编写程序声明一个车(vehicle)基类,有run, stop等成员函数,由此派生出自行车(bicycle)类,汽车(motorcar)类,由bicycle和motorcar派生出摩托车(motorcycle)类,它们都有Run、Stop等成员函数。观察虚函数的作用
时间: 2023-06-08 12:05:12 浏览: 234
请看下面的 C++ 代码:
```c++
class Vehicle {
public:
virtual void Run() {
cout << "Vehicle is running." << endl;
}
virtual void Stop() {
cout << "Vehicle is stopping." << endl;
}
};
class Bicycle : public Vehicle {
public:
void Run() {
cout << "Bicycle is running." << endl;
}
void Stop() {
cout << "Bicycle is stopping." << endl;
}
};
class Motorcar : public Vehicle {
public:
void Run() {
cout << "Motorcar is running." << endl;
}
void Stop() {
cout << "Motorcar is stopping." << endl;
}
};
class Motorcycle : public Bicycle, public Motorcar {
public:
void Run() {
cout << "Motorcycle is running." << endl;
}
void Stop() {
cout << "Motorcycle is stopping." << endl;
}
};
int main() {
Vehicle *ptrVehicle;
Bicycle bicycle;
Motorcar motorcar;
Motorcycle motorcycle;
ptrVehicle = &bicycle;
ptrVehicle->Run(); // 输出: Bicycle is running.
ptrVehicle->Stop(); // 输出: Bicycle is stopping.
ptrVehicle = &motorcar;
ptrVehicle->Run(); // 输出: Motorcar is running.
ptrVehicle->Stop(); // 输出: Motorcar is stopping.
ptrVehicle = &motorcycle;
ptrVehicle->Run(); // 输出: Motorcycle is running.
ptrVehicle->Stop(); // 输出: Motorcycle is stopping.
return 0;
}
```
虚函数的作用表现在多态上,当我们使用一个指向基类的指针或引用来调用一个虚函数时,会根据它所指向的对象的类型来选择相应的实现。例如上面代码中,ptrVehicle 指针指向 Bicycle 对象时,调用 Run 和 Stop 函数时输出 Bicycle 相关信息;指向 Motorcar 时,输出 Motorcar 相关信息;指向 Motorcycle 时,输出 Motorcycle 相关信息。虚函数的特性使得多态成为可能,让我们能够非常灵活地使用继承机制编写程序。
相关推荐
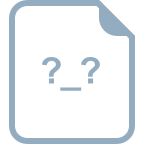
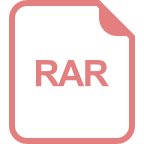














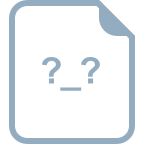
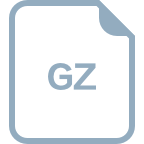