tortoise fatal: Could not read from remote repository. Please make sure you have the correct access rights and the repository exists.
时间: 2023-11-04 16:56:31 浏览: 162
tortoise fatal: Could not read from remote repository. Please make sure you have the correct access rights and the repository exists. 这个错误通常发生在使用TortoiseGit时,当你尝试从远程存储库读取时,发生了访问权限错误或存储库不存在。解决此问题的方法包括确保你拥有正确的访问权限,并确保存储库存在。
相关问题
下面是一道java编程题In the well-known story of "The tortoise and the hare", the hare was too confident and took a break in the middle of the competition, which led to the tortoise winning the competition. A multi-threaded program is required to simulate the race between the tortoise and the hare. The speed of the tortoise is 1 meter per 1500 milliseconds, and the speed of the hare is 5 meters per 500 milliseconds. When the hare reached the 700th meter, it chose to rest for 10,000 milliseconds. As a result, the tortoise wins the race. The output result of the program running is shown in the figure.
Sure, here is a possible implementation of the program in Java:
```java
public class TortoiseAndHareRace {
private static final int RACE_LENGTH = 1000;
private static final int TORTOISE_SPEED = 1; // meter per 1500 milliseconds
private static final int HARE_SPEED = 5; // meters per 500 milliseconds
private static final int HARE_REST_DISTANCE = 700;
private static final long HARE_REST_TIME = 10000; // milliseconds
public static void main(String[] args) throws InterruptedException {
int tortoiseDistance = 0;
int hareDistance = 0;
boolean hareIsResting = false;
System.out.println("ON YOUR MARK, GET SET");
System.out.println("BANG!!!!");
System.out.println("AND THEY'RE OFF!!!!");
while (tortoiseDistance < RACE_LENGTH && hareDistance < RACE_LENGTH) {
// Tortoise moves
Thread.sleep(1500);
tortoiseDistance += TORTOISE_SPEED;
System.out.println("Tortoise runs 1 meter");
// Hare moves
if (!hareIsResting) {
Thread.sleep(500);
hareDistance += HARE_SPEED;
System.out.println("Hare runs 5 meters");
// Check if the hare needs to rest
if (hareDistance >= HARE_REST_DISTANCE) {
hareIsResting = true;
System.out.println("Hare is resting");
Thread.sleep(HARE_REST_TIME);
hareIsResting = false;
System.out.println("Hare wakes up and continues running");
}
}
// Print the current distance
System.out.println("Tortoise: " + tortoiseDistance + " meters");
System.out.println("Hare: " + hareDistance + " meters");
}
// Print the race result
if (tortoiseDistance >= RACE_LENGTH && hareDistance >= RACE_LENGTH) {
System.out.println("It's a tie!");
} else if (tortoiseDistance >= RACE_LENGTH) {
System.out.println("TORTOISE WINS!!!");
} else {
System.out.println("Hare wins. Yuch.");
}
}
}
```
This program uses two integer variables `tortoiseDistance` and `hareDistance` to keep track of the distances the tortoise and the hare have traveled, respectively. It also uses a boolean variable `hareIsResting` to indicate whether the hare is resting or not.
The program starts by printing some messages to simulate the start of the race. It then enters a loop that simulates the race until one of the runners reaches the finish line (`RACE_LENGTH` meters). Inside the loop, the tortoise moves 1 meter every 1500 milliseconds, and the hare moves 5 meters every 500 milliseconds, except when it is resting. If the hare reaches the `HARE_REST_DISTANCE` (700 meters), it rests for `HARE_REST_TIME` (10000 milliseconds) before continuing to run.
After each iteration of the loop, the program prints the current distances of the tortoise and the hare. When the loop ends, the program prints a message indicating the winner of the race.
Note that the output result may vary slightly between runs due to the randomness in the timing of the threads.
ModuleNotFoundError: No module named 'tortoise.connection'
这个错误通常是因为你的Python环境缺少了`tortoise-orm`模块。你可以尝试在终端中使用以下命令来安装该模块:
```python
pip install tortoise-orm
```
如果你使用的是Anaconda环境,则可以使用以下命令:
```python
conda install -c conda-forge tortoise-orm
```
安装完成后,你就可以在Python代码中使用`tortoise.connection`模块了。
阅读全文
相关推荐
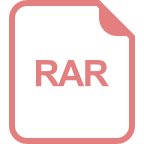
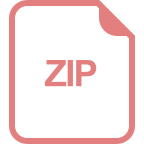
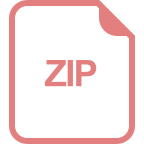
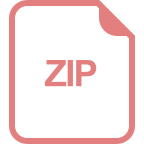
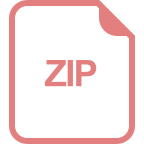
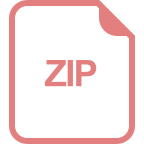
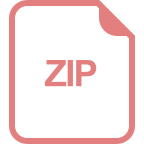
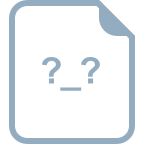
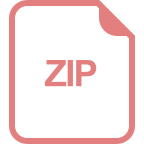
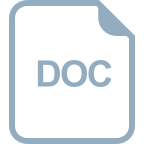
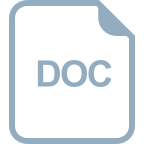
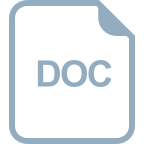
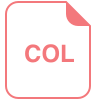

