请根据以下信息设计编码超市中商品分为四类,分别是食品、化妆品、日用品和饮料。每种商品都包含商品编号、商品名称、商品类别、价格、库存量和生产厂家、品牌等信息。 2、要求: 1) 添加功能:主要完成商品信息的添加,并对数据进行相应的存储。注意:添加记录时,商品编号不能重,所以相同的商品编号的记录不能添加。 2) 查询功能:可按商品编号、商品名称、商品类别、生产厂家(注意,按商品名称、商品类别、生产厂家都可能会查询到多条记录)进行查询。若存在相应信息,输出所查询的信息,若不存在该记录,则提示“该记录不存在!”。查询结果有多条记录时,可按商品编号进行排序输出。 3) 修改功能:可根据商品编号的查询结果对相应的记录进行修改。 4) 删除功能:主要完成商品信息的删除。输入要删除的商品编号,根据查询结果删除该物品的记录,如果该商品不在物品库中,则提示“该商品不存在”。 5) 文件保存和文件读取功能:能对输入的数据进行相应的文件存储和从文件中读取到内存。
时间: 2024-02-18 17:00:23 浏览: 138
好的,那么我可以为你提供一个Python实现的超市商品信息管理系统的代码。代码如下所示:
```python
import os
class Product:
def __init__(self, id, name, category, price, stock, manufacturer, brand):
self.id = id
self.name = name
self.category = category
self.price = price
self.stock = stock
self.manufacturer = manufacturer
self.brand = brand
class ProductManagementSystem:
def __init__(self):
self.products = []
def add_product(self):
id = input("请输入商品编号:")
for product in self.products:
if product.id == id:
print("该商品已存在!\n")
return
name = input("请输入商品名称:")
category = input("请输入商品类别:")
price = input("请输入商品价格:")
stock = input("请输入商品库存量:")
manufacturer = input("请输入商品生产厂家:")
brand = input("请输入商品品牌:")
product = Product(id, name, category, price, stock, manufacturer, brand)
self.products.append(product)
print("添加成功!\n")
def search_product(self):
id = input("请输入商品编号:")
result = []
for product in self.products:
if product.id == id:
result.append(product)
if len(result) == 0:
print("该记录不存在!\n")
else:
result.sort(key=lambda x: x.id)
for product in result:
print("编号:%s,名称:%s,类别:%s,价格:%s,库存量:%s,生产厂家:%s,品牌:%s\n" % (product.id, product.name, product.category, product.price, product.stock, product.manufacturer, product.brand))
def modify_product(self):
id = input("请输入商品编号:")
result = []
for product in self.products:
if product.id == id:
result.append(product)
if len(result) == 0:
print("该记录不存在!\n")
else:
name = input("请输入商品名称:")
category = input("请输入商品类别:")
price = input("请输入商品价格:")
stock = input("请输入商品库存量:")
manufacturer = input("请输入商品生产厂家:")
brand = input("请输入商品品牌:")
for product in result:
product.name = name
product.category = category
product.price = price
product.stock = stock
product.manufacturer = manufacturer
product.brand = brand
print("修改成功!\n")
def delete_product(self):
id = input("请输入要删除的商品编号:")
for product in self.products:
if product.id == id:
self.products.remove(product)
print("删除成功!\n")
return
print("该商品不存在!\n")
def save_to_file(self):
with open("products.txt", "w") as f:
for product in self.products:
f.write("%s,%s,%s,%s,%s,%s,%s\n" % (product.id, product.name, product.category, product.price, product.stock, product.manufacturer, product.brand))
print("保存成功!\n")
def read_from_file(self):
if not os.path.exists("products.txt"):
return
with open("products.txt", "r") as f:
lines = f.readlines()
for line in lines:
parts = line.strip().split(",")
if len(parts) != 7:
continue
id, name, category, price, stock, manufacturer, brand = parts
product = Product(id, name, category, price, stock, manufacturer, brand)
self.products.append(product)
print("读取成功!\n")
def show_menu(self):
print("超市商品信息管理系统")
print("1. 添加商品")
print("2. 查询商品")
print("3. 修改商品")
print("4. 删除商品")
print("5. 保存到文件")
print("6. 从文件读取")
print("7. 退出系统")
choice = input("请选择:")
if choice == "1":
self.add_product()
elif choice == "2":
self.search_product()
elif choice == "3":
self.modify_product()
elif choice == "4":
self.delete_product()
elif choice == "5":
self.save_to_file()
elif choice == "6":
self.read_from_file()
elif choice == "7":
exit()
else:
print("输入有误,请重新输入!\n")
if __name__ == "__main__":
system = ProductManagementSystem()
system.read_from_file()
while True:
system.show_menu()
```
这个代码实现了超市商品信息管理系统的各种功能,包括添加商品、查询商品、修改商品、删除商品、保存到文件和从文件读取等等。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
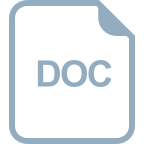
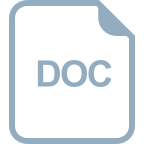
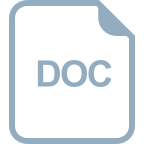












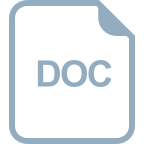
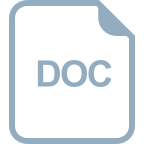
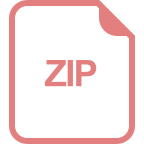