三方演化博弈仿真 python
时间: 2023-10-04 18:06:46 浏览: 374
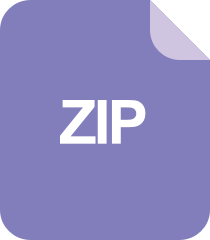
演化博弈仿真
在演化博弈中,三方演化博弈是指有三个个体参与的博弈过程。在Python中,可以使用Matplotlib库来进行三方演化博弈的仿真。
下面是一个简单的示例代码,实现了三方演化博弈的仿真:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义三方演化博弈的参与者策略
strategies = np.array([[1, 0, 0], [0, 1, 0], [0, 0, 1]])
# 定义三方演化博弈的收益矩阵
payoff_matrix = np.array([[3, 0, 0], [0, 2, 1], [0, 1, 2]])
# 定义三方演化博弈的演化规则
def evolution_rule(population):
# 计算每个个体的平均收益
average_payoff = np.dot(payoff_matrix, population)
# 选择平均收益最高的个体作为下一代
next_generation = strategies[np.argmax(average_payoff)]
return next_generation
# 定义演化轮数
num_generations = 100
# 定义初始个体分布
initial_population = np.array([0.3, 0.3, 0.4])
# 进行演化博弈仿真
population_history = [initial_population]
for _ in range(num_generations):
next_population = evolution_rule(population_history[-1])
population_history.append(next_population)
# 绘制演化过程
population_history = np.array(population_history)
plt.plot(population_history[:, 0], label='Player 1')
plt.plot(population_history[:, 1], label='Player 2')
plt.plot(population_history[:, 2], label='Player 3')
plt.xlabel('Generation')
plt.ylabel('Population')
plt.legend()
plt.show()
```
上述代码中,我们首先定义了三方演化博弈的参与者策略和收益矩阵。然后,通过演化规则计算每一代的个体分布,并将其保存在`population_history`列表中。最后,使用Matplotlib库将演化过程可视化出来。
请注意,以上只是一个简单的示例,实际的三方演化博弈可能涉及更复杂的策略和收益矩阵,具体情况可以根据需求进行相应的调整。
阅读全文
相关推荐
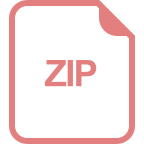

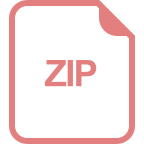
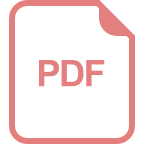
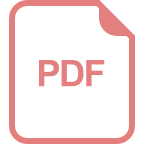
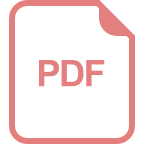
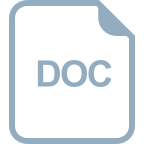








