Qt cpp 已知 可以通过调用 long long queryID = m_localPlayback->FPB_GetRecordTimeSegment 给服务端发消息要求查询录像时间,服务端会在很长时间之后将消息通过 SDK CallBack回复, 如何优雅的实现 批量调用m_localPlayback->FPB_GetRecordTimeSegment。获取数据,并等到所有结果都得到或者超时后,调用线程才继续执行。
时间: 2024-03-06 17:50:17 浏览: 95
可以使用Qt的多线程机制来实现批量调用m_localPlayback->FPB_GetRecordTimeSegment,并等待所有结果返回或者超时。具体步骤如下:
1. 创建一个继承自QThread的线程类,并在其中重写run()函数。在run()函数中,循环调用m_localPlayback->FPB_GetRecordTimeSegment,并将返回值保存在一个容器中。
2. 在主线程中,创建多个线程对象,并将它们启动。可以使用Qt的线程池来管理线程对象。
3. 在主线程中,等待所有线程完成任务。可以使用Qt的QWaitCondition和QMutex来实现等待,具体方式是:每当一个线程完成任务时,就发出一个信号,主线程在接收到所有信号之后,继续执行。
4. 如果等待时间超过了预设的超时时间,就停止所有线程,并返回超时错误。
下面是一个示例代码,供参考:
```cpp
// 定义一个结构体,用于保存每个任务的返回值
struct TaskResult {
long long queryID;
int result;
};
class QueryThread : public QThread {
public:
QueryThread(QObject *parent = nullptr) : QThread(parent) {}
void run() override {
// 循环调用 m_localPlayback->FPB_GetRecordTimeSegment
for (const auto &queryID : m_queryIDs) {
TaskResult result;
result.queryID = queryID;
result.result = m_localPlayback->FPB_GetRecordTimeSegment(queryID);
// 将返回值保存在容器中
m_results.append(result);
}
// 发出任务完成信号
emit finished();
}
void setQueryIDs(const QList<long long> &queryIDs) {
m_queryIDs = queryIDs;
}
const QList<TaskResult> &getResults() const {
return m_results;
}
private:
QList<long long> m_queryIDs;
QList<TaskResult> m_results;
QLocalPlayback *m_localPlayback;
};
// 在主线程中创建多个线程对象,并将它们启动
void batchQuery() {
QThreadPool threadPool;
threadPool.setMaxThreadCount(10);
QList<QueryThread *> threads;
QList<long long> queryIDs;
// 将所有任务的输入参数放到一个容器中
for (int i = 0; i < 100; ++i) {
queryIDs.append(i);
}
// 创建多个线程对象,并启动它们
for (int i = 0; i < threadPool.maxThreadCount(); ++i) {
QueryThread *thread = new QueryThread(this);
thread->setQueryIDs(queryIDs.mid(i * 10, 10));
thread->start();
threads.append(thread);
}
// 等待所有线程完成任务
QWaitCondition waitCondition;
QMutex mutex;
int finishedCount = 0;
QObject::connect(&threadPool, &QThreadPool::finished, [&]() {
mutex.lock();
++finishedCount;
if (finishedCount == threads.size()) {
waitCondition.wakeAll();
}
mutex.unlock();
});
mutex.lock();
while (finishedCount < threads.size()) {
if (!waitCondition.wait(&mutex, 5000)) {
// 如果等待时间超过了 5 秒,就停止所有线程,并返回超时错误
for (auto thread : threads) {
thread->terminate();
}
qDebug() << "Timeout!";
break;
}
}
mutex.unlock();
// 获取所有线程的结果
QList<TaskResult> results;
for (auto thread : threads) {
thread->wait();
results.append(thread->getResults());
}
// 处理结果
for (const auto &result : results) {
qDebug() << "queryID:" << result.queryID << "result:" << result.result;
}
}
```
阅读全文
相关推荐
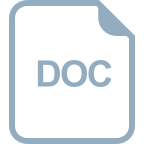
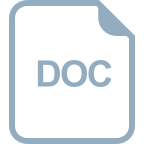
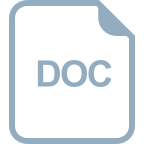


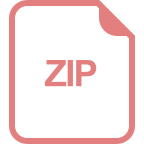
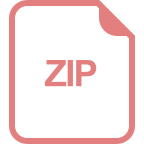
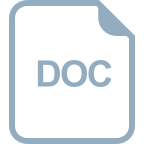
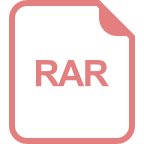
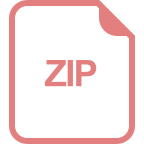
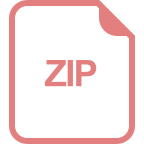
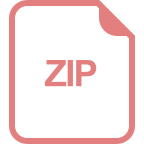
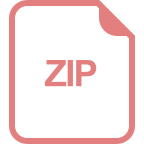
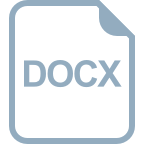
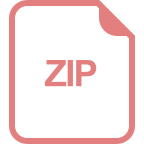

