153,`map` 函数会生成一个列表 `[1**3, 5**3, 3**3]`。
接着,`sum` 函数对上面生成的列表求和,得到这个数的立方和。如果这个和等于 `num`,那么这个数就是阿姆斯特朗数,满足条件,就会被输出。
#第二次作业 #26 #(1) lst=[1,2,3,4,5] square=map(lambda x:x*x,lst) print(list(square)) #(2) even=filter(lambda x:x%2==0,lst) print(list(even)) #27 #(1) file1=open("E:/大一/python与程序设计/file1.txt","r") content1=file1.read() lst1=content1.split() num=list(map(int,lst1)) allnum=sum(num) print(allnum) file1.close() #(2) file1=open("E:/大一/python与程序设计/file1.txt","r") content=[] for i in range(1,4): l=file1.readline() num= list(map(int, l.split())) num.sort() strs=" ".join(list(map(str,num))) strs2=strs+"\n" content.append(strs2) file2=open("E:/大一/python与程序设计/file2.txt","w") file2.writelines(content) file2.close() file1.close() #(3) file1=open("E:/大一/python与程序设计/file1.txt","r") content=file1.readlines() print(len(content)) #28 from datetime import datetime as dt file3=open("E:/大一/python与程序设计/file3.txt",'r',encoding='utf-8') line1=file3.readline() content=[] for i in range(1,4): l=file3.readline().split() content.append(l) col1=[content[0][0],content[1][0],content[2][0]] col2=[content[0][1],content[1][1],content[2][1]] col3=[content[0][2],content[1][2],content[2][2]] col4=[content[0][3],content[1][3],content[2][3]] day_formate="%H:%M:%S" Time=[] Code=[] Price=[] Volume=[] for t in col1: Time.append(dt.strptime(t,day_formate)) for c in col2: Code.append(str(c)) for p in col3: Price.append(float(p)) for v in col4: Volume.append(int(v)) file3.close() #29 #(1) mean=lambda x,y,z:(x+y+z)/3 #(2) def mean(*num): if bool(num)==0: return None else: return sum(num)/len(num) #30 def fibo(n): if n==1 or n==2: return 1 else: return fibo(n-1)+fibo(n-2) #31 from math import sqrt class Point(): def __init__(self,x,y): self.x=x self.y=y class Line(Point): def __init__(self,p1,p2): self.p1=p1 self.p2=p2 def lenth(self): lenth=sqrt((self.p1.x-self.p2.x)**2+(self.p1.y-self.p2.y)**2) return lenth def slope(self): if self.p1.x==self.p2.x: return None else: k=(self.p1.y-self.p2.y)/(self.p1.x-self.p2.x) return k def __repr__(self): return ((self.p1),(self.p2)) p1=Point(2,3) p2=Point(5,9) line=Line(p1,p2) l_line=line.lenth() k_line=line.slope() print(f"起点(2,3)到止点(5,9)的线段长度为{l_line},斜率为{k_line}") #32 class Point(): #(1) def __init__(self,x=0,y=0): self.x=x self.y=y #(2) def trans(self): return (self.y,self.x) #(3) def show(self): return print(f"该点坐标为({self.x},{self.y})") #(4) p1=Point(1,2) p1.trans() p1.show() p2=Point(3,5) p2.trans() p2.show()
对于你的代码,我进行了一些修改和注释,以便更好地理解。请查看以下代码:
# 26
# (1) 计算列表中每个元素的平方
lst = [1, 2, 3, 4, 5]
square = map(lambda x: x * x, lst)
print(list(square))
# (2) 过滤出列表中的偶数
even = filter(lambda x: x % 2 == 0, lst)
print(list(even))
# 27
# (1) 读取文件中的数字,并计算它们的总和
file1 = open("E:/大一/python与程序设计/file1.txt", "r")
