python创建一个三行两列的列表
时间: 2023-08-07 21:01:57 浏览: 65
可以使用Python语言创建一个20以内的奇数序列表,代码如下:
```python
odd_numbers = [num for num in range(1, 21) if num % 2 == 1]
print(odd_numbers)
```
这里使用了列表推导式,指定了数字范围为1到20,并通过筛选条件筛选出了奇数,最终生成了一个奇数序列列表。输出结果为:
```
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
```
相关问题
rich绘制一个三行两列的表格
您可以使用rich库的Table对象来创建一个三行两列的表格,并使用add_row()方法添加行和add_column()方法添加列。
以下是一个示例代码:
```python
from rich.console import Console
from rich.table import Table
console = Console()
table = Table(show_header=True, header_style="bold magenta")
table.add_column("Column 1")
table.add_column("Column 2")
table.add_row("Row 1, Column 1", "Row 1, Column 2")
table.add_row("Row 2, Column 1", "Row 2, Column 2")
table.add_row("Row 3, Column 1", "Row 3, Column 2")
console.print(table)
```
在上面的代码中,我们创建了一个包含两列的表格,并使用add_row()方法添加了三行数据。您可以根据需要更改列的数量和行的内容。
输出如下:
```
┏━━━━━━━━━━━┳━━━━━━━━━━━┓
┃ Column 1 ┃ Column 2 ┃
┡━━━━━━━━━━━╇━━━━━━━━━━━┩
│ Row 1, │ Row 1, │
│ Column 1 │ Column 2 │
├───────────┼───────────┤
│ Row 2, │ Row 2, │
│ Column 1 │ Column 2 │
├───────────┼───────────┤
│ Row 3, │ Row 3, │
│ Column 1 │ Column 2 │
└───────────┴───────────┘
```
可以看到,这是一个三行两列的表格。
使用python画图,三行两列,其中每个图是折线图
### 回答1:
可以使用Matplotlib库来画图,以下是示例代码:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y1 = [2, 4, 6, 8, 10]
y2 = [1, 3, 5, 7, 9]
y3 = [10, 8, 6, 4, 2]
y4 = [9, 7, 5, 3, 1]
y5 = [5, 5, 5, 5, 5]
# 绘图
fig, axs = plt.subplots(nrows=3, ncols=2, figsize=(8, 8))
axs[, ].plot(x, y1)
axs[, 1].plot(x, y2)
axs[1, ].plot(x, y3)
axs[1, 1].plot(x, y4)
axs[2, ].plot(x, y5)
# 设置标题和标签
axs[, ].set_title('Line 1')
axs[, 1].set_title('Line 2')
axs[1, ].set_title('Line 3')
axs[1, 1].set_title('Line 4')
axs[2, ].set_title('Line 5')
fig.suptitle('Multiple Lines')
# 显示图像
plt.show()
```
这段代码会生成一个三行两列的图像,每个图像是一个折线图。你可以根据自己的需求修改数据和图像的样式。
### 回答2:
使用Python语言绘制图形可以借助于matplotlib库来完成,以下以绘制三行两列的折线图为例:
首先,我们需要导入matplotlib库中的pyplot模块,并给定数据进行绘制。
```python
import matplotlib.pyplot as plt
# 数据准备
x = [1, 2, 3, 4, 5] # x轴数据
y1 = [1, 3, 4, 2, 5] # y轴数据1
y2 = [2, 4, 1, 3, 6] # y轴数据2
y3 = [3, 2, 5, 4, 1] # y轴数据3
# 创建绘图窗口,设置布局为3行2列
plt.subplot(321)
plt.plot(x, y1) # 绘制第一个子图
plt.subplot(322)
plt.plot(x, y2) # 绘制第二个子图
plt.subplot(323)
plt.plot(x, y3) # 绘制第三个子图
# 可以依此类推,绘制其他子图
# 显示图形
plt.show()
```
上述代码中,我们通过`plt.subplot(321)`来创建一个3行2列的图形窗口,并指定绘制第一个子图。然后通过`plt.plot(x, y1)`函数绘制该子图的折线图。依此类推,通过更改`plt.subplot`和`plt.plot`的参数,即可绘制其他子图的折线图。
最后,通过`plt.show()`函数来显示绘制好的图形。
以上就是使用Python绘制三行两列折线图的简单示例。通过类似的方法,我们可以绘制更复杂的图形,实现更丰富的数据可视化。
### 回答3:
使用Python绘制三行两列的折线图可以通过matplotlib库来实现。下面是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
# 数据准备
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 7, 3, 5]
y2 = [2, 5, 2, 6, 1]
y3 = [3, 2, 4, 5, 7]
y4 = [4, 1, 3, 2, 6]
y5 = [5, 6, 1, 4, 3]
y6 = [6, 3, 5, 1, 2]
# 绘制图表
fig, axs = plt.subplots(3, 2, figsize=(10, 10))
# 第一行第一列
axs[0, 0].plot(x, y1)
axs[0, 0].set_title('Plot 1')
# 第一行第二列
axs[0, 1].plot(x, y2)
axs[0, 1].set_title('Plot 2')
# 第二行第一列
axs[1, 0].plot(x, y3)
axs[1, 0].set_title('Plot 3')
# 第二行第二列
axs[1, 1].plot(x, y4)
axs[1, 1].set_title('Plot 4')
# 第三行第一列
axs[2, 0].plot(x, y5)
axs[2, 0].set_title('Plot 5')
# 第三行第二列
axs[2, 1].plot(x, y6)
axs[2, 1].set_title('Plot 6')
# 调整子图间距
plt.tight_layout()
# 显示图表
plt.show()
```
这段代码使用matplotlib库的`subplots`函数创建一个包含3行2列子图的画布,并使用`plot`函数分别在不同的子图中绘制了6个折线图。
相关推荐
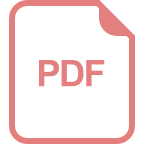
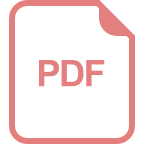













