java 如何使用epoll 来接收消息
时间: 2023-12-13 18:05:42 浏览: 82
Java中使用epoll来接收消息,通常需要以下步骤:
1. 创建一个Selector对象,通过Selector.open()方法实现。
2. 创建ServerSocketChannel或SocketChannel对象,并将其注册到Selector对象上。在注册过程中,需要指定感兴趣的事件类型,如OP_READ、OP_WRITE等。
3. 调用Selector的select()方法,阻塞等待事件发生。当有事件发生时,select()方法会返回事件数量。
4. 遍历Selector.selectedKeys()集合,处理事件。对于每个事件,根据事件类型进行相应的处理,如读取数据、写入数据等。
5. 处理完事件后,需要将对应的SelectionKey从Selector.selectedKeys()集合中移除。
以下是使用epoll来接收消息的示例代码:
```java
Selector selector = Selector.open();
ServerSocketChannel serverChannel = ServerSocketChannel.open();
serverChannel.configureBlocking(false);
serverChannel.socket().bind(new InetSocketAddress(port));
serverChannel.register(selector, SelectionKey.OP_ACCEPT);
while (true) {
selector.select();
Iterator<SelectionKey> it = selector.selectedKeys().iterator();
while (it.hasNext()) {
SelectionKey key = it.next();
it.remove();
if (key.isAcceptable()) {
SocketChannel clientChannel = serverChannel.accept();
clientChannel.configureBlocking(false);
clientChannel.register(selector, SelectionKey.OP_READ);
} else if (key.isReadable()) {
SocketChannel clientChannel = (SocketChannel) key.channel();
ByteBuffer buffer = ByteBuffer.allocate(1024);
int count = clientChannel.read(buffer);
if (count > 0) {
buffer.flip();
byte[] bytes = new byte[buffer.remaining()];
buffer.get(bytes);
String message = new String(bytes, "UTF-8");
System.out.println("Received message: " + message);
} else {
clientChannel.close();
}
}
}
}
```
在这个示例中,我们创建了一个Selector对象,并将ServerSocketChannel注册到Selector上。使用while循环不断调用Selector.select()方法,等待事件发生。当有事件发生时,我们遍历Selector.selectedKeys()集合,处理对应的事件。在处理读事件时,我们使用SocketChannel.read()方法读取数据,并将数据转换为字符串输出。
阅读全文
相关推荐
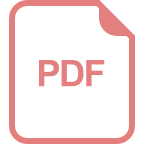
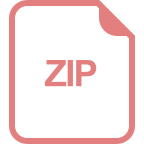



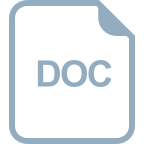
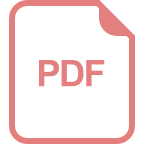
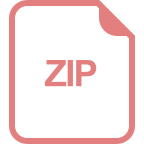
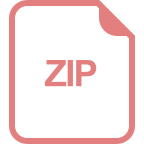
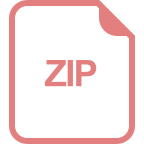
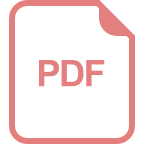
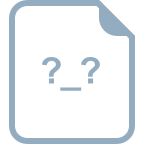
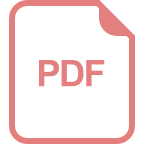
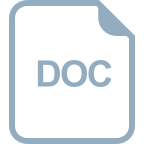
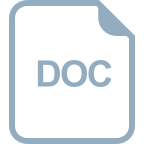
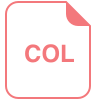
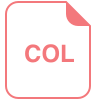
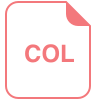