在opencv-python中怎么对数据集的直方图进行保存
时间: 2024-03-23 18:39:42 浏览: 30
在 OpenCV-Python 中,可以使用 `cv2.calcHist` 函数计算数据集的直方图,然后使用 `cv2.normalize` 函数将直方图归一化,最后使用 `cv2.imwrite` 函数将直方图保存为图像文件。具体步骤如下:
1. 读取数据集,并将其转换为灰度图像。
```
import cv2
import numpy as np
dataset = np.load("dataset.npy")
dataset_gray = cv2.cvtColor(dataset, cv2.COLOR_BGR2GRAY)
```
2. 计算直方图。这里以灰度图像为例,计算灰度值范围为 [0, 255] 的直方图。
```
hist = cv2.calcHist([dataset_gray], [0], None, [256], [0, 256])
```
3. 归一化直方图。
```
cv2.normalize(hist, hist, 0, 255, cv2.NORM_MINMAX)
```
4. 创建直方图图像。
```
hist_image = np.zeros((256, 256), dtype=np.uint8)
```
5. 绘制直方图。
```
bin_width = hist_image.shape[1] / hist.shape[0]
for i in range(hist.shape[0]):
x = int(i * bin_width)
y = int(hist_image.shape[0] - np.round(hist[i]))
cv2.line(hist_image, (x, hist_image.shape[0]), (x, y), 255)
```
6. 保存直方图图像。
```
cv2.imwrite("histogram.png", hist_image)
```
这里将直方图保存为 PNG 格式,文件名为 "histogram.png"。
完整代码示例:
```
import cv2
import numpy as np
# 读取数据集
dataset = np.load("dataset.npy")
# 转换为灰度图像
dataset_gray = cv2.cvtColor(dataset, cv2.COLOR_BGR2GRAY)
# 计算直方图
hist = cv2.calcHist([dataset_gray], [0], None, [256], [0, 256])
# 归一化直方图
cv2.normalize(hist, hist, 0, 255, cv2.NORM_MINMAX)
# 创建直方图图像
hist_image = np.zeros((256, 256), dtype=np.uint8)
# 绘制直方图
bin_width = hist_image.shape[1] / hist.shape[0]
for i in range(hist.shape[0]):
x = int(i * bin_width)
y = int(hist_image.shape[0] - np.round(hist[i]))
cv2.line(hist_image, (x, hist_image.shape[0]), (x, y), 255)
# 保存直方图图像
cv2.imwrite("histogram.png", hist_image)
```
这段代码将读取名为 "dataset.npy" 的数据集,将其转换为灰度图像,并计算并绘制其直方图,并将直方图保存为名为 "histogram.png" 的文件。
相关推荐
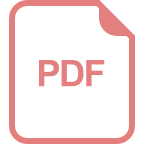
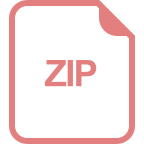
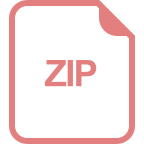
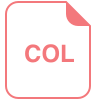
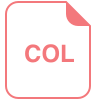
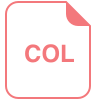











