#include <iostream> #include <opencv2/highgui.hpp> #include <opencv2/core.hpp> #include <opencv2/imgcodecs.hpp> #include <opencv2/opencv.hpp> using namespace cv; using namespace std; int main() { Mat img = imread("F:\\图像处理\\图片\\待修复图像.png"); if (img.empty()) { cout << "请检查文件名称是否有误!" << endl; return -1; } imshow("img", img); //转化为灰度图 Mat gray; cvtColor(img, gray, COLOR_BGR2GRAY); //通过阈值处理生成Mask掩码 Mat imgMask; threshold(gray, imgMask, 245, 255, THRESH_BINARY); //对Mask掩码膨胀处理,增加Mask的面积 Mat Kernel = getStructuringElement(MORPH_RECT, Size(3, 3)); dilate(imgMask, imgMask, Kernel); //图像修复 Mat imgInpaint; inpaint(img, imgMask, imgInpaint, 5, INPAINT_NS); //显示处理结果 imshow("imgMask", imgMask); imshow("img修复后", imgInpaint); waitKey(0); return 0; }
时间: 2024-03-19 21:41:44 浏览: 20
import cv2
img = cv2.imread("F:\\图像处理\\图片\\待修复图像.png")
if img is None:
print("请检查文件名称是否有误!")
else:
cv2.imshow("img", img)
# 转化为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 通过阈值处理生成Mask掩码
ret, imgMask = cv2.threshold(gray, 245, 255, cv2.THRESH_BINARY)
# 对Mask掩码膨胀处理,增加Mask的面积
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
imgMask = cv2.dilate(imgMask, kernel)
# 图像修复
imgInpaint = cv2.inpaint(img, imgMask, 5, cv2.INPAINT_NS)
# 显示处理结果
cv2.imshow("imgMask", imgMask)
cv2.imshow("img修复后", imgInpaint)
cv2.waitKey(0)
cv2.destroyAllWindows()
相关问题
#ifndef MY_HEADER_FILE_H #define MY_HEADER_FILE_H //#include "stdafx.h" #include <WINSOCK2.H> #include <iostream> #include <stdio.h> //#include <opencv/cv.h> #include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui.hpp> #include <opencv2/imgproc/imgproc.hpp>
这段代码中的条件编译指令 `#ifndef` 和 `#define` 是正确的,但是在这个指令的下面,又出现了包含其他头文件的指令 `#include`。如果这些头文件中包含了当前头文件,就可能导致文件包含自身的问题。
为了避免这种情况,建议将所有的 `#include` 指令放在条件编译指令之间,例如:
```
#ifndef MY_HEADER_FILE_H
#define MY_HEADER_FILE_H
// 包含其他头文件
#include "stdafx.h"
#include <WINSOCK2.H>
#include <iostream>
#include <stdio.h>
#include <opencv/cv.h>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
// 头文件内容
#endif
```
这样,可以确保在条件编译指令之前包含的头文件不会包含当前头文件,避免了文件包含自身的问题。
解释这个代码:#include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui.hpp> #include <opencv2/imgproc/imgproc.hpp> #include <iostream> using namespace cv; using namespace std; int main() { //载入图像 Mat image = imread("jiaoyan.jpg"); if (!image.data) //Check for invalid input { cout << "Could not open or find the image" << endl; return -1; } //创建窗口,显示原图 namedWindow("均值滤波原图"); imshow("均值滤波原图", image); //进行滤波 Mat out; blur(image, out, Size(3, 3)); namedWindow("均值滤波效果图"); imshow("均值滤波效果图", out); waitKey(0); return 0; }
这是一个使用 OpenCV 库进行图像处理的 C++ 代码。
首先,它引入了 OpenCV 库的核心、GUI 和图像处理模块的头文件,以及iostream库。
```#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <iostream>
using namespace cv;
using namespace std;
```
然后,它定义了一个主函数 `main()`。在主函数中,它通过 `imread()` 函数加载了一张名为 "jiaoyan.jpg" 的图像,并将其存储在 `Mat` 类型的变量 `image` 中。接着,通过判断 `image` 是否为空来检查图像是否加载成功。如果加载失败,程序将输出错误信息并返回 -1。
```
int main() {
// 载入图像
Mat image = imread("jiaoyan.jpg");
if (!image.data) // Check for invalid input
{
cout << "Could not open or find the image" << endl;
return -1;
}
```
接下来,它创建了一个名为 "均值滤波原图" 的窗口,并在该窗口中显示原始图像。
```
// 创建窗口,显示原图
namedWindow("均值滤波原图");
imshow("均值滤波原图", image);
```
然后,通过调用 `blur()` 函数进行均值滤波处理。均值滤波是一种常见的图像处理方法,它可以消除图像中的噪声,并平滑图像。在这个例子中,它使用了 3x3 的卷积核。
```
// 进行滤波
Mat out;
blur(image, out, Size(3, 3));
```
最后,它创建了一个名为 "均值滤波效果图" 的新窗口,并在该窗口中显示处理后的图像。
```
namedWindow("均值滤波效果图");
imshow("均值滤波效果图", out);
```
最后使用 `waitKey()` 函数等待用户按下键盘。
```
waitKey(0);
return 0;
}
```
这个程序的功能是加载图像并对其进行均值滤波处理,然后在窗口中显示原始图像和处理后的图像。
相关推荐
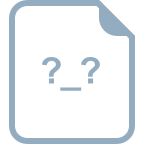
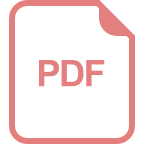
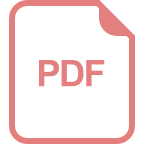












