//DivideByZeroException.java public class DivideByZeroException extends ArithmeticException ( public DivideByZeroException()l super("Attempted to divide by zero"); //DivideByZeroExceptionTester.java public class DivideByZeroExceptionTester ( private static int quotient(int numerator, int denominator) .throws DivideByZeroException ( if (denominator == 0) throw new DivideByZeroException();return(numerator / denominator); public static yoid main(String args[]) int numberl=0, number2=0, result=0; tryl System.out.println("Enter the first number:"); numberl = Integer.valueof(Keyboard.getString()).intValue(): System.out.println("Enter the second number:"); number2= Integer.valueof(Keyboard.getString()).intvalue(); result = quotient (number1, number2); catch (NumberFormatException e) System.out.println("Invalid integer entered!")i System.exit(-1); catch (DivideByZeroException e) System.out.println(e.toString()): System.exit(-1); System.out.println(numberl + "/ "+ number2 + "="+ result);解释一下
时间: 2024-01-29 08:03:53 浏览: 46
这段代码是一个简单的Java程序,用于演示如何处理除以零的异常情况。当用户输入两个整数作为被除数和除数时,程序会尝试计算它们的商。如果除数为0,将抛出自定义的DivideByZeroException异常,提示用户不能除以0。
DivideByZeroException类继承了Java内置的ArithmeticException类,用于表示算术异常。在构造函数中,它调用了父类的构造函数,并传递了一个字符串参数,用于描述异常情况。
DivideByZeroExceptionTester类包含了一个静态方法quotient,用于计算两个整数的商。如果除数为0,它将抛出DivideByZeroException异常。在main方法中,程序通过调用quotient方法来计算两个整数的商,并在捕获异常后输出相应的提示信息。如果用户输入的不是整数,也会抛出NumberFormatException异常。
总的来说,这段代码用于演示如何使用自定义异常来处理程序中的特定异常情况。
相关问题
class DivideByZeroException extends Exception { public DivideByZeroException() { super("除数不能为0!"); } }为什么super写在带参的构造方法里就会报错
在 Java 中,如果一个类继承了另一个类,它必须调用父类的构造方法来初始化父类的属性。在使用 `super()` 时,如果没有传递参数,则默认调用父类的无参构造方法。如果你在带参的构造方法中使用 `super()`,就相当于你要调用父类的无参构造方法,并且没有对应的无参构造方法,编译器就会报错。如果你想调用父类的带参构造方法,就需要使用 `super(参数)` 的方式来调用。
给这段代码增加详细注释import java.util.Scanner;// 自定义除数为0的异常类class DivideByZeroException extends Exception { public DivideByZeroException(String message) { super(message); }}public class CustomExceptionDemo { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); try { System.out.print("请输入被除数:"); double dividend = Double.parseDouble(scanner.nextLine()); System.out.print("请输入除数:"); double divisor = Double.parseDouble(scanner.nextLine()); if (divisor == 0) { throw new DivideByZeroException("除数不能为0"); } System.out.println("结果为:" + (dividend / divisor)); } catch (NumberFormatException e) { System.out.println("数字格式异常:" + e.getMessage()); } catch (DivideByZeroException e) { System.out.println("自定义异常:" + e.getMessage()); } catch (Exception e) { System.out.println("通用型异常:" + e.getMessage()); } }}
// 导入 Scanner 类用于读取用户输入
import java.util.Scanner;
// 自定义除数为0的异常类
class DivideByZeroException extends Exception {
public DivideByZeroException(String message) {
super(message);
}
}
public class CustomExceptionDemo {
public static void main(String[] args) {
// 创建一个 Scanner 对象,用于读取用户输入
Scanner scanner = new Scanner(System.in);
try {
System.out.print("请输入被除数:");
// 读取用户输入的被除数
double dividend = Double.parseDouble(scanner.nextLine());
System.out.print("请输入除数:");
// 读取用户输入的除数
double divisor = Double.parseDouble(scanner.nextLine());
// 如果除数为0,则抛出自定义异常
if (divisor == 0) {
throw new DivideByZeroException("除数不能为0");
}
// 计算结果并输出
System.out.println("结果为:" + (dividend / divisor));
} catch (NumberFormatException e) {
// 如果用户输入的数字格式不正确,则捕获该异常并提示用户
System.out.println("数字格式异常:" + e.getMessage());
} catch (DivideByZeroException e) {
// 如果除数为0,则捕获自定义异常并提示用户
System.out.println("自定义异常:" + e.getMessage());
} catch (Exception e) {
// 捕获其他未知异常并提示用户
System.out.println("通用型异常:" + e.getMessage());
}
}
}
阅读全文
相关推荐




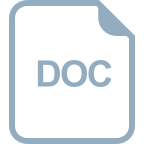