content1 = file1.read()
lst1 = content1.split()
num = list(map(int, lst1))
allnum = sum(num)
print(allnum)
file1.close()
# (2) 读取文件中的数字,并将每行数字进行排序后写入新文件
file1 = open("E:/大一/python与程序设计/file1.txt", "r")
content = []
for i in range(1, 4):
l = file1.readline()
num = list(map(int, l.split()))
num.sort()
strs = " ".join(list(map(str, num)))
strs2 = strs + "\n"
content.append(strs2)
file2 = open("E:/大一/python与程序设计/file2.txt", "w")
file2.writelines(content)
file2.close()
file1.close()
# (3) 读取文件中的行数
file1 = open("E:/大一/python与程序设计/file1.txt", "r")
content = file1.readlines()
print(len(content))
# 28
# 从文件中读取数据,并按照列进行解析
from datetime import datetime as dt
file3 = open("E:/大一/python与程序设计/file3.txt", 'r', encoding='utf-8')
line1 = file3.readline()
content = []
for i in range(1, 4):
l = file3.readline().split()
content.append(l)
# 将字符串时间转换为datetime类型
col1 = [content[0][0], content[1][0], content[2][0]]
day_formate = "%H:%M:%S"
Time = [dt.strptime(t, day_formate) for t in col1]
# 将字符串转换为其他类型
col2 = [content[0][1], content[1][1], content[2][1]]
Code = [str(c) for c in col2]
col3 = [content[0][2], content[1][2], content[2][2]]
Price = [float(p) for p in col3]
col4 = [content[0][3], content[1][3], content[2][3]]
Volume = [int(v) for v in col4]
file3.close()
# 29
# (1) 求三个数的平均值
mean = lambda x, y, z: (x + y + z) / 3
# (2) 求任意数量的数的平均值
def mean(*num):
if bool(num) == 0:
return None
else:
return sum(num) / len(num)
# 30
# 计算斐波那契数列的第n项
def fibo(n):
if n == 1 or n == 2:
return 1
else:
return fibo(n - 1) + fibo(n - 2)
# 31
# 定义Point和Line类,并计算线段长度和斜率
from math import sqrt
class Point():
def __init__(self, x, y):
self.x = x
self.y = y
class Line(Point):
def __init__(self, p1, p2):
self.p1 = p1
self.p2 = p2
def length(self):
length = sqrt((self.p1.x - self.p2.x) ** 2 + (self.p1.y - self.p2.y) ** 2)
return length
def slope(self):
if self.p1.x == self.p2.x:
return None
else:
k = (self.p1.y - self.p2.y) / (self.p1.x - self.p2.x)
return k
def __repr__(self):
return ((self.p1), (self.p2))
p1 = Point(2, 3)
p2 = Point(5, 9)
line = Line(p1, p2)
l_length = line.length()
k_line = line.slope()
print(f"起点(2,3)到止点(5,9)的线段长度为{l_length},斜率为{k_line}")
# 32
# (1) 定义一个Point类,包含x和y坐标
class Point():
def __init__(self, x=0, y=0):
self.x = x
self.y = y
# (2) 定义一个trans()函数,将坐标轴进行转换
def trans(self):
return (self.y, self.x)
# (3) 定义一个show()函数,打印出点的坐标
def show(self):
return print(f"该点坐标为({self.x},{self.y})")
p1 = Point(1, 2)
p1.trans()
p1.show()
p2 = Point(3, 5)
p2.trans()
p2.show()
阅读全文
相关推荐
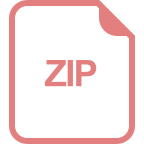
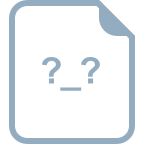
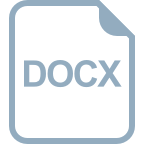





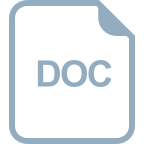
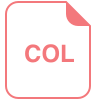





