I)编写求两个整数最大公约数的函数 getGCM(int numberl, int numbe2)和求最小公倍数的函数 getLCM(int numberl, int number2),并在主函数中验证程序的正确性。
时间: 2024-12-24 14:41:15 浏览: 2
在编程中,你可以通过欧几里得算法(也称辗转相除法)来实现求两个整数的最大公约数 (GCD) 和最小公倍数 (LCM)。以下是用 C++ 编写的示例:
```cpp
#include <iostream>
using namespace std;
// 函数用于计算两个数的最大公约数
int gcd(int a, int b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
// 根据最大公约数计算最小公倍数
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
// 主函数测试 getGCM 和 getLCM 的功能
int main() {
int number1 = 48, number2 = 18;
// 输出最大公约数
cout << "The GCD of " << number1 << " and " << number2 << " is: " << getGCM(number1, number2) << endl;
// 输出最小公倍数
cout << "The LCM of " << number1 << " and " << number2 << " is: " << getLCM(number1, number2) << endl;
// 验证 GCD 和 LCM 的结果是否满足性质: |number1| * |number2| = GCD * LCM
if (abs(number1 * number2) == abs(getGCM(number1, number2) * getLCM(number1, number2)))
cout << "The validation for GCD and LCM is correct." << endl;
else
cout << "Validation failed. GCD and LCM do not satisfy the property." << endl;
return 0;
}
```
在这个例子中,`getGCM` 函数调用了 `gcd` 函数来计算两数的最大公约数,而 `getLCM` 则利用了 GCD 计算最小公倍数。在 `main` 函数中,我们首先计算并打印出两个数的 GCD 和 LCM,然后验证它们是否满足关系 `|number1| * |number2| = GCD * LCM`。
阅读全文
相关推荐
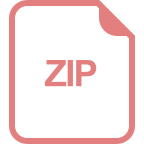

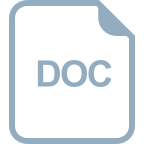



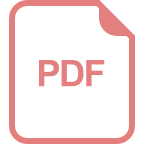
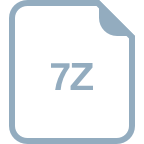
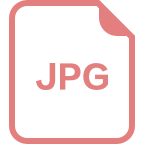
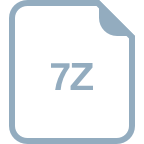
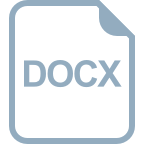