Mat dstMat = new Mat(); Imgproc.cvtColor(dst,dstMat,Imgproc.COLOR_BGRA2GRAY); Imgproc.threshold(dstMat,dstMat,127,255,Imgproc.THRESH_BINARY); Vector<Vector<Point>> contours2; findContours(dst, contours2, RETR_TREE, CHAIN_APPROX_SIMPLE, new Point(0, 0)); for (int i = 0; i < contours2.size(); i++) { Rect rect2 = Imgproc.boundingRect(contours2.get(i)); if (rect2.width*5 < rect2.height || rect2.width>rect2.height*5) { count ++; System.out.println(count); } }
时间: 2024-02-15 10:27:59 浏览: 74
这段代码主要实现了对二值化图像的轮廓检测,以及对符合条件的矩形区域计数并输出计数结果。如果您需要修改代码,可以根据具体需求进行修改,例如:
1. 修改二值化的阈值,可以通过修改 `threshold` 函数中的参数来实现。例如,将阈值从 127 修改为 100:`Imgproc.threshold(dstMat,dstMat,100,255,Imgproc.THRESH_BINARY)`。
2. 修改轮廓检测的方式,可以通过修改 `findContours` 函数的参数来实现。例如,将检测方式从 `RETR_TREE` 修改为 `RETR_EXTERNAL`:`findContours(dst, contours2, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE, new Point(0, 0))`。
3. 修改判断矩形是否符合条件的方式,可以根据具体需求修改 `if` 语句中的判断条件。例如,将条件修改为矩形宽度小于高度的一半:`if (rect2.width < rect2.height/2)`。
希望以上信息能够对您有所帮助。
相关问题
代码解释:public static String charsSegment(Mat inMat, PlateColor color, Boolean debug, String tempPath) { int charCount = 7; // 车牌字符个数 if (color.equals(PlateColor.GREEN)) { charCount = 8; } // 切换到灰度图 Mat gray = new Mat(); Imgproc.cvtColor(inMat, gray, Imgproc.COLOR_BGR2GRAY); ImageUtil.gaussianBlur(gray, gray, debug, tempPath); Imgcodecs.imwrite(tempPath + Constant.TEMP_CHAR_PLATE_PREDICT, gray); // 图像进行二值化 Mat threshold = new Mat(); switch (color) { case BLUE: Imgproc.threshold(gray, threshold, 10, 255, Imgproc.THRESH_OTSU + Imgproc.THRESH_BINARY); break; default: // GREEN YELLOW Imgproc.threshold(gray, threshold, 10, 255, Imgproc.THRESH_OTSU + Imgproc.THRESH_BINARY_INV); break; } ImageUtil.debugImg(debug, tempPath, "plateThreshold", threshold); // 输出二值图 Imgcodecs.imwrite(tempPath + Constant.TEMP_CHAR_PLATE_PREDICT, threshold); // 边缘腐蚀 threshold = ImageUtil.erode(threshold, debug, tempPath, 2, 2); Imgcodecs.imwrite(tempPath + Constant.TEMP_CHAR_ERODE, threshold); // 垂直方向投影,错切校正 // 理论上,还可以用于分割字符 Integer px = getShearPx(threshold); ImageUtil.shearCorrection(threshold, threshold, px, debug, tempPath); // 前面已经结果错切校正了,可以按照垂直、水平方向投影进行精确定位 // 垂直投影 + 垂直分割线,分割字符 // 水平投影,去掉上下边框、铆钉干扰 threshold = sepAndClear(threshold, px, charCount, debug, tempPath); Imgcodecs.imwrite(tempPath + Constant.TEMP_CHAR_SEP_AND_CLEAR, threshold); // 边缘膨胀 // 还原腐蚀操作产生的影响 // 会影响中文字符的精确度 threshold = ImageUtil.dilate(threshold, debug, tempPath, 2, 2, true); Imgcodecs.imwrite(tempPath + Constant.TEMP_CHAR_DILATE, threshold); // 提取外部轮廓 List<MatOfPoint> contours = Lists.newArrayList(); Imgproc.findContours(threshold, contours, new Mat(), Imgproc.RETR_EXTERNAL, Imgproc.CHAIN_APPROX_NONE); Vector<Rect> charRect = new Vector<Rect>(); // 字符轮廓集合 Mat dst; dst = inMat.clone(); Imgproc.cvtColor(threshold, dst, Imgproc.COLOR_GRAY2BGR); if (debug) {
这段代码是一个字符分割的函数,输入参数包括原始图像、车牌颜色、是否开启调试模式和临时文件保存路径。主要功能是对车牌图像进行预处理,包括切换到灰度图、二值化、边缘腐蚀、错切校正、垂直方向投影、字符分割、边缘膨胀和提取外部轮廓等步骤,最终输出字符轮廓集合。
在函数中,首先根据车牌颜色确定字符个数,然后将原始图像转换为灰度图并进行高斯模糊。接着根据不同车牌颜色使用不同的二值化方法,输出二值图并进行边缘腐蚀。然后进行错切校正,根据垂直方向投影进行精确定位,并根据垂直投影和垂直分割线进行字符分割,去掉上下边框和铆钉干扰。最后进行边缘膨胀,还原腐蚀操作产生的影响,并提取外部轮廓,输出字符轮廓集合。如果开启了调试模式,还会输出中间过程的图像以便调试。
Imgproc.cvtColor
Imgproc.cvtColor是OpenCV中的一个函数,用于将一个图像从一种颜色空间转换为另一种颜色空间。它的语法如下:
```java
Imgproc.cvtColor(Mat src, Mat dst, int code);
```
其中,src是输入图像,dst是输出图像,code是颜色空间转换类型的代码。常用的颜色空间转换类型包括:
- Imgproc.COLOR_BGR2GRAY:BGR彩色图像转灰度图像
- Imgproc.COLOR_BGR2HSV:BGR彩色图像转HSV颜色空间
- Imgproc.COLOR_BGR2Lab:BGR彩色图像转Lab颜色空间
- Imgproc.COLOR_BGR2RGB:BGR彩色图像转RGB彩色图像
等等。
阅读全文
相关推荐
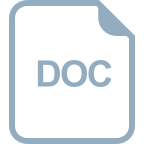
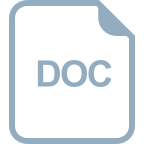
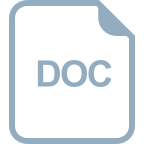





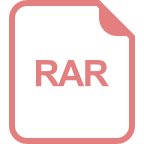
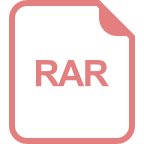
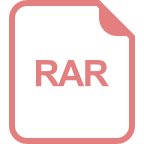






