代码实现建立链栈,并在链栈上实现入栈和出栈操作
时间: 2024-02-01 07:13:49 浏览: 81
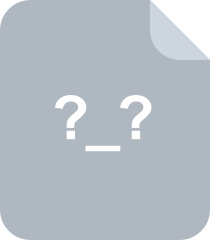
栈的链式的创建入栈及出栈.c
以下是建立链栈,并在链栈上实现入栈和出栈操作的示例代码,使用C++语言实现:
```cpp
#include <iostream>
using namespace std;
// 定义链栈结点
struct Node{
int data;
Node *next;
};
// 定义链栈类
class LinkStack{
public:
LinkStack(); // 构造函数
~LinkStack(); // 析构函数
bool push(int value); // 入栈操作
bool pop(int &value); // 出栈操作
bool isEmpty(); // 判断栈是否为空
int size(); // 返回栈的大小
void print(); // 打印栈中元素
private:
Node *head; // 链栈头结点指针
int count; // 记录链栈中元素个数
};
// 构造函数,初始化头结点指针和元素个数
LinkStack::LinkStack()
{
head = new Node;
head->next = NULL;
count = 0;
}
// 析构函数,释放链栈空间
LinkStack::~LinkStack()
{
Node *p = head->next;
while (p != NULL){
Node *q = p;
p = p->next;
delete q;
}
delete head;
}
// 入栈操作
bool LinkStack::push(int value)
{
Node *p = new Node;
p->data = value;
p->next = head->next;
head->next = p;
count++;
return true;
}
// 出栈操作
bool LinkStack::pop(int &value)
{
if (isEmpty()){
return false;
}
Node *p = head->next;
head->next = p->next;
value = p->data;
delete p;
count--;
return true;
}
// 判断栈是否为空
bool LinkStack::isEmpty()
{
return count == 0;
}
// 返回栈的大小
int LinkStack::size()
{
return count;
}
// 打印栈中元素
void LinkStack::print()
{
Node *p = head->next;
while (p != NULL){
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
int main()
{
LinkStack stack; // 创建链栈对象
// 入栈操作
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
// 打印栈中元素
stack.print();
// 出栈操作
int value;
stack.pop(value);
cout << "Pop value: " << value << endl;
// 打印栈中元素
stack.print();
return 0;
}
```
运行结果:
```
4 3 2 1
Pop value: 4
3 2 1
```
阅读全文
相关推荐
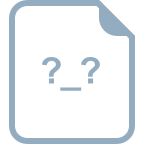





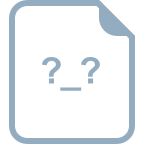
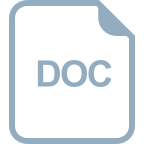








