#include <iostream> #include <opencv2/imgcodecs.hpp> #include <opencv2/imgproc.hpp> #include <opencv2/videoio.hpp> #include <opencv2/highgui.hpp> #include <opencv2/video.hpp> #include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui_c.h> using namespace cv; using namespace std; int main(int argc, char** argv) { VideoCapture capture("D:/dvp/sample/dataset/traffic.mp4"); if (!capture.isOpened()) { //error in opening the video input cerr << "Unable to open file!" << endl; return 0; } Mat frame, roi, hsv_roi, mask; // take first frame of the video capture >> frame; // setup initial location of window Rect track_window(300, 200, 100, 50); // simply hardcoded the values // set up the ROI for tracking roi = frame(track_window); cvtColor(roi, hsv_roi, COLOR_BGR2HSV); inRange(hsv_roi, Scalar(0, 60, 32), Scalar(180, 255, 255), mask); float range_[] = { 0, 180 }; const float* range[] = { range_ }; Mat roi_hist; int histSize[] = { 180 }; int channels[] = { 0 }; calcHist(&hsv_roi, 1, channels, mask, roi_hist, 1, histSize, range); normalize(roi_hist, roi_hist, 0, 255, NORM_MINMAX); // Setup the termination criteria, either 10 iteration or move by atleast 1 pt TermCriteria term_crit(TermCriteria::EPS | TermCriteria::COUNT, 10, 1); while (true) { Mat hsv, dst; capture >> frame; if (frame.empty()) break; cvtColor(frame, hsv, COLOR_BGR2HSV); calcBackProject(&hsv, 1, channels, roi_hist, dst, range); // apply meanshift to get the new location meanShift(dst, track_window, term_crit); // Draw it on image rectangle(frame, track_window, 255, 2); imshow("img2", frame); setMouseCallback("img2", onMouse, 0); int keyboard = waitKey(30); if (keyboard == 'q' || keyboard == 27) break; } }帮我更改此段代码,使其能够通过gui使用鼠标来框选指定区域
时间: 2024-01-29 11:03:07 浏览: 32
以下是修改后的代码,可以通过GUI使用鼠标来框选指定区域:
```
#include <iostream>
#include <opencv2/imgcodecs.hpp>
#include <opencv2/imgproc.hpp>
#include <opencv2/videoio.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/video.hpp>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui_c.h>
using namespace cv;
using namespace std;
Rect box; //定义一个矩形框,用于记录鼠标选择的区域
bool drawing_box = false; //标记是否正在通过鼠标绘制矩形框
void draw_box(Mat& img, Rect box)
{
//绘制矩形框
rectangle(img, box.tl(), box.br(), Scalar(0, 0, 255), 2);
}
void onMouse(int event, int x, int y, int flags, void* param)
{
Mat& image = *(Mat*)param;
switch (event)
{
case EVENT_MOUSEMOVE:
if (drawing_box)
{
//更新矩形框位置
box.width = x - box.x;
box.height = y - box.y;
}
break;
case EVENT_LBUTTONDOWN:
//开始绘制矩形框
drawing_box = true;
box = Rect(x, y, 0, 0);
break;
case EVENT_LBUTTONUP:
//结束绘制矩形框
drawing_box = false;
if (box.width < 0)
{
box.x += box.width;
box.width *= -1;
}
if (box.height < 0)
{
box.y += box.height;
box.height *= -1;
}
draw_box(image, box);
break;
}
}
int main(int argc, char** argv)
{
VideoCapture capture("D:/dvp/sample/dataset/traffic.mp4");
if (!capture.isOpened()) {
//error in opening the video input
cerr << "Unable to open file!" << endl;
return 0;
}
Mat frame, roi, hsv_roi, mask;
// take first frame of the video
capture >> frame;
namedWindow("img2");
setMouseCallback("img2", onMouse, &frame);
while (true) {
capture >> frame;
if (frame.empty()) break;
if (!box.empty()) {
// set up the ROI for tracking
roi = frame(box);
cvtColor(roi, hsv_roi, COLOR_BGR2HSV);
inRange(hsv_roi, Scalar(0, 60, 32), Scalar(180, 255, 255), mask);
float range_[] = { 0, 180 };
const float* range[] = { range_ };
Mat roi_hist;
int histSize[] = { 180 };
int channels[] = { 0 };
calcHist(&hsv_roi, 1, channels, mask, roi_hist, 1, histSize, range);
normalize(roi_hist, roi_hist, 0, 255, NORM_MINMAX);
// Setup the termination criteria, either 10 iteration or move by atleast 1 pt
TermCriteria term_crit(TermCriteria::EPS | TermCriteria::COUNT, 10, 1);
Mat hsv, dst;
cvtColor(frame, hsv, COLOR_BGR2HSV);
calcBackProject(&hsv, 1, channels, roi_hist, dst, range);
// apply meanshift to get the new location
meanShift(dst, box, term_crit);
// Draw it on image
rectangle(frame, box, 255, 2);
}
imshow("img2", frame);
int keyboard = waitKey(30);
if (keyboard == 'q' || keyboard == 27) break;
}
return 0;
}
```
在主函数中,我们添加了一个名为“img2”的窗口,并将其用作鼠标事件的回调函数。在主循环中,我们检查矩形框是否已经被绘制,如果是,则设置ROI并进行跟踪。最后,在每个循环迭代中,我们都显示图像,并等待用户按下“q”或“ESC”键退出程序。
相关推荐
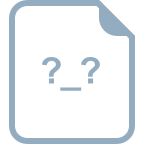
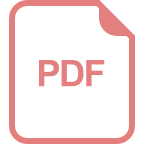
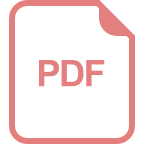















