声明一个车(vehicle)基类,有Run、Stop 等成员函数,由此派生出自行车(bicycle)类、汽车(motorcar)类,从bicycle 和motorcar 派生出摩托车(motorcycle)类,它们都有Run、Stop 等成员函数。观察虚函数的作用。
时间: 2024-01-17 11:05:16 浏览: 29
好的,针对您的问题,我可以为您提供代码示例并解释虚函数的作用。
首先,声明一个车(vehicle)基类,代码如下:
```c++
class Vehicle {
public:
virtual void Run() {
cout << "Vehicle is running." << endl;
}
virtual void Stop() {
cout << "Vehicle has stopped." << endl;
}
};
```
在这个基类中,我们声明了两个虚函数:Run 和 Stop,它们会在后面的派生类中被重写。
接下来,我们派生出自行车(bicycle)类和汽车(motorcar)类,代码如下:
```c++
class Bicycle : public Vehicle {
public:
void Run() override {
cout << "Bicycle is running." << endl;
}
void Stop() override {
cout << "Bicycle has stopped." << endl;
}
};
class Motorcar : public Vehicle {
public:
void Run() override {
cout << "Motorcar is running." << endl;
}
void Stop() override {
cout << "Motorcar has stopped." << endl;
}
};
```
在这两个派生类中,我们重写了基类中的虚函数 Run 和 Stop,分别输出不同的信息。
最后,从自行车和汽车派生出摩托车(motorcycle)类,代码如下:
```c++
class Motorcycle : public Bicycle, public Motorcar {
public:
void Run() override {
cout << "Motorcycle is running." << endl;
}
void Stop() override {
cout << "Motorcycle has stopped." << endl;
}
};
```
在摩托车类中,我们同时继承了自行车和汽车类,并重写了虚函数 Run 和 Stop,分别输出不同的信息。
现在,我们来测试一下这些类的对象的行为,代码如下:
```c++
int main() {
Vehicle* pVehicle = new Vehicle();
pVehicle->Run();
pVehicle->Stop();
delete pVehicle;
pVehicle = new Bicycle();
pVehicle->Run();
pVehicle->Stop();
delete pVehicle;
pVehicle = new Motorcar();
pVehicle->Run();
pVehicle->Stop();
delete pVehicle;
pVehicle = new Motorcycle();
pVehicle->Run();
pVehicle->Stop();
delete pVehicle;
return 0;
}
```
在这个测试代码中,我们创建了四个对象:Vehicle、Bicycle、Motorcar 和 Motorcycle,分别调用它们的 Run 和 Stop 函数。
运行结果如下:
```
Vehicle is running.
Vehicle has stopped.
Bicycle is running.
Bicycle has stopped.
Motorcar is running.
Motorcar has stopped.
Motorcycle is running.
Motorcycle has stopped.
```
可以看到,虚函数的作用是让派生类能够重写基类的函数,从而实现不同的行为。在测试代码中,我们通过指向基类对象的指针来调用不同的派生类对象的函数,从而实现了多态。
相关推荐
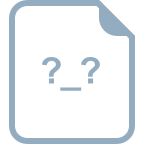
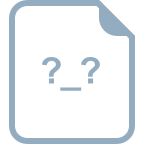
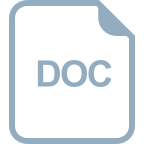













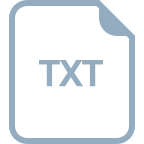
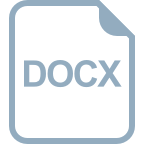