java 单链表树结构node,孩子节点为children,怎么查询最深层数,请提供代码
时间: 2024-04-29 17:23:57 浏览: 30
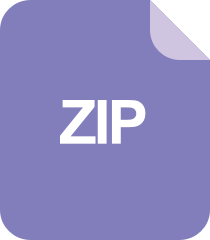
js代码-输出树各个节点的深度
可以使用递归来遍历整个树,每次递归时记录当前深度,最终返回最大深度即可。
以下是示例代码:
```
public class Node {
private int value;
private List<Node> children;
public Node(int value) {
this.value = value;
this.children = new ArrayList<>();
}
public int getValue() {
return value;
}
public List<Node> getChildren() {
return children;
}
public int getMaxDepth() {
return getMaxDepth(this, 0);
}
private int getMaxDepth(Node node, int depth) {
if (node.getChildren().isEmpty()) {
return depth;
} else {
int maxDepth = depth;
for (Node child : node.getChildren()) {
maxDepth = Math.max(maxDepth, getMaxDepth(child, depth + 1));
}
return maxDepth;
}
}
}
```
使用示例:
```
Node root = new Node(1);
Node child1 = new Node(2);
Node child2 = new Node(3);
Node child3 = new Node(4);
Node grandChild1 = new Node(5);
Node grandChild2 = new Node(6);
Node grandChild3 = new Node(7);
root.getChildren().add(child1);
root.getChildren().add(child2);
child1.getChildren().add(grandChild1);
child1.getChildren().add(grandChild2);
child2.getChildren().add(grandChild3);
child3.getChildren().add(new Node(8));
int maxDepth = root.getMaxDepth();
System.out.println("Max depth: " + maxDepth);
```
输出结果:
```
Max depth: 3
```
阅读全文
相关推荐
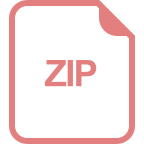
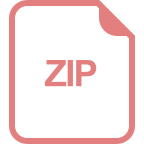
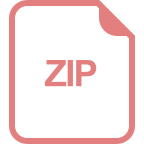
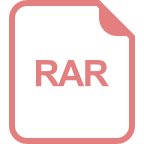
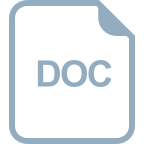
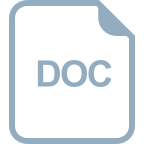
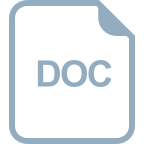









