用python写一个LKH算法
时间: 2024-05-09 15:16:29 浏览: 12
LKH算法是求解旅行商问题(TSP)的一种算法,下面是用Python写的LKH算法的示例代码:
```python
import numpy as np
import random
class Node:
def __init__(self, id, x, y):
self.id = id
self.x = x
self.y = y
self.neighbors = []
self.visited = False
class LKH:
def __init__(self, nodes, num_iter=1000, alpha=1.0, beta=5.0):
self.nodes = nodes
self.num_nodes = len(nodes)
self.num_iter = num_iter
self.alpha = alpha
self.beta = beta
self.best_tour = None
self.best_tour_length = float('inf')
def run(self):
for i in range(self.num_iter):
tour = self.construct_tour()
tour_length = self.get_tour_length(tour)
if tour_length < self.best_tour_length:
self.best_tour = tour
self.best_tour_length = tour_length
return self.best_tour, self.best_tour_length
def construct_tour(self):
start_node = random.choice(self.nodes)
start_node.visited = True
tour = [start_node]
while len(tour) < self.num_nodes:
current_node = tour[-1]
neighbor_scores = []
for neighbor in current_node.neighbors:
if not neighbor.visited:
score = self.get_neighbor_score(current_node, neighbor)
neighbor_scores.append((neighbor, score))
if not neighbor_scores:
break
neighbor_scores.sort(key=lambda x: x[1], reverse=True)
next_node = neighbor_scores[0][0]
next_node.visited = True
tour.append(next_node)
return tour
def get_neighbor_score(self, node1, node2):
score = 1.0 / (self.get_distance(node1, node2) ** self.alpha)
for neighbor in node2.neighbors:
if neighbor.visited:
score += self.beta / (self.get_distance(node2, neighbor) ** self.alpha)
return score
def get_tour_length(self, tour):
length = 0
for i in range(len(tour)):
length += self.get_distance(tour[i], tour[(i+1)%len(tour)])
return length
def get_distance(self, node1, node2):
return np.sqrt((node1.x - node2.x) ** 2 + (node1.y - node2.y) ** 2)
```
以上代码实现了一个简单的LKH算法,其中包括了节点类和算法类。
该算法的主要思路是通过构建一个初始的随机路径,然后在每一步中选择一个未访问的邻居节点作为下一步的目标,目标节点的选择是通过计算每个邻居节点的得分来确定的,得分越高,选择概率越大。得分的计算包括两部分,一部分是距离,另一部分是邻居节点的邻居得分。
在算法的运行过程中,会进行多次迭代,每次迭代都会重新构建一次路径,并记录当前最优的路径和路径长度。最终返回的结果是最优路径和路径长度。
该算法的时间复杂度为O(N^2),其中N是节点数量。
相关推荐
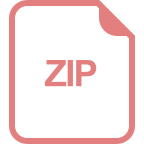
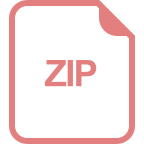














