基于esp-idf 帮我写一个iic代码
时间: 2024-05-15 10:13:35 浏览: 126
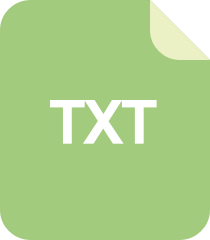
iic文档代码
好的,以下是一个简单的 ESP-IDF I2C 代码:
```c
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/i2c.h"
#define I2C_MASTER_SCL_IO 22 /*!< gpio number for I2C master clock */
#define I2C_MASTER_SDA_IO 21 /*!< gpio number for I2C master data */
#define I2C_MASTER_NUM I2C_NUM_0 /*!< I2C port number for master dev */
#define I2C_MASTER_FREQ_HZ 100000 /*!< I2C master clock frequency */
#define I2C_MASTER_TX_BUF_DISABLE 0 /*!< I2C master doesn't need buffer */
#define I2C_MASTER_RX_BUF_DISABLE 0 /*!< I2C master doesn't need buffer */
static void i2c_master_init()
{
int i2c_master_port = I2C_MASTER_NUM;
i2c_config_t conf;
conf.mode = I2C_MODE_MASTER;
conf.sda_io_num = I2C_MASTER_SDA_IO;
conf.sda_pullup_en = GPIO_PULLUP_ENABLE;
conf.scl_io_num = I2C_MASTER_SCL_IO;
conf.scl_pullup_en = GPIO_PULLUP_ENABLE;
conf.master.clk_speed = I2C_MASTER_FREQ_HZ;
i2c_param_config(i2c_master_port, &conf);
i2c_driver_install(i2c_master_port, conf.mode, I2C_MASTER_TX_BUF_DISABLE, I2C_MASTER_RX_BUF_DISABLE, 0);
}
void i2c_master_write_slave(uint8_t address, uint8_t reg, uint8_t data)
{
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (address << 1) | I2C_MASTER_WRITE, true);
i2c_master_write_byte(cmd, reg, true);
i2c_master_write_byte(cmd, data, true);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
}
void i2c_master_read_slave(uint8_t address, uint8_t reg, uint8_t *data, size_t size)
{
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (address << 1) | I2C_MASTER_WRITE, true);
i2c_master_write_byte(cmd, reg, true);
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (address << 1) | I2C_MASTER_READ, true);
if (size > 1) {
i2c_master_read(cmd, data, size - 1, I2C_MASTER_ACK);
}
i2c_master_read_byte(cmd, data + size - 1, I2C_MASTER_NACK);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
}
void i2c_test_task(void *arg)
{
uint8_t data = 0;
while (1) {
// 向从设备写入数据
i2c_master_write_slave(0x3C, 0x00, 0x01);
// 从从设备读取数据
i2c_master_read_slave(0x3C, 0x00, &data, 1);
printf("Read data from slave: 0x%02X\n", data);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
void app_main()
{
i2c_master_init();
xTaskCreate(i2c_test_task, "i2c_test_task", 1024 * 2, NULL, 10, NULL);
}
```
该代码通过 `i2c_master_init()` 初始化 I2C 主机,并提供了两个函数:`i2c_master_write_slave()` 和 `i2c_master_read_slave()`,用于向从设备写入数据和从从设备读取数据。
在 `i2c_test_task()` 中,我们首先向从设备写入数据(0x01),然后从从设备读取一个字节的数据,并将其打印到控制台上。这个任务会每秒钟重复执行一次。
注意:在使用此代码之前,请确保您已正确连接 I2C 总线和从设备,并将它们的地址设置为 0x3C。
阅读全文
相关推荐
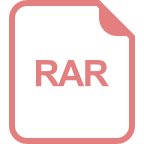
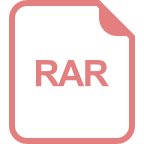
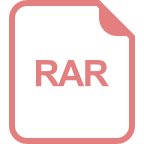
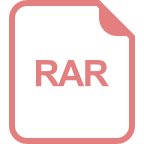
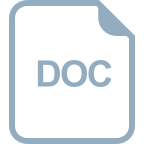
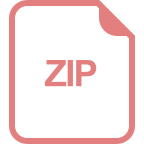
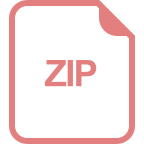
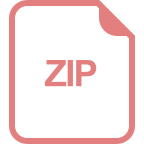
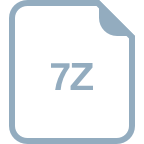
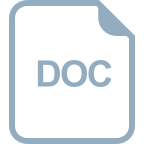



