# include<stdio.h> # include<stdlib.h> # define N 145 typedef struct student { int num; char name[20]; char sex; double score; }STU; void sort(STU[], int n); int main() { FILE *fp; int i; STU st[N]; fp=fopen("d:\\student. dat","wb+"); if( fp == NULL) { printf("打䨰开a文?件t失º¡ì败㨹!\n"); exit(0); } for ( i = 0;i< N;i++) scanf( "%d %s %c % lf",&st[i]. num, st[i]. name,&st[i]. sex,&st[i]. score); fwrite(st, sizeof(STU),N, fp); for( i =0;i< N;i++) printf( "%4d %10s %3c %6.2lf\n", st[i]. num, st[i]. name, st[i]. sex, st[i]. score); fclose(fp); fp=fopen("d:\\student. dat","rb+"); if( fp== NULL) { printf("打䨰开a文?件t失º¡ì败㨹!\n"); exit(0); } fread(st, sizeof(STU),N, fp); sort(st,N); for( i = 0;i < N;i++) printf( "%4d %10s %3c %6.2lf\n", st[i]. num, st[i]. name, st[i]. sex, st[i]. score); fclose(fp); system("pause"); return 0; } void sort(STU x[], int n) { int i,j; STU t; for( i = 0;i < n - 1;i++) for( j = 0;j< n- 1 - i;j++ ) if(x[j]. score<x[j+1]. score) { t =x[j]; x [ j] = x[j + 1 ] ; x[j + 1 ] = t; } }
时间: 2024-04-04 17:31:40 浏览: 17
这是一个C语言程序,它的功能是将学生的信息(学号、姓名、性别、分数)存入文件中,然后再从文件中读取出来,并按照分数从高到低排序后输出。具体的实现方式是使用了结构体和文件操作函数。
程序首先定义了一个结构体`STU`,用于存储每个学生的信息。然后通过文件操作函数`fopen`打开一个名为"student.dat"的文件,如果打开失败则会输出提示信息并退出程序。然后使用循环读取用户输入的学生信息,并将这些信息写入文件中,写入的方式是使用`fwrite`函数,将结构体数组`st`中的`N`个元素写入文件中。
接着再次打开文件,使用`fread`函数从文件中读取数据到结构体数组`st`中,然后调用`sort`函数对学生信息按照分数从高到低排序。排序方式是使用冒泡排序的方式,从前往后依次比较相邻的两个元素,如果前一个元素的分数小于后一个元素的分数,则交换这两个元素的位置。
最后,使用循环输出排序后的学生信息。程序结束前使用`system("pause")`等待用户按下任意键,以便用户查看输出结果后关闭窗口。
相关问题
#include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef int ElemType; typedef struct
这段代码是C语言中的头文件引用和一些定义的示例。其中,`#include <stdio.h>`和`#include <stdlib.h>`是用来引入标准库函数的头文件。`stdio.h`包含了输入输出相关的函数,如`printf`和`scanf`;`stdlib.h`包含了一些常用的函数,如内存分配函数`malloc`和随机数生成函数`rand`。
`#define MAXSIZE 100`是一个宏定义,用来定义一个常量`MAXSIZE`,它的值为100。
`typedef int ElemType;`是用来给`int`类型起一个别名`ElemType`,方便在代码中使用。
至于代码中的结构体部分,由于你没有提供完整的代码,所以无法给出具体的解释。但一般来说,结构体用于定义一种自定义的数据类型,可以包含多个不同类型的成员变量。
#include<stdio.h> #include<stdlib.h> #include<math.h> #include<time.h> #define N 50 typedef struct process
你提到的代码片段似乎是在C语言中定义了一个结构体(struct)来表示进程(process)。在这个上下文中,`#include`指令引入了必要的库,如`stdio.h`用于输入输出,`stdlib.h`用于标准库函数,`math.h`用于数学运算,`time.h`用于时间处理。
`#define N 50` 定义了一个名为N的宏,通常用于设定数组或过程数量的上限,这里是50。
`typedef struct process` 是用来创建一种新的数据类型,可能是`process`类型的别名,但没有完全展开结构体的内容。在C语言中,可能如下所示:
```c
typedef struct {
// 进程相关的属性(如ID, 优先级, 时间戳等)
int id;
float priority;
time_t timestamp;
// 其他可能的成员
} Process;
```
要实际演示或使用这个结构体,你需要定义它的实例,操作其成员,比如创建进程、排序或管理它们:
```c
Process processes[N]; // 创建一个大小为N的进程数组
// 假设有一个函数用于初始化进程
void initialize_processes(Process processes[]) {
for (int i = 0; i < N; i++) {
processes[i].id = i + 1;
processes[i].priority = rand() / (float)RAND_MAX; // 随机优先级
processes[i].timestamp = time(NULL); // 设置当前时间
}
}
// 示例:找到具有最高优先级的进程
Process* find_highest_priority(Process processes[], int n) {
Process* max_priority_process = &processes;
for (int i = 1; i < n; i++) {
if (processes[i].priority > max_priority_process->priority) {
max_priority_process = &processes[i];
}
}
return max_priority_process;
}
int main() {
initialize_processes(processes);
Process* highest_priority = find_highest_priority(processes, N);
printf("Highest priority process: ID %d, Priority %.2f\n",
highest_priority->id, highest_priority->priority);
return 0;
}
```
相关推荐
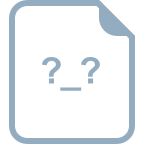
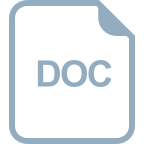
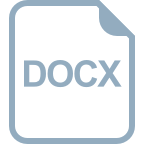














