matlab RMS error
时间: 2023-10-16 22:07:05 浏览: 184
In MATLAB, the RMS (Root Mean Square) error can be calculated using the `rms` function. The `rms` function takes an array or vector of values as input and returns the root mean square value. Here's an example:
```matlab
% Example data
predicted = [1.5, 2.0, 3.2, 4.8, 5.1];
actual = [1.8, 2.2, 3.4, 4.6, 5.0];
% Calculate RMS error
error = rms(predicted - actual);
```
In this example, the difference between the predicted and actual values is calculated, and then the `rms` function is used to compute the root mean square error. The resulting error value represents the average magnitude of the differences between the predicted and actual values.
相关问题
MATLAB的RMS计算矩阵误差
### 如何在MATLAB中使用RMS计算矩阵之间的误差
均方根误差(Root Mean Square Error, RMS)是衡量两个矩阵之间差异的一种常用度量方式。具体来说,对于给定的预测矩阵 \( \hat{X} \) 和真实矩阵 \( X \),可以通过以下公式计算它们之间的RMS误差:
\[ \text{RMS} = \sqrt{\frac{1}{mn}\sum_{i=1}^{m}\sum_{j=1}^{n}(X(i,j)-\hat{X}(i,j))^2 } \]
其中 \( m \) 是矩阵的行数,\( n \) 是列数。
下面是一个具体的MATLAB代码示例,用于计算两个相同大小矩阵间的RMS误差:
```matlab
function rmsError = calculateRMSError(X, X_hat)
% 计算两矩阵间RMS误差
% 获取矩阵尺寸
[rows, cols] = size(X);
% 验证输入矩阵是否具有相同的维度
if ~isequal(size(X), size(X_hat))
error('Input matrices must have the same dimensions.');
end
% 计算平方差之和
sumOfSquaredDifferences = sum(sum((X - X_hat).^2));
% 应用RMS公式
rmsError = sqrt(sumOfSquaredDifferences / (rows * cols));
end
```
此函数接受两个参数 `X` 和 `X_hat` ,分别代表原始的真实值矩阵以及预测或估算得到的结果矩阵,并返回两者之间的RMS误差值[^3]。
MATLAB ICP
### MATLAB中的ICP算法实现与使用
在MATLAB环境中,ICP(迭代最近点)算法用于配准两个三维点云数据集。该过程通过最小化两组几何特征之间的距离来找到最佳匹配位置和姿态。
#### 函数定义
为了简化操作并提高效率,可以创建一个名为`icp_registration.m`的函数文件[^1]:
```matlab
function [T, distances, iterations] = icp_registration(sourcePoints, targetPoints, maxIterations, tolerance)
% ICP_REGISTRATION performs Iterative Closest Point registration between two point clouds.
%
% Inputs:
% sourcePoints - Nx3 matrix representing the points to be registered (source).
% targetPoints - Mx3 matrix representing reference points (target).
% maxIterations - Maximum number of iterations allowed before stopping.
% tolerance - Threshold value used as convergence criteria.
% Initialize transformation parameters with identity matrix
T = eye(4);
distances = Inf;
iterations = 0;
while distances > tolerance && iterations < maxIterations
% Find nearest neighbors from source to target using KDTreeSearcher object
searcher = KDTreeSearcher(targetPoints);
[idx,dist] = knnsearch(searcher,sourcePoints,'K',1);
% Compute rigid body transform via singular value decomposition method
T_iter = computeRigidTransform(sourcePoints,targetPoints(idx,:));
% Update cumulative transformation parameter estimates
T = T * T_iter;
% Transform current set of moving points according to updated estimate
transformedSourcePts = homogeneousTransformation(T,sourcePoints);
% Calculate mean squared error after applying new estimated pose change
distances = sqrt(sum((transformedSourcePts-targetPoints(idx,:)).^2,2))';
% Increment iteration counter
iterations = iterations + 1;
end
disp(['Number of iterations:', num2str(iterations)]);
disp(['Final RMS distance : ',num2str(mean(distances))]);
end
function H = homogeneousTransformation(transformMatrix,points)
% Convert Cartesian coordinates into Homogeneous form by appending ones column vector,
% apply given affine transformation represented through a square matrix,
% finally convert back results obtained in standard coordinate system format
N=size(points,1);
H=[ones(N,1), points]*transformMatrix';
end
function R = computeRigidTransform(A,B)
% Computes optimal rotation & translation matrices that aligns one dataset onto another based on SVD approach described here: http://nghiaho.com/?page_id=671
assert(size(A)==size(B));
centroid_A = mean(A,1);
centroid_B = mean(B,1);
AA = bsxfun(@minus,A,centroid_A);
BB = bsxfun(@minus,B,centroid_B);
H = AA'*BB;
[U,S,V] = svd(H);
R = V*U';
if det(R)<0
V(:,3)=-V(:,3);
R = V*U';
end
t = centroid_B'-(R*centroid_A');
R = [[R,t'];[zeros(1,3),1]];
end
```
此代码实现了完整的ICP流程,包括寻找最邻近点、计算刚体变换以及更新源点的位置直到满足收敛条件为止。此外还提供了辅助功能如齐次坐标转换和基于奇异值分解(SVD)的方法求解最优旋转和平移矩阵。
对于实际应用而言,可能还需要考虑更多细节优化性能或处理特殊情况,比如当输入的数据存在噪声时如何改进鲁棒性等问题。
阅读全文
相关推荐






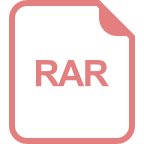









