nsga-ii python
时间: 2023-09-02 11:08:43 浏览: 115
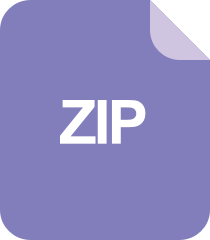
NSGA-II源代码
NSGA-II (Non-dominated Sorting Genetic Algorithm II) is a popular multi-objective optimization algorithm that is widely used in various fields such as engineering, finance, and biology. It is an extension of the standard genetic algorithm and uses a non-dominated sorting technique to rank the solutions based on their dominance relationship.
To implement NSGA-II in Python, we can use the DEAP (Distributed Evolutionary Algorithms in Python) library. DEAP provides a comprehensive set of tools for implementing various evolutionary algorithms, including NSGA-II.
Here is a simple example of how to use DEAP to implement NSGA-II in Python:
```python
import random
from deap import base, creator, tools, algorithms
# Define the fitness function (minimize two objectives)
creator.create("FitnessMin", base.Fitness, weights=(-1.0, -1.0))
# Define the individual class (a list of two floats)
creator.create("Individual", list, fitness=creator.FitnessMin)
# Initialize the toolbox
toolbox = base.Toolbox()
# Define the range of the two objectives
BOUND_LOW, BOUND_UP = 0.0, 1.0
# Define the evaluation function (two objectives)
def evaluate(individual):
return individual[0], individual[1]
# Register the evaluation function and the individual class
toolbox.register("evaluate", evaluate)
toolbox.register("individual", tools.initCycle, creator.Individual,
(random.uniform(BOUND_LOW, BOUND_UP) for _ in range(2)), n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# Define the genetic operators
toolbox.register("mate", tools.cxSimulatedBinaryBounded, low=BOUND_LOW, up=BOUND_UP, eta=20.0)
toolbox.register("mutate", tools.mutPolynomialBounded, low=BOUND_LOW, up=BOUND_UP, eta=20.0, indpb=1.0/2)
toolbox.register("select", tools.selNSGA2)
# Define the main function
def main(seed=0):
random.seed(seed)
# Initialize the population
pop = toolbox.population(n=100)
# Evaluate the initial population
fitnesses = [toolbox.evaluate(ind) for ind in pop]
for ind, fit in zip(pop, fitnesses):
ind.fitness.values = fit
# Run the algorithm
pop = algorithms.eaMuPlusLambda(pop, toolbox, mu=100, lambda_=100, cxpb=0.9, mutpb=0.1, ngen=100, verbose=False)
# Print the final population
print("Final population:")
for ind in pop:
print(ind, ind.fitness.values)
if __name__ == "__main__":
main()
```
This code defines a simple two-objective optimization problem and uses NSGA-II to find the Pareto front. The `creator` module is used to define the fitness and individual classes. The `toolbox` is used to register the genetic operators and the evaluation function. Finally, the `algorithms` module is used to run the algorithm and obtain the Pareto front.
阅读全文
相关推荐
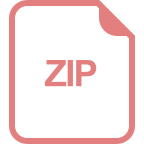

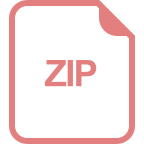
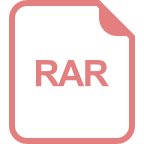




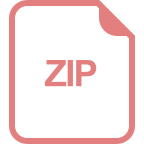



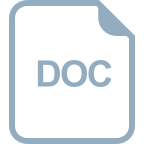

