定义一个rational类
时间: 2023-09-15 15:05:46 浏览: 93
### 回答1:
下面是一个简单的 rational 类的定义,它表示有理数:
```python
class Rational:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
self.reduce()
def reduce(self):
gcd_num = gcd(self.numerator, self.denominator)
self.numerator //= gcd_num
self.denominator //= gcd_num
def __add__(self, other):
new_num = self.numerator * other.denominator + self.denominator * other.numerator
new_den = self.denominator * other.denominator
return Rational(new_num, new_den)
def __sub__(self, other):
new_num = self.numerator * other.denominator - self.denominator * other.numerator
new_den = self.denominator * other.denominator
return Rational(new_num, new_den)
def __mul__(self, other):
new_num = self.numerator * other.numerator
new_den = self.denominator * other.denominator
return Rational(new_num, new_den)
def __truediv__(self, other):
new_num = self.numerator * other.denominator
new_den = self.denominator * other.numerator
return Rational(new_num, new_den)
def __str__(self):
return str(self.numerator) + '/' + str(self.denominator)
def __repr__(self):
return 'Rational({}, {})'.format(self.numerator, self.denominator)
```
这个类实现了有理数的加、减、乘、除等基本运算,并且重载了相应的运算符。注意,这个类的构造函数需要传入分子和分母,而且需要在构造函数中进行约分操作。
### 回答2:
Rational类是用来表示有理数的一个类。有理数是能够由一个整数除以另一个整数得到的数。在Rational类中,我们可以定义和操作这些有理数。
首先,这个类应该有两个私有成员变量:一个分子和一个分母,分别用来表示有理数的分子和分母。这两个成员变量应该是整数类型。
接下来,需要提供一个构造函数,用来初始化Rational类的对象。这个构造函数接受两个整数作为参数,分别用来初始化分子和分母。
然后,可以提供一些公有方法来操作有理数。比如,可以提供一个加法方法,可以接受另一个Rational对象作为参数,将两个有理数相加并返回一个新的Rational对象。类似地,还可以提供减法、乘法和除法等方法。
此外,为了方便输出有理数的结果,可以重写toString方法,将有理数的分子和分母以分数的形式输出。比如,一个Rational对象的输出可能是"2/3"。
最后,为了方便使用,还可以提供一些辅助方法。比如,可以提供一个简化方法,将有理数表示为最简分数。可以使用最大公约数来实现简化操作。
总之,Rational类就是用来表示有理数的一个类,它可以提供一些方法和功能,用于操作和处理有理数。
### 回答3:
Rational类是用于表示有理数的类,在数学中有理数是指可以表示为两个整数的比值的数。以下是一个定义Rational类的示例:
```python
class Rational:
def __init__(self, numerator, denominator):
self.numerator = numerator
self.denominator = denominator
self.simplify()
def simplify(self):
'''简化有理数'''
gcd = self.find_gcd(self.numerator, self.denominator)
self.numerator //= gcd
self.denominator //= gcd
@staticmethod
def find_gcd(a, b):
'''求最大公约数'''
while b:
a, b = b, a % b
return a
def __add__(self, other):
'''重载加法运算符'''
new_numerator = self.numerator * other.denominator + other.numerator * self.denominator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
def __sub__(self, other):
'''重载减法运算符'''
new_numerator = self.numerator * other.denominator - other.numerator * self.denominator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
def __mul__(self, other):
'''重载乘法运算符'''
new_numerator = self.numerator * other.numerator
new_denominator = self.denominator * other.denominator
return Rational(new_numerator, new_denominator)
def __truediv__(self, other):
'''重载除法运算符'''
new_numerator = self.numerator * other.denominator
new_denominator = self.denominator * other.numerator
return Rational(new_numerator, new_denominator)
def __str__(self):
'''重载字符串表示方法'''
return f"{self.numerator}/{self.denominator}"
```
上述代码定义了一个Rational类,使用初始化方法`__init__`接受分子和分母参数,并通过简化方法`simplify`将有理数化简为最简形式。静态方法`find_gcd`用于求最大公约数。重载了加减乘除运算符,分别返回相应操作后得到的有理数对象。`__str__`方法将有理数对象以字符串形式表示。通过这个Rational类,我们可以进行有理数的加减乘除等运算。
相关推荐
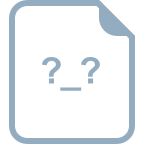
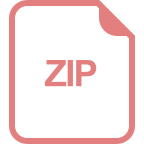














