接收键盘输入的字符串,用fileoutputstream类将字符串写入文件,用fileinputstream类读出文件内容显示在屏幕上。
时间: 2023-05-31 07:17:51 浏览: 252
### 回答1:
首先,需要使用Scanner类接收键盘输入的字符串,然后使用FileOutputStream类将字符串写入文件。代码如下:
```
import java.io.*;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要写入文件的字符串:");
String str = scanner.nextLine();
try {
FileOutputStream fos = new FileOutputStream("test.txt");
fos.write(str.getBytes());
fos.close();
System.out.println("写入文件成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
接下来,使用FileInputStream类读取文件内容,并将内容显示在屏幕上。代码如下:
```
import java.io.*;
public class Main {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("test.txt");
byte[] bytes = new byte[1024];
int len = ;
while ((len = fis.read(bytes)) != -1) {
System.out.println(new String(bytes, , len));
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上就是接收键盘输入的字符串,用FileOutputStream类将字符串写入文件,用FileInputStream类读出文件内容显示在屏幕上的代码实现。
### 回答2:
首先,需要在程序中接收键盘输入的字符串。我们可以使用Scanner类来获取控制台输入的字符串。代码如下:
```
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要写入文件的内容:");
String content = scanner.nextLine();
```
接下来,我们使用FileOutputStream类将字符串写入文件。需要先创建一个File对象,指定文件路径和文件名。代码如下:
```
File file = new File("test.txt");
FileOutputStream fos = new FileOutputStream(file);
byte[] bytes = content.getBytes();
fos.write(bytes);
fos.close();
```
在这里,将字符串转为字节数组,再使用write方法将字节数组写入文件。最后关闭输出流。
接下来,我们使用FileInputStream类读出文件内容,并显示在屏幕上。代码如下:
```
FileInputStream fis = new FileInputStream(file);
byte[] bytes = new byte[(int) file.length()];
int len;
while ((len = fis.read(bytes)) != -1) {
System.out.println(new String(bytes, 0, len));
}
fis.close();
```
这里要注意,读取字节时,要根据文件的长度来指定字节数组的大小。使用while循环读取字节,直到文件结束。将读取到的字节数组转为字符串,并输出到屏幕上。最后关闭输入流。
完整代码如下:
```
import java.io.*;
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要写入文件的内容:");
String content = scanner.nextLine();
File file = new File("test.txt");
try {
FileOutputStream fos = new FileOutputStream(file);
byte[] bytes = content.getBytes();
fos.write(bytes);
fos.close();
FileInputStream fis = new FileInputStream(file);
bytes = new byte[(int) file.length()];
int len;
while ((len = fis.read(bytes)) != -1) {
System.out.println(new String(bytes, 0, len));
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
运行程序,可以输入要写入文件的字符串,然后在控制台中显示出来。接着程序会将字符串写入文件,并读出并输出到屏幕上。
### 回答3:
接收键盘输入的字符串可以使用Scanner类,代码示例如下:
```
import java.util.Scanner;
public class FileWrite {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要写入文件的字符串:");
String str = scanner.nextLine();
// 使用FileOutputStream将字符串写入文件
try {
FileOutputStream fos = new FileOutputStream("test.txt", true);
fos.write(str.getBytes());
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
// 使用FileInputStream读取文件内容并显示在屏幕上
try {
FileInputStream fis = new FileInputStream("test.txt");
byte[] bytes = new byte[fis.available()];
fis.read(bytes);
fis.close();
String content = new String(bytes);
System.out.println("从文件读取的内容如下:");
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
上述代码中,先使用Scanner类接收键盘输入的字符串,然后使用FileOutputStream类将字符串写入文件,FileOutputStream的构造函数中第一个参数是文件名,第二个参数是是否以追加的方式写入文件。接着使用FileInputStream类读取文件内容,FileInputStream的构造函数中只需要传入文件名即可。由于读取文件内容后返回的是字节数组,因此需要将其转换成字符串后再输出。完成后运行程序,便可在test.txt文件中看到输入的字符串,并在控制台上显示该文件内容。
相关推荐
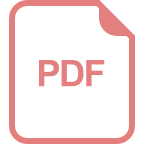
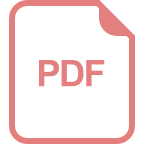
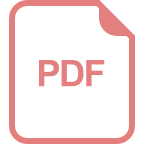













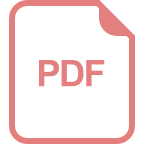
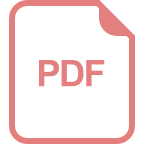