实现一个按优先级进程调度算法以及时间片轮转调度算法的c++程序
时间: 2024-02-28 07:55:52 浏览: 102
好的,下面是一个同时支持按优先级和时间片轮转的进程调度算法的 C++ 程序。程序中使用了两个优先级队列(一个按照优先级排列,一个按照时间片轮转顺序排列),并且使用了一个计时器来模拟时间片轮转。程序会不断从两个队列中取出优先级最高的进程,然后执行一个时间片。如果进程还未运行完毕,那么会将其重新插入到队列中,否则将其从队列中删除。
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Process {
int pid; // 进程 ID
int priority; // 优先级
int burst_time; // 还需要运行的时间
int runtime; // 已经运行的时间
// 构造函数
Process(int pid, int priority, int burst_time)
: pid(pid)
, priority(priority)
, burst_time(burst_time)
, runtime(0)
{
}
// 运行一个时间片,返回是否已经完成
bool run(int quantum)
{
runtime += quantum;
burst_time -= quantum;
return burst_time <= 0;
}
// 重载小于运算符,用于优先级队列排序
bool operator<(const Process& other) const
{
if (priority == other.priority) {
return pid > other.pid;
}
return priority < other.priority;
}
};
int main()
{
// 创建两个优先级队列,一个按照优先级排序,一个按照时间片轮转顺序排序
priority_queue<Process> pq_priority;
priority_queue<Process, vector<Process>, function<bool(const Process&, const Process&)>> pq_rr(
[](const Process& a, const Process& b) {
return a.runtime > b.runtime;
});
// 添加一些进程到队列中
pq_priority.emplace(1, 2, 20);
pq_priority.emplace(2, 1, 30);
pq_priority.emplace(3, 3, 15);
pq_priority.emplace(4, 2, 25);
// 定义一个计时器,用于模拟时间片轮转
int timer = 0;
// 循环运行进程,直到队列为空
while (!pq_priority.empty() || !pq_rr.empty()) {
// 从两个队列中取出优先级最高的进程
Process current;
if (pq_priority.empty()) {
current = pq_rr.top();
pq_rr.pop();
} else if (pq_rr.empty()) {
current = pq_priority.top();
pq_priority.pop();
} else if (pq_priority.top() < pq_rr.top()) {
current = pq_priority.top();
pq_priority.pop();
} else {
current = pq_rr.top();
pq_rr.pop();
}
// 运行一个时间片
bool completed = current.run(10);
timer += 10;
if (!completed) {
// 进程还未完成,根据已运行时间重新插入到队列中
pq_rr.push(current);
}
// 打印当前进程信息
cout << "Time: " << timer << "ms, ";
cout << "PID: " << current.pid << ", ";
cout << "Priority: " << current.priority << ", ";
cout << "Burst Time: " << current.burst_time << endl;
}
return 0;
}
```
希望这个程序可以帮到您!
阅读全文
相关推荐
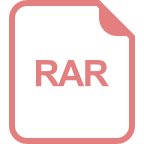
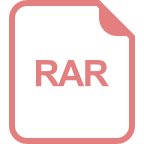
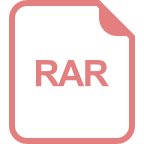
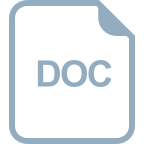
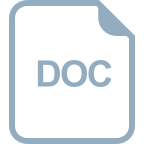
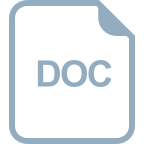
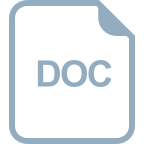
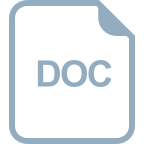
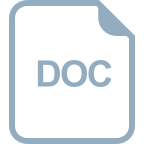
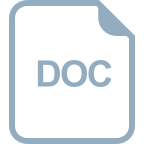






