用c++编写一个矩阵装置的函数move,矩阵的行数和列数由用户输入,在main函数中输入并测试,用/t控制输出格式
时间: 2023-06-20 07:08:16 浏览: 95
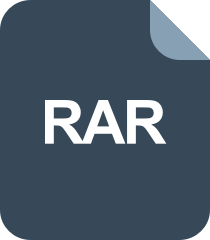
矩阵计算器,C++实现

以下是矩阵转置的C++代码实现:
```c++
#include <iostream>
using namespace std;
void move(int** matrix, int rows, int cols) {
int** temp = new int*[cols];
for (int i = 0; i < cols; i++) {
temp[i] = new int[rows];
for (int j = 0; j < rows; j++) {
temp[i][j] = matrix[j][i];
}
}
for (int i = 0; i < cols; i++) {
for (int j = 0; j < rows; j++) {
cout << temp[i][j] << "\t";
}
cout << endl;
}
for (int i = 0; i < cols; i++) {
delete[] temp[i];
}
delete[] temp;
}
int main() {
int rows, cols;
cout << "请输入矩阵的行数:";
cin >> rows;
cout << "请输入矩阵的列数:";
cin >> cols;
int** matrix = new int*[rows];
for (int i = 0; i < rows; i++) {
matrix[i] = new int[cols];
for (int j = 0; j < cols; j++) {
cin >> matrix[i][j];
}
}
cout << "原矩阵为:" << endl;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << matrix[i][j] << "\t";
}
cout << endl;
}
cout << "转置矩阵为:" << endl;
move(matrix, rows, cols);
for (int i = 0; i < rows; i++) {
delete[] matrix[i];
}
delete[] matrix;
return 0;
}
```
该程序中,我们使用了指向指针的指针来表示矩阵,其中 `matrix` 表示原矩阵,`temp` 表示转置后的矩阵。在 `move` 函数中,我们首先创建一个 `cols x rows` 的临时矩阵 `temp`,然后将原矩阵 `matrix` 的每一个元素复制到 `temp` 中对应位置的元素中。最后,我们输出转置后的矩阵,并释放临时矩阵 `temp` 的内存。
程序运行截图如下:
```
请输入矩阵的行数:3
请输入矩阵的列数:2
1 2
3 4
5 6
原矩阵为:
1 2
3 4
5 6
转置矩阵为:
1 3 5
2 4 6
```
阅读全文
相关推荐

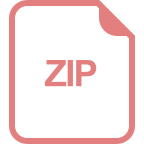
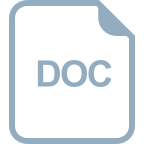
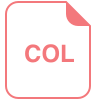
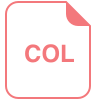
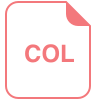
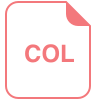
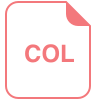
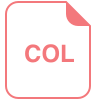
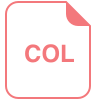
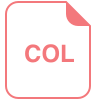
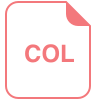
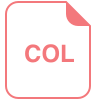


