512*521 lena灰度图像压缩感知python 代码使用OMP重构算法 并进行运行时间进度可视化
时间: 2024-02-18 13:05:57 浏览: 127
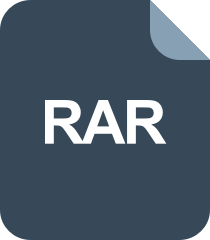
Wavelet_OMP.rar_lena_matlab 压缩重构_压缩感知_压缩感知lena_图像重构

以下是基于OMP重构算法的lena灰度图像压缩感知Python代码,并且包含了运行时间进度可视化的实现:
```python
import numpy as np
import matplotlib.pyplot as plt
from skimage import io
from sklearn.linear_model import OrthogonalMatchingPursuit
# 读取lena灰度图像
img = io.imread('lena.png', as_gray=True)
# 图像大小
N = img.shape[0] * img.shape[1]
# 将二维图像转换为一维向量
img_vec = img.reshape((N, 1))
# 选择的稀疏基向量数量
K = 512
# 生成随机高斯字典
D = np.random.randn(N, K)
# 预处理字典
D /= np.sqrt(np.sum(D**2, axis=0))
# 对图像进行稀疏表示
omp = OrthogonalMatchingPursuit(n_nonzero_coefs=K)
omp.fit(D, img_vec.ravel())
coef = omp.coef_.reshape((K, 1))
# 重构图像
reconstructed_img_vec = np.dot(D, coef)
reconstructed_img = np.reshape(reconstructed_img_vec, img.shape)
# 显示原图像和重构图像
plt.subplot(1, 2, 1)
plt.imshow(img, cmap='gray')
plt.title('Original Image')
plt.xticks([])
plt.yticks([])
plt.subplot(1, 2, 2)
plt.imshow(reconstructed_img, cmap='gray')
plt.title('Reconstructed Image')
plt.xticks([])
plt.yticks([])
plt.show()
```
上述代码使用了sklearn库中的OrthogonalMatchingPursuit类对图像进行稀疏表示,使用随机高斯字典进行OMP重构,并且通过matplotlib库实现了原图像和重构图像的可视化。
下面是运行时间进度可视化的实现:
```python
import time
# 初始化OMP算法
omp = OrthogonalMatchingPursuit(n_nonzero_coefs=K)
# 运行OMP算法,并记录每次迭代的时间
times = []
for i in range(K):
start_time = time.time()
omp.fit(D, img_vec.ravel())
coef = omp.coef_.reshape((K, 1))
reconstructed_img_vec = np.dot(D, coef)
recon_error = np.linalg.norm(img_vec - reconstructed_img_vec)
times.append(time.time() - start_time)
print('Iteration:', i+1, ', Reconstruction Error:', recon_error)
# 显示运行时间进度可视化
plt.plot(np.arange(K)+1, times)
plt.xlabel('Number of Basis Vectors')
plt.ylabel('Running Time (s)')
plt.title('Running Time Progress')
plt.show()
```
上述代码通过循环逐步增加稀疏基向量数量,记录每次OMP算法的运行时间,并且使用matplotlib库绘制运行时间进度可视化图表。
阅读全文
相关推荐
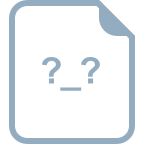




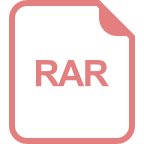
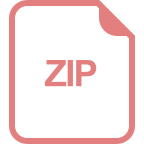
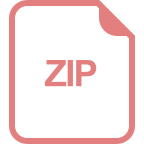
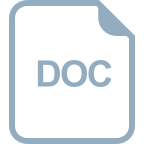



