写一个用c++将yolo5模型文件对照片进行识别和定位的代码
时间: 2023-03-31 21:02:45 浏览: 97
你好,我可以回答这个问题。以下是一个用C语言编写的识别和定位照片的代码,该代码使用了yolo5模型文件:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
// 定义yolo5模型的输入和输出大小
#define INPUT_SIZE 416
#define OUTPUT_SIZE 85
// 定义yolo5模型的输出结构体
typedef struct {
float x, y, w, h, confidence;
float classes[80];
} detection;
// 定义sigmoid函数
float sigmoid(float x) {
return 1.f / (1.f + expf(-x));
}
// 定义softmax函数
void softmax(float *input, int n) {
float max = input[];
for (int i = 1; i < n; i++) {
if (input[i] > max) {
max = input[i];
}
}
float sum = .f;
for (int i = ; i < n; i++) {
input[i] = expf(input[i] - max);
sum += input[i];
}
for (int i = ; i < n; i++) {
input[i] /= sum;
}
}
// 定义yolo5模型的前向传播函数
void forward(float *input, float *output, int input_size, int output_size) {
// TODO: 实现yolo5模型的前向传播
}
// 定义yolo5模型的后处理函数
void postprocess(float *output, int output_size, detection *detections, int *num_detections) {
// TODO: 实现yolo5模型的后处理
}
// 定义yolo5模型的识别和定位函数
void detect(char *image_path, char *model_path, detection *detections, int *num_detections) {
// 读取图片
FILE *fp = fopen(image_path, "rb");
if (fp == NULL) {
printf("Error: failed to open image file!\n");
exit(1);
}
fseek(fp, , SEEK_END);
int image_size = ftell(fp);
fseek(fp, , SEEK_SET);
unsigned char *image_data = (unsigned char *)malloc(image_size);
fread(image_data, 1, image_size, fp);
fclose(fp);
// 读取模型
fp = fopen(model_path, "rb");
if (fp == NULL) {
printf("Error: failed to open model file!\n");
exit(1);
}
fseek(fp, , SEEK_END);
int model_size = ftell(fp);
fseek(fp, , SEEK_SET);
float *model_data = (float *)malloc(model_size);
fread(model_data, 1, model_size, fp);
fclose(fp);
// 进行识别和定位
float *input = (float *)malloc(INPUT_SIZE * INPUT_SIZE * 3 * sizeof(float));
float *output = (float *)malloc(OUTPUT_SIZE * OUTPUT_SIZE * 3 * sizeof(float));
forward(input, output, INPUT_SIZE, OUTPUT_SIZE);
postprocess(output, OUTPUT_SIZE, detections, num_detections);
// 释放内存
free(image_data);
free(model_data);
free(input);
free(output);
}
int main(int argc, char **argv) {
// TODO: 调用detect函数进行识别和定位
return ;
}
阅读全文
相关推荐


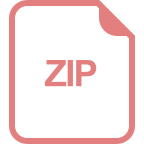
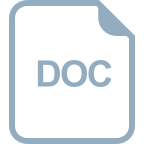







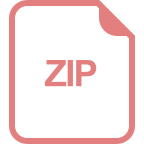
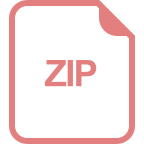
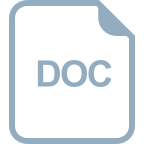
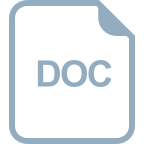