Android如何通过代码获取cpu使用率
时间: 2023-09-18 12:06:46 浏览: 49
你可以通过以下代码获取Android设备的CPU使用率:
1. 首先,你需要获取设备当前的CPU使用情况:
```java
private static final String TAG = "CpuUsage";
private static final int UPDATE_INTERVAL = 1000; // 更新间隔时间
private static long lastTotalCpuTime = 0;
private static long lastProcessCpuTime = 0;
public static float getCpuUsage() {
float cpuUsage = 0.0f;
long totalCpuTime = getTotalCpuTime();
long processCpuTime = getProcessCpuTime();
if (lastTotalCpuTime != 0) {
long deltaTotalCpuTime = totalCpuTime - lastTotalCpuTime;
long deltaProcessCpuTime = processCpuTime - lastProcessCpuTime;
cpuUsage = deltaProcessCpuTime * 100.0f / deltaTotalCpuTime;
}
lastTotalCpuTime = totalCpuTime;
lastProcessCpuTime = processCpuTime;
return cpuUsage;
}
private static long getTotalCpuTime() {
try {
BufferedReader reader = new BufferedReader(new FileReader("/proc/stat"));
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith("cpu ")) {
String[] fields = line.split("\\s+");
long totalCpuTime = 0;
for (int i = 1; i < fields.length; i++) {
totalCpuTime += Long.parseLong(fields[i]);
}
return totalCpuTime;
}
}
} catch (IOException e) {
Log.e(TAG, "Failed to read /proc/stat", e);
}
return 0;
}
private static long getProcessCpuTime() {
try {
int pid = android.os.Process.myPid();
BufferedReader reader = new BufferedReader(new FileReader("/proc/" + pid + "/stat"));
String line = reader.readLine();
String[] fields = line.split("\\s+");
long utime = Long.parseLong(fields[13]);
long stime = Long.parseLong(fields[14]);
long cutime = Long.parseLong(fields[15]);
long cstime = Long.parseLong(fields[16]);
return utime + stime + cutime + cstime;
} catch (IOException e) {
Log.e(TAG, "Failed to read /proc/pid/stat", e);
}
return 0;
}
```
2. 然后,你需要使用一个定时器来定时获取CPU使用情况:
```java
private Timer mTimer = new Timer();
private TimerTask mTimerTask = new TimerTask() {
@Override
public void run() {
float cpuUsage = getCpuUsage();
Log.d(TAG, "CPU Usage: " + cpuUsage);
}
};
// 在onCreate()中启动定时器
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mTimer.schedule(mTimerTask, 0, UPDATE_INTERVAL);
}
// 在onDestroy()中停止定时器
@Override
protected void onDestroy() {
super.onDestroy();
mTimer.cancel();
}
```
这样,你就可以通过代码获取Android设备的CPU使用率了。
相关推荐
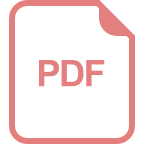
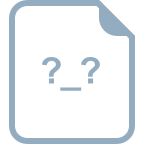














